Convert Excel files to HTML
GemBox.Spreadsheet provides C# and VB.NET API for easy conversion between Excel workbooks and HTML pages. The following sections provide example codes for the most common conversion options:
- Convert an Excel file to multiple HTML pages
- Convert Excel sheets to a single HTML page
- Convert an HTML page to an Excel file
Convert an Excel file to multiple HTML pages
The following example shows how to save an entire Excel workbook, with its sheets and images to HTML, using GemBox.Spreadsheet.
using GemBox.Spreadsheet;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY");
var workbook = ExcelFile.Load("%#HtmlExport.xlsx%");
var worksheet = workbook.Worksheets[0];
// Set some ExcelPrintOptions properties for HTML export.
worksheet.PrintOptions.PrintHeadings = true;
worksheet.PrintOptions.PrintGridlines = true;
// Specify cell range which should be exported to HTML.
worksheet.NamedRanges.SetPrintArea(worksheet.Cells.GetSubrange("A1", "J42"));
var options = new HtmlSaveOptions()
{
HtmlType = HtmlType.Html,
SelectionType = SelectionType.EntireFile
};
workbook.Save("HtmlExport.html", options);
}
}
Imports GemBox.Spreadsheet
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY")
Dim workbook = ExcelFile.Load("%#HtmlExport.xlsx%")
Dim worksheet = workbook.Worksheets(0)
' Set some ExcelPrintOptions properties for HTML export.
worksheet.PrintOptions.PrintHeadings = True
worksheet.PrintOptions.PrintGridlines = True
' Specify cell range which should be exported to HTML.
worksheet.NamedRanges.SetPrintArea(worksheet.Cells.GetSubrange("A1", "J42"))
Dim options = New HtmlSaveOptions() With
{
.HtmlType = HtmlType.Html,
.SelectionType = SelectionType.EntireFile
}
workbook.Save("HtmlExport.html", options)
End Sub
End Module
The code above will produce an HTML file and related files directory (for HtmlExport.xlsx the output will contain HtmlExport.html file and HtmlExport_files directory). The HTML file represents the workbook where you can view worksheets by clicking on the desired sheet tab (sheet selector) at the bottom of the page.
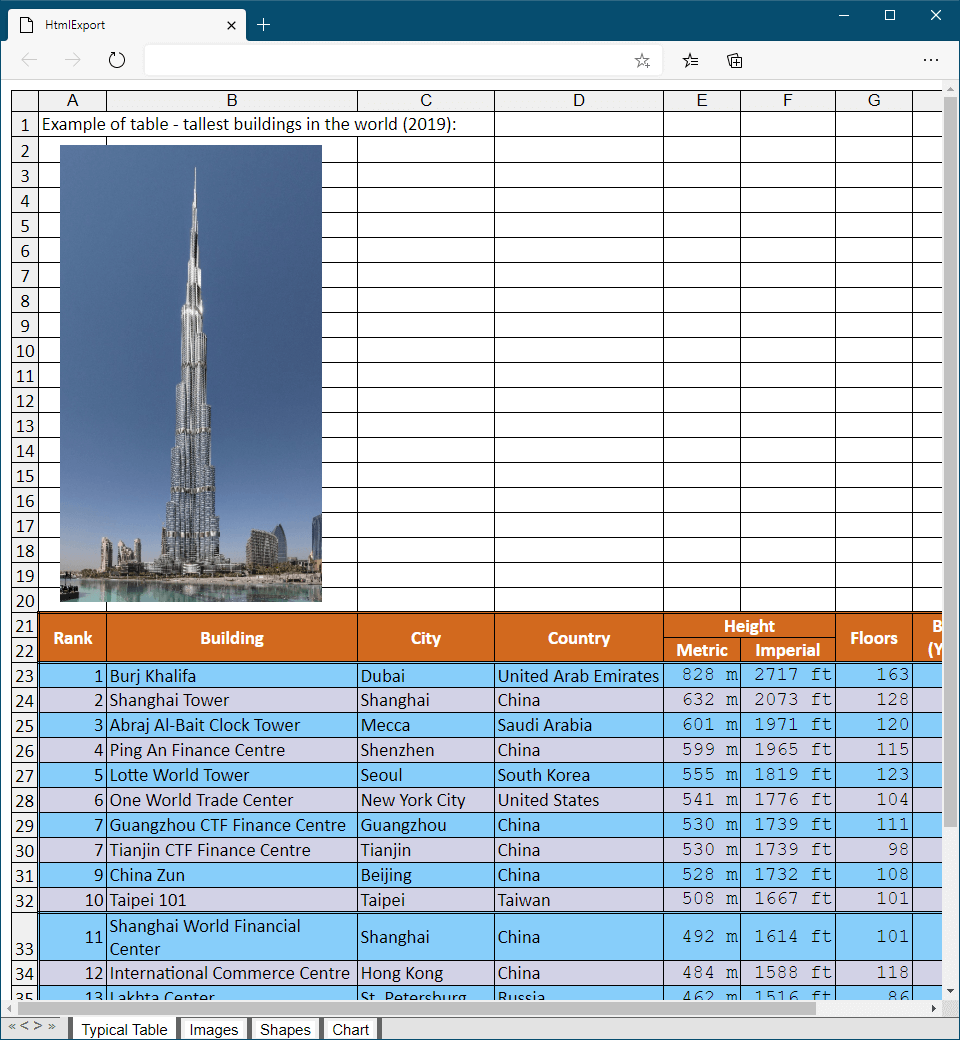
