Create a PowerPoint file in MAUI
GemBox.Presentation is a standalone .NET component with cross-platform support for processing presentations. With the help of MAUI, you can use it on platforms like Android, iOS, macOS, and Windows for reading, writing, editing, and converting PowerPoint files.
The following example shows how you can create a PPTX file in a mobile application.
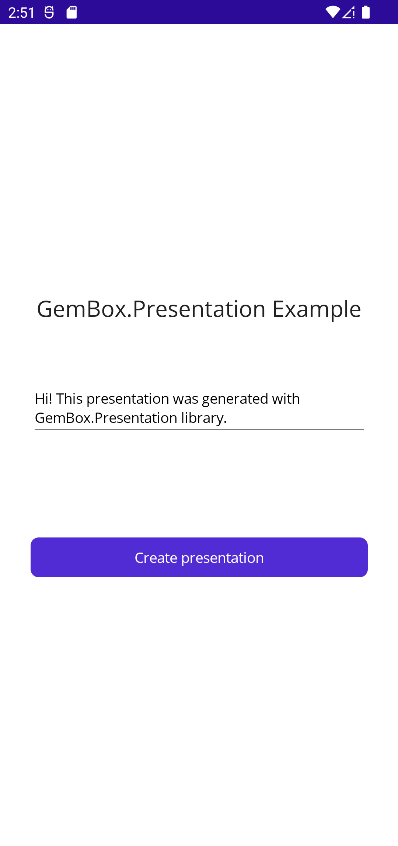
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="PresentationMaui.MainPage">
<ScrollView>
<VerticalStackLayout
Spacing="25"
Padding="30"
VerticalOptions="Center">
<Label Text="GemBox.Presentation Example"
HorizontalOptions="Center"
FontSize="Large"
Margin="0,0,0,30" />
<Editor x:Name="text"
VerticalOptions="FillAndExpand"
Text="Hi! This presentation was generated with GemBox.Presentation library." />
<ActivityIndicator x:Name="activity" />
<Button x:Name="button"
Text="Create presentation"
Clicked="Button_Clicked"/>
</VerticalStackLayout>
</ScrollView>
</ContentPage>
using GemBox.Presentation;
using System;
using System.IO;
using System.Threading.Tasks;
namespace PresentationMaui
{
public partial class MainPage : ContentPage
{
static MainPage()
{
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
}
public MainPage()
{
InitializeComponent();
}
private async Task<string> CreatePresentationAsync()
{
var presentation = new PresentationDocument();
presentation.Slides.AddNew(SlideLayoutType.Custom)
.Content.AddTextBox(ShapeGeometryType.Rectangle, 2, 2, 10, 4, LengthUnit.Centimeter)
.AddParagraph()
.AddRun(text.Text);
var filePath = Path.Combine(Environment.GetFolderPath(Environment.SpecialFolder.MyDocuments), "Example.pptx");
await Task.Run(() => presentation.Save(filePath));
return filePath;
}
private async void Button_Clicked(object sender, EventArgs e)
{
button.IsEnabled = false;
activity.IsRunning = true;
try
{
var filePath = await CreatePresentationAsync();
await Launcher.OpenAsync(new OpenFileRequest(Path.GetFileName(filePath), new ReadOnlyFile(filePath)));
}
catch (Exception ex)
{
await DisplayAlert("Error", ex.Message, "Close");
}
activity.IsRunning = false;
button.IsEnabled = true;
}
}
}
Using full functionality of GemBox.Presentation in MAUI application requires adjustments explained in detail on Supported Platforms help page.