Excel Form Controls in C# and VB.NET
The following example shows how to create an Excel workbook with some of the most common form controls such as checkboxes, radio (option) buttons, combo boxes, buttons, list boxes, spin buttons, scroll bars, labels, or group boxes, using the GemBox.Spreadsheet library in C# and VB.NET.
using GemBox.Spreadsheet;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY");
var workbook = new ExcelFile();
var worksheet = workbook.Worksheets.Add("Form Controls");
var checkBox = worksheet.FormControls.AddCheckBox("Simple check box", "B2", 100, 15, LengthUnit.Point);
checkBox.CellLink = worksheet.Cells["A2"];
checkBox.Checked = true;
worksheet.Cells["A4"].Value = "VALUE A";
worksheet.Cells["A5"].Value = "VALUE B";
worksheet.Cells["A6"].Value = "VALUE C";
worksheet.Cells["A7"].Value = "VALUE D";
var comboBox = worksheet.FormControls.AddComboBox("B4", 100, 20, LengthUnit.Point);
comboBox.InputRange = worksheet.Cells.GetSubrange("A4:A7");
comboBox.SelectedIndex = 2;
var scrollBar = worksheet.FormControls.AddScrollBar("B9", 100, 20, LengthUnit.Point);
scrollBar.CellLink = worksheet.Cells["A9"];
scrollBar.MinimumValue = 10;
scrollBar.MaximumValue = 50;
scrollBar.CurrentValue = 20;
workbook.Save("Form Controls.xlsx");
}
}
Imports GemBox.Spreadsheet
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY")
Dim workbook As New ExcelFile()
Dim worksheet = workbook.Worksheets.Add("Form Controls")
Dim checkBox = worksheet.FormControls.AddCheckBox("Simple check box", "B2", 100, 15, LengthUnit.Point)
checkBox.CellLink = worksheet.Cells("A2")
checkBox.Checked = True
worksheet.Cells("A4").Value = "VALUE A"
worksheet.Cells("A5").Value = "VALUE B"
worksheet.Cells("A6").Value = "VALUE C"
worksheet.Cells("A7").Value = "VALUE D"
Dim comboBox = worksheet.FormControls.AddComboBox("B4", 100, 20, LengthUnit.Point)
comboBox.InputRange = worksheet.Cells.GetSubrange("A4:A7")
comboBox.SelectedIndex = 2
Dim scrollBar = worksheet.FormControls.AddScrollBar("B9", 100, 20, LengthUnit.Point)
scrollBar.CellLink = worksheet.Cells("A9")
scrollBar.MinimumValue = 10
scrollBar.MaximumValue = 50
scrollBar.CurrentValue = 20
workbook.Save("Form Controls.xlsx")
End Sub
End Module
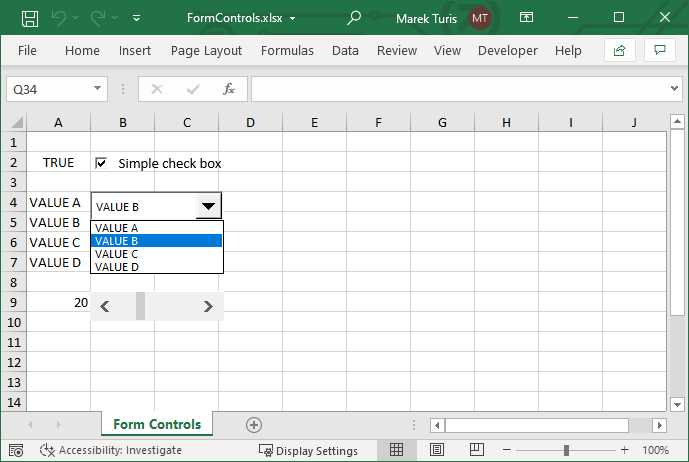
The form controls are stored in the ExcelWorksheet.FormControls
collection and are represented with one of the derived types of ExcelFormControl
.
The following example shows how to read and update form controls.
using GemBox.Spreadsheet;
using GemBox.Spreadsheet.Drawing;
using System;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY");
var workbook = ExcelFile.Load("%#FormControls.xlsx%");
var worksheet = workbook.Worksheets[0];
// Update CheckBox control.
var checkBox = worksheet.FormControls[0] as CheckBox;
checkBox.Checked = false;
// Read CheckBox control.
Console.WriteLine("CheckBox checked: " + checkBox.Checked);
Console.WriteLine("Linked cell value: " + checkBox.CellLink?.Value);
Console.WriteLine();
// Update ComboBox control.
var comboBox = worksheet.FormControls[1] as ComboBox;
comboBox.SelectedIndex = 1;
// Read ComboBox control.
Console.WriteLine("ComboBox range: " + comboBox.InputRange?.Name);
Console.WriteLine("ComboBox selected: " + comboBox.SelectedValue);
Console.WriteLine();
// Update ScrollBar control.
var scrollBar = worksheet.FormControls[2] as ScrollBar;
scrollBar.CurrentValue = 33;
// Read ScrollBar control.
Console.WriteLine("ScrollBar current: " + scrollBar.CurrentValue);
Console.WriteLine("Linked cell value: " + scrollBar.CellLink?.Value);
}
}
Imports GemBox.Spreadsheet
Imports GemBox.Spreadsheet.Drawing
Imports System
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY")
Dim workbook = ExcelFile.Load("%#FormControls.xlsx%")
Dim worksheet = workbook.Worksheets(0)
' Update CheckBox control.
Dim checkBox = TryCast(worksheet.FormControls(0), CheckBox)
checkBox.Checked = False
' Read CheckBox control.
Console.WriteLine("CheckBox checked: " & checkBox.Checked)
Console.WriteLine("Linked cell value: " & checkBox.CellLink?.Value)
Console.WriteLine()
' Update ComboBox control.
Dim comboBox = TryCast(worksheet.FormControls(1), ComboBox)
comboBox.SelectedIndex = 1
' Read ComboBox control.
Console.WriteLine("ComboBox range: " & comboBox.InputRange?.Name)
Console.WriteLine("ComboBox selected: " & comboBox.SelectedValue)
Console.WriteLine()
' Update ScrollBar control.
Dim scrollBar = TryCast(worksheet.FormControls(2), ScrollBar)
scrollBar.CurrentValue = 33
' Read ScrollBar control.
Console.WriteLine("ScrollBar current: " & scrollBar.CurrentValue)
Console.WriteLine("Linked cell value: " & scrollBar.CellLink?.Value)
End Sub
End Module
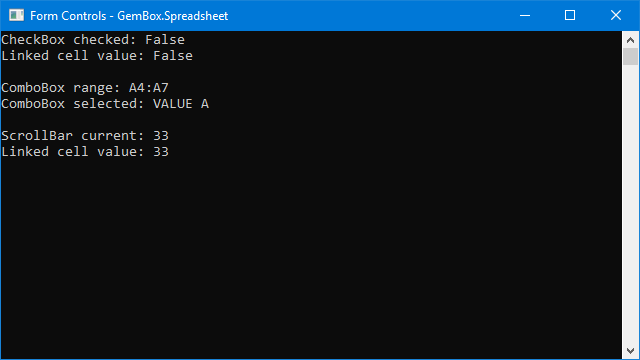