Pictures in PowerPoint
Create Pictures
The example below shows how to use GemBox.Presentation to create and customize pictures and picture fills in your C# and VB.NET applications.
using GemBox.Presentation;
using System.IO;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var presentation = new PresentationDocument();
// Create new presentation slide.
var slide = presentation.Slides.AddNew(SlideLayoutType.Custom);
// Create first picture from resource data.
Picture picture = null;
using (var stream = File.OpenRead("%#Dices.png%"))
picture = slide.Content.AddPicture(PictureContentType.Png, stream, 2, 2, 6, 5, LengthUnit.Centimeter);
// Create "rounded rectangle" shape.
var shape = slide.Content.AddShape(ShapeGeometryType.RoundedRectangle, 10, 2, 8, 5, LengthUnit.Centimeter);
// Fill shape with picture content.
var fillFormat = shape.Format.Fill.SetPicture(picture.Fill.Data.Content);
// Set the offset of the edges of the stretched picture fill.
fillFormat.StretchLeft = 0.1;
fillFormat.StretchRight = 0.4;
fillFormat.StretchTop = 0.1;
fillFormat.StretchBottom = 0.4;
// Get shape outline format.
var lineFormat = shape.Format.Outline;
// Set shape red outline.
lineFormat.Fill.SetSolid(Color.FromName(ColorName.Red));
lineFormat.Width = Length.From(0.2, LengthUnit.Centimeter);
// Create second picture from SVG resource.
using (var stream = File.OpenRead("%#Graphics1.svg%"))
picture = slide.Content.AddPicture(PictureContentType.Svg, stream, 2, 8, 6, 3, LengthUnit.Centimeter);
presentation.Save("Pictures.pptx");
}
}
Imports GemBox.Presentation
Imports System.IO
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim presentation = New PresentationDocument
' Create New presentation slide.
Dim slide = presentation.Slides.AddNew(SlideLayoutType.Custom)
' Create first picture from resource data.
Dim picture As Picture = Nothing
Using stream As Stream = File.OpenRead("%#Dices.png%")
picture = slide.Content.AddPicture(PictureContentType.Png, stream, 2, 2, 6, 5, LengthUnit.Centimeter)
End Using
' Create "rounded rectangle" shape.
Dim shape = slide.Content.AddShape(ShapeGeometryType.RoundedRectangle, 10, 2, 8, 5, LengthUnit.Centimeter)
' Fill shape with picture content.
Dim fillFormat = shape.Format.Fill.SetPicture(picture.Fill.Data.Content)
' Set the offset of the edges of the stretched picture fill.
fillFormat.StretchLeft = 0.1
fillFormat.StretchRight = 0.4
fillFormat.StretchTop = 0.1
fillFormat.StretchBottom = 0.4
' Get shape outline format.
Dim lineFormat = shape.Format.Outline
' Set shape red outline.
lineFormat.Fill.SetSolid(Color.FromName(ColorName.Red))
lineFormat.Width = Length.From(0.2, LengthUnit.Centimeter)
' Create second picture from SVG resource.
Using stream As Stream = File.OpenRead("%#Graphics1.svg%")
picture = slide.Content.AddPicture(PictureContentType.Svg, stream, 2, 8, 6, 3, LengthUnit.Centimeter)
End Using
presentation.Save("Pictures.pptx")
End Sub
End Module
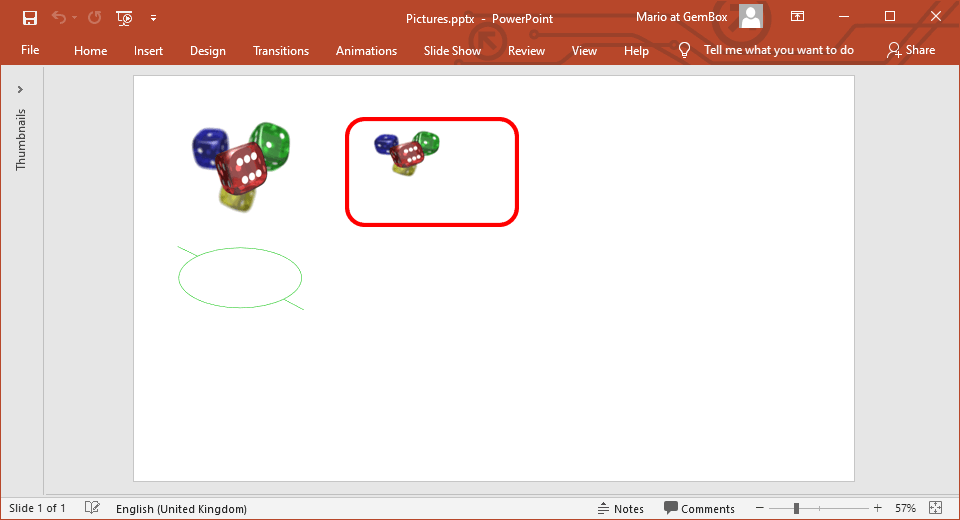
Note that images in SVG format are rendered in PDF files as vector graphics, resulting in smaller file sizes and better quality than bitmap images.