GemBox.Spreadsheet also supports properties specified on the workbook level, such as print headings, print gridlines, and print area. You can use the HtmlSaveOptions
class to set additional conversion options such as:
- Choose between HTML and MHTML (MIME HTML) output format.
- Whether to embed images directly within the HTML file or not.
- Whether to export the whole workbook or only the selected worksheet.
- Whether to export a part of the file as an independent HTML table that can be inserted into your HTML page.
Convert Excel sheets to a single HTML page
The example below shows how to use GemBox.Spreadsheet to export all sheets from an Excel file as HTML table elements and combine them into a single HTML page.
using GemBox.Spreadsheet;
using System.Linq;
using System.Xml;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY");
var workbook = ExcelFile.Load("%InputFileName%");
// Specify exporting of Excel data as an HTML table with embedded images.
var options = new HtmlSaveOptions()
{
EmbedImages = true,
HtmlType = HtmlType.HtmlTable
};
using (var writer = XmlWriter.Create("SingleHtmlExport.html",
new XmlWriterSettings() { OmitXmlDeclaration = true }))
{
writer.WriteStartElement("html");
writer.WriteStartElement("body");
// Write Excel sheets to a single HTML file in reverse order.
foreach (var worksheet in workbook.Worksheets.Reverse())
{
if (worksheet.Visibility != SheetVisibility.Visible)
continue;
writer.WriteElementString("h1", worksheet.Name);
workbook.Worksheets.ActiveWorksheet = worksheet;
workbook.Save(writer, options);
}
writer.WriteEndDocument();
}
}
}
Imports GemBox.Spreadsheet
Imports System.Linq
Imports System.Xml
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY")
Dim workbook = ExcelFile.Load("%InputFileName%")
' Specify exporting of Excel data as an HTML table with embedded images.
Dim options As New HtmlSaveOptions() With
{
.EmbedImages = True,
.HtmlType = HtmlType.HtmlTable
}
Using writer = XmlWriter.Create("SingleHtmlExport.html",
New XmlWriterSettings() With {.OmitXmlDeclaration = True})
writer.WriteStartElement("html")
writer.WriteStartElement("body")
' Write Excel sheets to a single HTML file in reverse order.
