Excel Fixed Columns Width Text
The following example shows how to read and write a fixed-width text file programmatically in C# and VB.NET, using the GemBox.Spreadsheet library.
using GemBox.Spreadsheet;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY");
// Define columns width (for input file format).
var loadOptions = new FixedWidthLoadOptions(
new FixedWidthColumn(8),
new FixedWidthColumn(8),
new FixedWidthColumn(8));
// Load file.
var workbook = ExcelFile.Load("%#FixedColumnsWidthText.prn%", loadOptions);
// Modify file.
workbook.Worksheets.ActiveWorksheet.GetUsedCellRange(true).Sort(false).By(1).Apply();
// Define columns width (for output file format).
var saveOptions = new FixedWidthSaveOptions(
new FixedWidthColumn(8),
new FixedWidthColumn(8),
new FixedWidthColumn(8));
workbook.Save("Fixed Columns Width Text.prn", saveOptions);
}
}
Imports GemBox.Spreadsheet
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY")
' Define columns width (for input file format).
Dim loadOptions As New FixedWidthLoadOptions(
New FixedWidthColumn(8),
New FixedWidthColumn(8),
New FixedWidthColumn(8))
' Load file.
Dim workbook = ExcelFile.Load("%#FixedColumnsWidthText.prn%", loadOptions)
' Modify file.
workbook.Worksheets.ActiveWorksheet.GetUsedCellRange(True).Sort(False).By(1).Apply()
' Define columns width (for output file format).
Dim saveOptions As New FixedWidthSaveOptions(
New FixedWidthColumn(8),
New FixedWidthColumn(8),
New FixedWidthColumn(8))
workbook.Save("Fixed Columns Width Text.prn", saveOptions)
End Sub
End Module
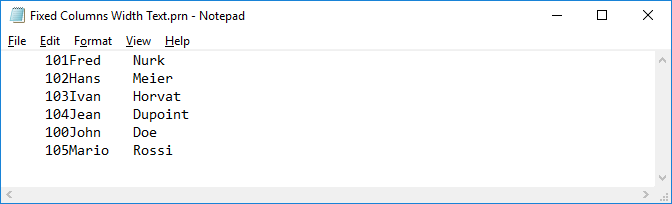
A fixed-width text file is a type of text file where data is organized into columns, and each column has a fixed number of characters allocated for it. There is no need for field delimiters or text quoting, as the columns are fixed in width. If a value is shorter than the specified column width, it is padded with space characters to fill the remaining space.