Convert Excel files to image formats
With the following example you will learn how to use GemBox.Spreadsheet to convert an Excel page to a PNG image of a specified size.
using GemBox.Spreadsheet;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY");
// Load an Excel file into the ExcelFile object.
var workbook = ExcelFile.Load("%InputFileName%");
// Create image save options.
var imageOptions = new ImageSaveOptions(ImageSaveFormat.Png)
{
PageNumber = 0, // Select the first Excel page.
Width = 1240, // Set the image width.
CropToContent = true // Export only the sheet's content.
};
// Save the ExcelFile object to a PNG file.
workbook.Save("Output.png", imageOptions);
}
}
Imports GemBox.Spreadsheet
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY")
' Load an Excel file into the ExcelFile object.
Dim workbook = ExcelFile.Load("%InputFileName%")
' Create image save options.
Dim imageOptions As New ImageSaveOptions(ImageSaveFormat.Png) With
{
.PageNumber = 0, ' Select the first Excel page.
.Width = 1240, ' Set the image width.
.CropToContent = True ' Export only the sheet's content.
}
' Save the ExcelFile object to a PNG file.
workbook.Save("Output.png", imageOptions)
End Sub
End Module
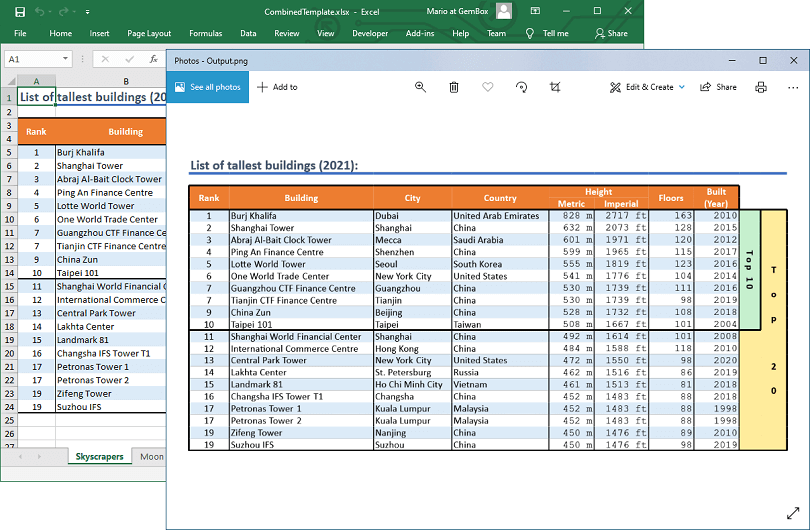
The code above is useful for generating the workbook's preview or thumbnail. Alongside from PNG, GemBox.Spreadsheet supports other You can also save multiple pages from an Excel workbook as a single multi-frame image. For this, you need to use an image format that supports multiple frames, like TIFF or GIF, and you need to specify the The following example shows how you can convert the whole Excel workbook (all its sheets) to a multi-frame image, by selecting an image format that supports multiple frames, like TIFF or GIF, and specifying the To save each page from an Excel workbook as a separate PNG or JPEG image, we recommend saving each ImageSaveOptions
like JPEG, SVG, BMP, WMP, GIF, and TIFF.Convert an Excel file to a multi-frame image
ImageSaveOptions.PageCount
property.ImageSaveOptions.PageCount
property.using GemBox.Spreadsheet;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY");
// Load an Excel file.
var workbook = ExcelFile.Load("%InputFileName%");
// Max integer value indicates that all spreadsheet pages should be saved.
var imageOptions = new ImageSaveOptions(ImageSaveFormat.Tiff)
{
SelectionType = SelectionType.EntireFile,
PageCount = int.MaxValue
};
// Save the TIFF file with multiple frames, each frame represents a single Excel page.
workbook.Save("Output.tiff", imageOptions);
}
}
Imports GemBox.Spreadsheet
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY")
' Load an Excel file.
Dim workbook = ExcelFile.Load("%InputFileName%")
' Max integer value indicates that all spreadsheet pages should be saved.
Dim imageOptions As New ImageSaveOptions(ImageSaveFormat.Tiff) With
{
.SelectionType = SelectionType.EntireFile,
.PageCount = Integer.MaxValue
}
' Save the TIFF file with multiple frames, each frame represents a single Excel page.
workbook.Save("Output.tiff", imageOptions)
End Sub
End Module
Convert an Excel file to multiple images
ExcelFilePage
as a single image file instead of saving the ExcelFile
into multiple image files, as shown in the next example.using GemBox.Spreadsheet;
using System.IO;
using System.IO.Compression;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY");
// Load an Excel file.
var workbook = ExcelFile.Load("%InputFileName%");
// Get Excel pages.
var paginatorOptions = new PaginatorOptions() { SelectionType = SelectionType.EntireFile };
var pages = workbook.GetPaginator(paginatorOptions).Pages;
// Create a ZIP file for storing PNG files.
using (var archiveStream = File.OpenWrite("Output.zip"))
using (var archive = new ZipArchive(archiveStream, ZipArchiveMode.Create))
{
var imageOptions = new ImageSaveOptions();
// Iterate through the Excel pages.
for (int pageIndex = 0; pageIndex < pages.Count; pageIndex++)
{
ExcelFilePage page = pages[pageIndex];
// Create a ZIP entry for each spreadsheet page.
var entry = archive.CreateEntry($"Page {pageIndex + 1}.png");
// Save each spreadsheet page as a PNG image to the ZIP entry.
using (var imageStream = new MemoryStream())
using (var entryStream = entry.Open())
{
page.Save(imageStream, imageOptions);
imageStream.CopyTo(entryStream);
}
}
}
}
}
Imports GemBox.Spreadsheet
Imports System.IO
Imports System.IO.Compression
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY")
' Load an Excel file.
Dim workbook = ExcelFile.Load("%InputFileName%")
' Get Excel pages.
Dim paginatorOptions As New PaginatorOptions() With {.SelectionType = SelectionType.EntireFile}
Dim pages = workbook.GetPaginator(paginatorOptions).Pages
' Create a ZIP file for storing PNG files.
Using archiveStream = File.OpenWrite("Output.zip")
Using archive As New ZipArchive(archiveStream, ZipArchiveMode.Create)
Dim imageOptions As New ImageSaveOptions()
' Iterate through the Excel pages.
For pageIndex As Integer = 0 To pages.Count - 1
Dim page As ExcelFilePage = pages(pageIndex)
' Create a ZIP entry for each spreadsheet page.
Dim entry = archive.CreateEntry($"Page {pageIndex + 1}.png")
' Save each spreadsheet page as a PNG image to the ZIP entry.
Using imageStream As New MemoryStream()
Using entryStream = entry.Open()
page.Save(imageStream, imageOptions)
imageStream.CopyTo(entryStream)
End Using
End Using
Next
End Using
End Using
End Sub
End Module