For Each worksheet In workbook.Worksheets.Reverse()
If worksheet.Visibility <> SheetVisibility.Visible Then Continue For
writer.WriteElementString("h1", worksheet.Name)
workbook.Worksheets.ActiveWorksheet = worksheet
workbook.Save(writer, options)
Next
writer.WriteEndDocument()
End Using
End Sub
End Module
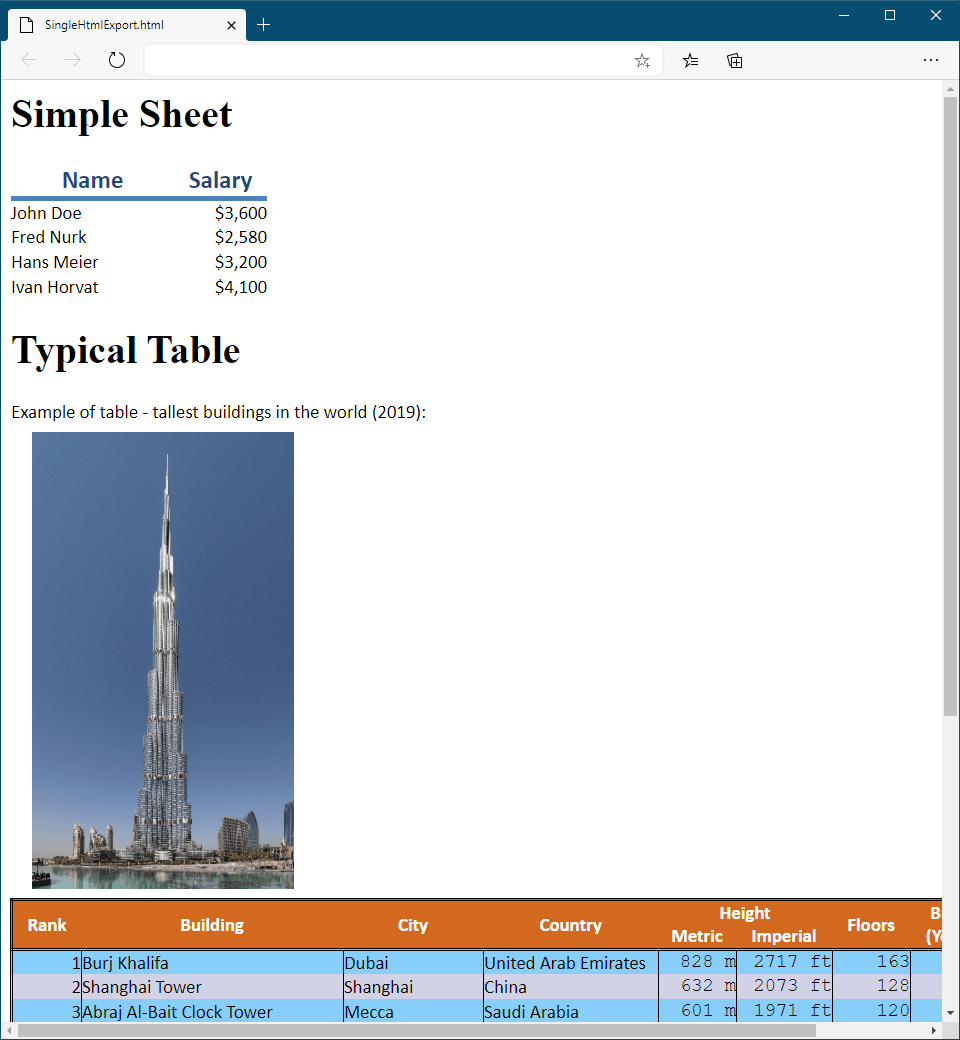
As an alternative way of converting an entire XLSX to a single HTML, you can use HtmlType.Html
. The output MHTML file will include all sheets, images, shapes, textboxes, and charts from the input Excel workbook.
If you want to export the whole Excel file together with images, shapes, textboxes, and charts, into a single HTML page, you can use The following example shows how to convert an HTML file to an Excel workbook. Apart from converting to an ExcelFile, it's possible to import HTML into a single cell by using the HtmlType.Mhtml
which will export the entire spreadsheet content as a MIME HTML (MHTML) file.Convert an HTML page to an Excel file
using GemBox.Spreadsheet;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY");
// Load input HTML file.
var workbook = ExcelFile.Load("%InputFileName%");
// Save output XLSX file.
workbook.Save("HtmlImport.xlsx");
}
}
Imports GemBox.Spreadsheet
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY")
' Load input HTML file.
Dim workbook = ExcelFile.Load("%InputFileName%")
' Save output XLSX file.
workbook.Save("HtmlImport.xlsx")
End Sub
End Module
ExcelCell.SetValue(String, HtmlLoadOptions)
method.