Excel Row and Column AutoFit in C# and VB.NET
The following example shows how to autofit all columns in an Excel worksheet in C# and VB.NET, using the GemBox.Spreadsheet library. Autofiting ensures that all data is displayed correctly when opened in Excel, by adjusting the cells width.
using GemBox.Spreadsheet;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY");
var workbook = ExcelFile.Load("%InputFileName%");
var worksheet = workbook.Worksheets.ActiveWorksheet;
int columnCount = worksheet.CalculateMaxUsedColumns();
for (int i = 0; i < columnCount; i++)
worksheet.Columns[i].AutoFit(1, worksheet.Rows[1], worksheet.Rows[worksheet.Rows.Count - 1]);
workbook.Save("Row_Column AutoFit.%OutputFileType%");
}
}
Imports GemBox.Spreadsheet
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY")
Dim workbook = ExcelFile.Load("%InputFileName%")
Dim worksheet = workbook.Worksheets.ActiveWorksheet
Dim columnCount = worksheet.CalculateMaxUsedColumns()
For i As Integer = 0 To columnCount - 1
worksheet.Columns(i).AutoFit(1, worksheet.Rows(1), worksheet.Rows(worksheet.Rows.Count - 1))
Next
workbook.Save("Row_Column AutoFit.%OutputFileType%")
End Sub
End Module
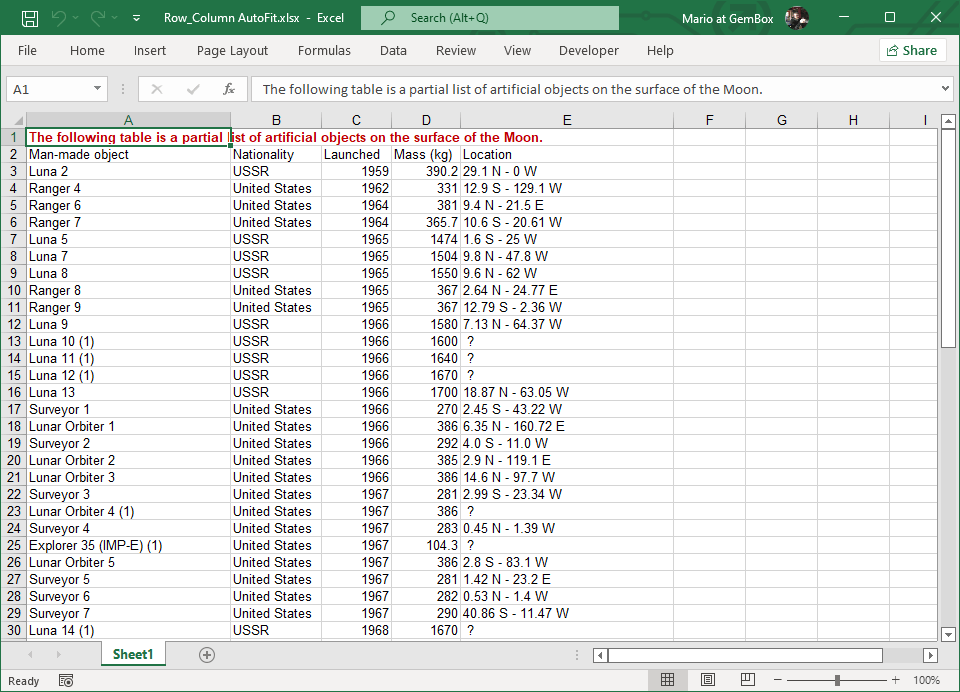