Use Placeholders in PowerPoint with C# and VB.NET
Placeholder
elements are special drawings that establish a connection between a slide and its parent layout slide (Slide.ParentTemplate
), which consequently enables users to specify common properties like layout or formatting in a single place (the layout slide).
These settings are automatically propagated to all slides whose template parent is that layout slide. You can synchronize a slide with its parent layout slide by calling the Slide.Reset
method.
With the following example, you will learn how to create and customize placeholders in C# and VB.NET using the GemBox.Presentation API.
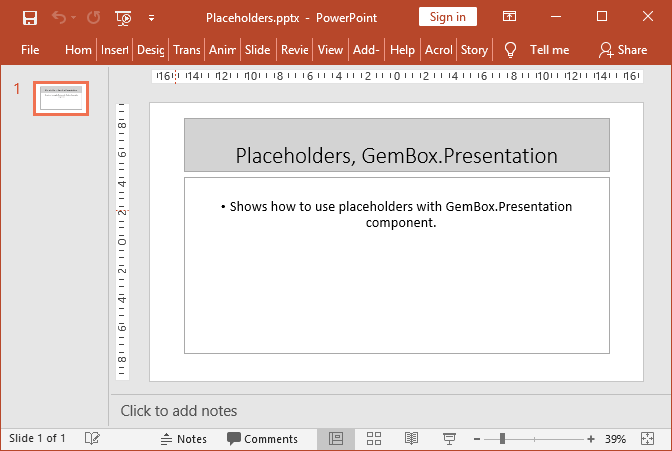
using GemBox.Presentation;
using System.Linq;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var presentation = new PresentationDocument();
// Create new master slide.
var master = presentation.MasterSlides.AddNew();
// Create new empty layout slide for existing master slide.
var layout = master.LayoutSlides.AddNew(SlideLayoutType.Custom);
// Create title and subtitle placeholders on layout slide.
layout.Content.AddPlaceholder(PlaceholderType.Title);
layout.Content.AddPlaceholder(PlaceholderType.Subtitle);
// Create new slide; will inherit all placeholders (title and subtitle) from template layout slide.
var slide = presentation.Slides.AddNew(layout);
// Retrieve "Title" placeholder.
var shape = slide.Content.Drawings.OfType<Shape>().Where(item => item.Placeholder?.PlaceholderType == PlaceholderType.Title).First();
// Set shape fill and outline format.
shape.Format.Outline.Fill.SetSolid(Color.FromName(ColorName.DarkGray));
shape.Format.Fill.SetSolid(Color.FromName(ColorName.LightGray));
// Set shape text.
shape.Text.AddParagraph().AddRun("Placeholders, GemBox.Presentation");
// Retrieve "Subtitle" placeholder.
shape = slide.Content.Drawings.OfType<Shape>().Where(item => item.Placeholder?.PlaceholderType == PlaceholderType.Subtitle).First();
// Set shape outline format.
shape.Format.Outline.Fill.SetSolid(Color.FromName(ColorName.DarkGray));
// Set shape text.
shape.Text.AddParagraph().AddRun("Shows how to use placeholders with GemBox.Presentation component.");
presentation.Save("Placeholders.pptx");
}
}
Imports GemBox.Presentation
Imports System.Linq
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim presentation = New PresentationDocument
' Create New master slide.
Dim master = presentation.MasterSlides.AddNew()
' Create New empty layout slide for existing master slide.
Dim layout = master.LayoutSlides.AddNew(SlideLayoutType.Custom)
' Create title And subtitle placeholders on layout slide.
layout.Content.AddPlaceholder(PlaceholderType.Title)
layout.Content.AddPlaceholder(PlaceholderType.Subtitle)
' Create New slide; will inherit all placeholders (title And subtitle) from template layout slide.
Dim slide = presentation.Slides.AddNew(layout)
' Retrieve "Title" placeholder.
Dim shape = slide.Content.Drawings.OfType(Of Shape).Where(Function(item) item.Placeholder IsNot Nothing AndAlso item.Placeholder.PlaceholderType = PlaceholderType.Title).First()
' Set shape fill and outline format.
shape.Format.Outline.Fill.SetSolid(Color.FromName(ColorName.DarkGray))
shape.Format.Fill.SetSolid(Color.FromName(ColorName.LightGray))
' Set shape text.
shape.Text.AddParagraph().AddRun("Placeholders, GemBox.Presentation")
' Retrieve "Subtitle" placeholder.
shape = slide.Content.Drawings.OfType(Of Shape).Where(Function(item) item.Placeholder IsNot Nothing AndAlso item.Placeholder.PlaceholderType = PlaceholderType.Subtitle).First()
' Set shape outline format.
shape.Format.Outline.Fill.SetSolid(Color.FromName(ColorName.DarkGray))
' Set shape text.
shape.Text.AddParagraph().AddRun("Shows how to use placeholders with GemBox.Presentation component.")
presentation.Save("Placeholders.pptx")
End Sub
End Module