Create an Excel file in MAUI
GemBox.Spreadsheet is a standalone .NET component with cross-platform support for processing workbooks. With the help of MAUI, you can use it on platforms like Android, iOS, macOS, and Windows for reading, writing, editing, and converting Excel files.
The following example shows how use GemBox.Spreadsheet to create an XLSX file in an Android or iOS mobile application using MAUI.
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="SpreadsheetMaui.MainPage">
<ScrollView>
<VerticalStackLayout
Spacing="20"
Padding="50"
VerticalOptions="Center">
<Label
Text="GemBox.Spreadsheet Example"
HorizontalOptions="Center"
FontSize="Large"
Margin="0,0,0,30" />
<TableView x:Name="table" Intent="Data" >
<TableRoot>
<TableSection>
<EntryCell Label="A1" Text="Joe Doe" />
<EntryCell Label="A2" Text="Fred Nurk" />
<EntryCell Label="A3" Text="Hans Meier" />
<EntryCell Label="A4" Text="Ivan Horvat" />
<EntryCell Label="A5" Text="Jean Dupont" />
</TableSection>
</TableRoot>
</TableView>
<ActivityIndicator x:Name="activity" />
<Button
x:Name="button"
Text="Create workbook"
Clicked="Button_Clicked"/>
</VerticalStackLayout>
</ScrollView>
</ContentPage>
using GemBox.Spreadsheet;
namespace SpreadsheetMaui
{
public partial class MainPage : ContentPage
{
static MainPage()
{
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY");
}
public MainPage()
{
InitializeComponent();
}
private async Task<string> CreateWorkbookAsync()
{
var workbook = new ExcelFile();
var worksheet = workbook.Worksheets.Add("Sheet1");
foreach (var cell in table.Root[0].Cast<EntryCell>())
worksheet.Cells[cell.Label].Value = cell.Text;
worksheet.Columns["A"].AutoFit();
using var stream = new MemoryStream();
using (var imageStream = await FileSystem.OpenAppPackageFileAsync("dices.png"))
await imageStream.CopyToAsync(stream);
worksheet.Pictures.Add(stream, ExcelPictureFormat.Png, "C1", "E5");
var filePath = Path.Combine(Environment.GetFolderPath(Environment.SpecialFolder.MyDocuments), "Example.pdf");
await Task.Run(() => workbook.Save(filePath));
return filePath;
}
private async void Button_Clicked(object sender, EventArgs e)
{
button.IsEnabled = false;
activity.IsRunning = true;
try
{
var filePath = await CreateWorkbookAsync();
await Launcher.OpenAsync(new OpenFileRequest(Path.GetFileName(filePath), new ReadOnlyFile(filePath)));
}
catch (Exception ex)
{
await DisplayAlert("Error", ex.Message, "Close");
}
activity.IsRunning = false;
button.IsEnabled = true;
}
}
}
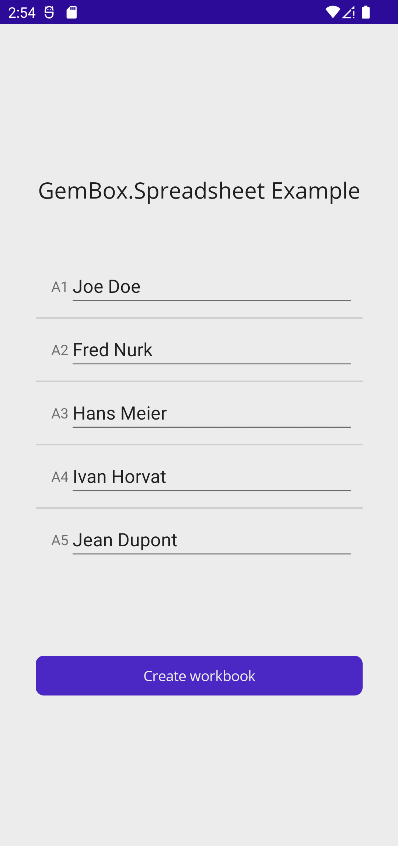
Using full functionality of GemBox.Spreadsheet in MAUI application requires adjustments explained in detail on Supported Platforms help page.