Export Pictures
The following example shows how you can export pictures from PowerPoint files.
using GemBox.Presentation;
using System.IO;
using System.Linq;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var presentation = PresentationDocument.Load("%#InputPictures.pptx%");
var slide = presentation.Slides[0];
// Get all pictures from first slide.
var pictures = slide.Content.Drawings.All().OfType<Picture>();
// Get first picture data.
Picture picture = pictures.First();
PictureContent pictureContent = picture.Fill.Data;
// Export picture data to image file.
using (var fileStream = File.Create($"Output.{pictureContent.ContentType}"))
using (var pictureStream = pictureContent.Content.Open())
pictureStream.CopyTo(fileStream);
}
}
Imports GemBox.Presentation
Imports System.IO
Imports System.Linq
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim presentation = PresentationDocument.Load("%#InputPictures.pptx%")
Dim slide = presentation.Slides(0)
' Get all pictures from first slide.
Dim pictures = slide.Content.Drawings.All().OfType(Of Picture)()
' Get first picture data.
Dim picture As Picture = pictures.First()
Dim pictureContent As PictureContent = picture.Fill.Data
' Export picture data to image file.
Using fileStream = File.Create($"Output.{pictureContent.ContentType}")
Using pictureStream = pictureContent.Content.Open()
pictureStream.CopyTo(fileStream)
End Using
End Using
End Sub
End Module
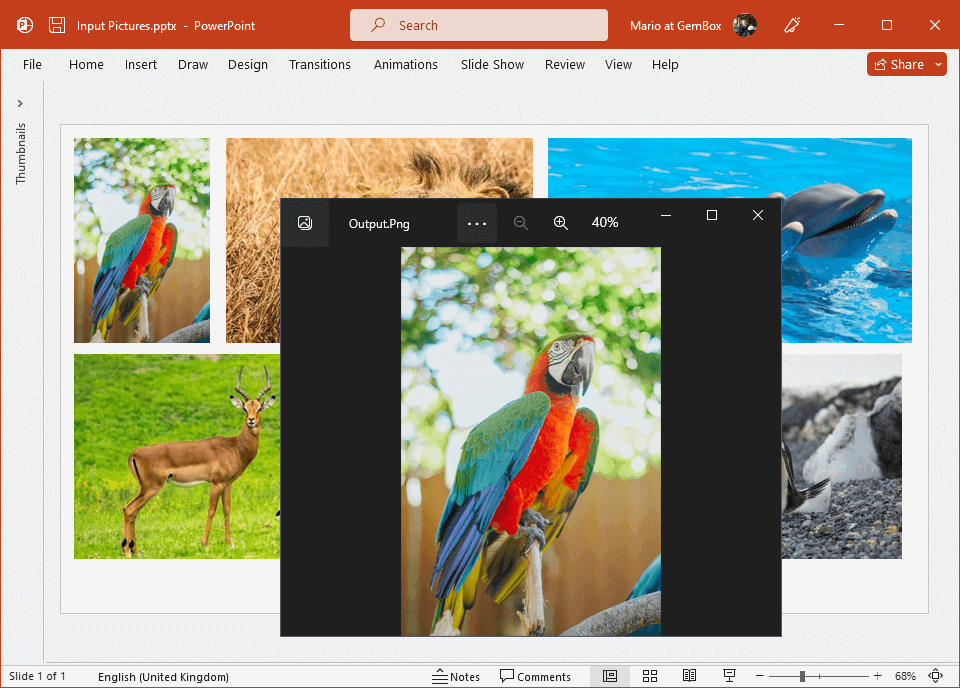
Update Pictures
The following example shows how you can update pictures in PowerPoint files by replacing them with a different picture.
