Excel Encryption for XLSX
Below you can find an example that demonstrates how to load an encrypted XLSX file and then save it as an encrypted XLSX file using a different password in both C# and VB.NET, using the GemBox.Spreadsheet library.
using GemBox.Spreadsheet;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY");
var inputPassword = "%InputPassword%";
var outputPassword = "%OutputPassword%";
var workbook = ExcelFile.Load("%InputFileName%",
new XlsxLoadOptions() { Password = inputPassword });
workbook.Save("XLSX Encryption.xlsx",
new XlsxSaveOptions() { Password = outputPassword });
}
}
Imports GemBox.Spreadsheet
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY")
Dim inputPassword = "%InputPassword%"
Dim outputPassword = "%OutputPassword%"
Dim workbook = ExcelFile.Load("%InputFileName%",
New XlsxLoadOptions With {.Password = inputPassword})
workbook.Save("XLSX Encryption.xlsx",
New XlsxSaveOptions With {.Password = outputPassword})
End Sub
End Module
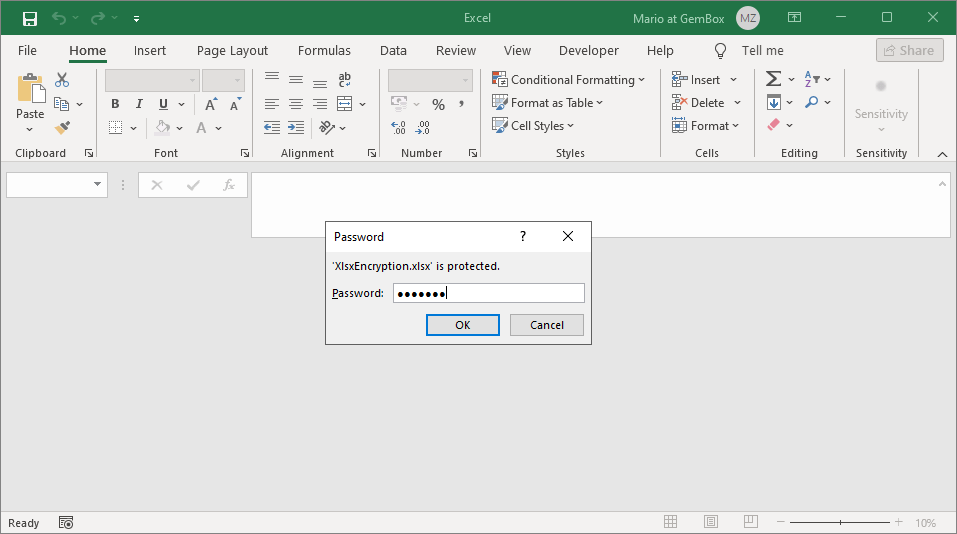
To access an encrypted Excel workbook, you'll need to provide a valid password using the XlsxLoadOptions.Password
property. In case of an invalid password, GemBox.Spreadsheet won't be able to decrypt the file, and will throw a CryptographicException
when trying to load it.
To write an encrypted Excel workbook, all you need to do is provide a password using the XlsxSaveOptions.Password
property. In Microsoft Excel, that would be the following option:
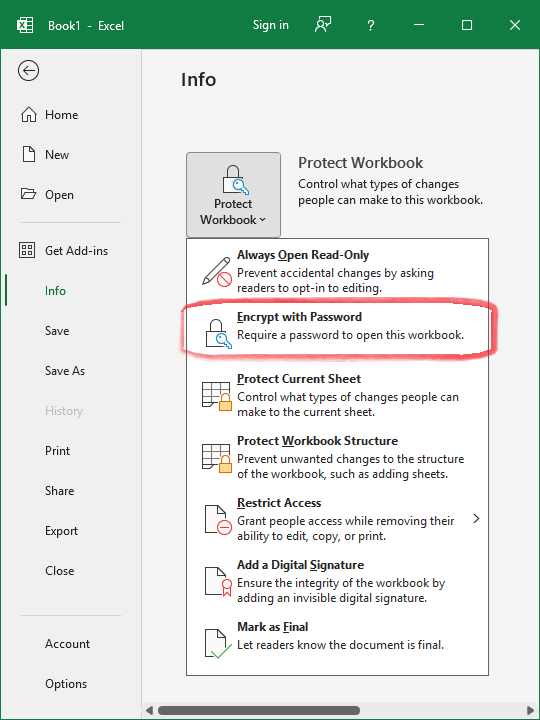
Note that passwords are case-sensitive and can't be empty.
You can use the XlsxLoadOptions.IsEncrypted
static methods to check if an Excel workbook is encrypted.