using GemBox.Presentation;
using System.IO;
using System.Linq;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var presentation = PresentationDocument.Load("%InputFileName%");
var slide = presentation.Slides[0];
// Get all pictures from first slide.
var pictures = slide.Content.Drawings.All().OfType<Picture>();
// Replace pictures data with image file.
foreach (var picture in pictures)
using (var fileStream = File.OpenRead("%#Jellyfish.jpg%"))
picture.Fill.SetData(fileStream, PictureContentType.Jpeg);
presentation.Save("UpdatedPictures.pptx");
}
}
Imports GemBox.Presentation
Imports System.IO
Imports System.Linq
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim presentation = PresentationDocument.Load("%InputFileName%")
Dim slide = presentation.Slides(0)
' Get all pictures from first slide.
Dim pictures = slide.Content.Drawings.All().OfType(Of Picture)()
' Replace pictures data with image file.
For Each picture In pictures
Using fileStream = File.OpenRead("%#Jellyfish.jpg%")
picture.Fill.SetData(fileStream, PictureContentType.Jpeg)
End Using
Next
presentation.Save("UpdatedPictures.pptx")
End Sub
End Module
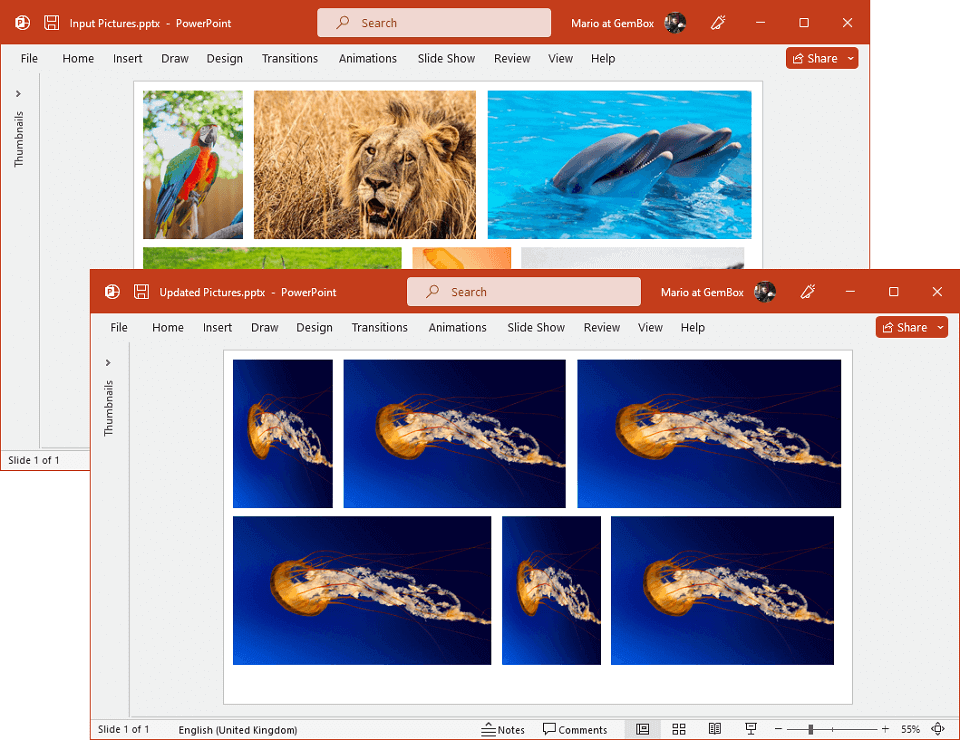