Cloning Drawings and Slides in PowerPoint in C# and VB.NET
It’s often necessary to clone slides in PowerPoint presentations, which is basically copying the contents of a slide into a new one. It’s possible to do this process programmatically in C# and VB.NET with the GemBox.Presentation component.
Drawing (such as shapes, pictures, connectors, tables, etc.) and slide instances in GemBox.Presentation can be only at one single location in a presentation at a time. When you want to add a drawing or a slide into some other part of the same or another presentation, you must insert it as a clone.
The example below shows how you can clone drawings and slides.
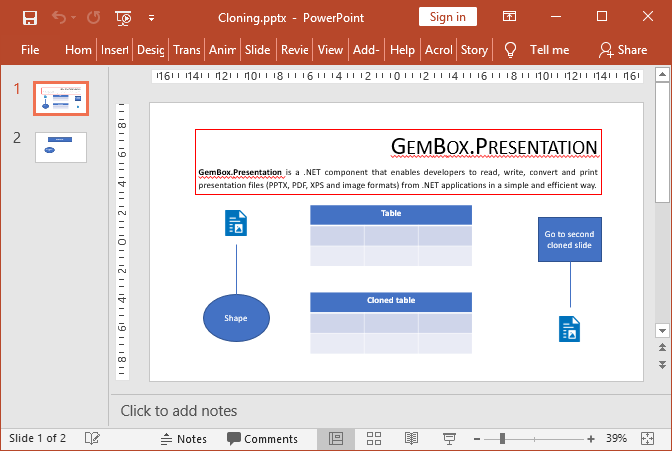
using GemBox.Presentation;
using System;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var presentation = PresentationDocument.Load("%InputFileName%");
var sourcePresentation = PresentationDocument.Load("%#CloneSource.pptx%");
// Use context so that references between
// shapes and slides are maintained between all cloning operations.
var context = CloneContext.Create(sourcePresentation, presentation);
// Clone all drawings from the first slide of another presentation
// into the first slide of the current presentation.
foreach (var drawing in sourcePresentation.Slides[0].Content.Drawings)
presentation.Slides[0].Content.Drawings.AddClone(drawing, context);
// Establish explicit mapping between slides so that
// hyperlink on the second slide is correctly cloned.
context.Set(sourcePresentation.Slides[0], presentation.Slides[0]);
// Clone the second slide from another presentation.
presentation.Slides.AddClone(sourcePresentation.Slides[1], context);
presentation.Save("Cloning.%OutputFileType%");
}
}
Imports GemBox.Presentation
Imports System
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim presentation = PresentationDocument.Load("%InputFileName%")
Dim sourcePresentation = PresentationDocument.Load("%#CloneSource.pptx%")
' Use context so that references between
' shapes and slides are maintained between all cloning operations.
Dim context = CloneContext.Create(sourcePresentation, presentation)
' Clone all drawings from the first slide of another presentation
' into the first slide of the current presentation.
For Each drawing In sourcePresentation.Slides(0).Content.Drawings
presentation.Slides(0).Content.Drawings.AddClone(drawing, context)
Next
' Establish explicit mapping between slides so that
' hyperlink on the second slide is correctly cloned.
context.Set(sourcePresentation.Slides(0), presentation.Slides(0))
' Clone the second slide from another presentation.
presentation.Slides.AddClone(sourcePresentation.Slides(1), context)
presentation.Save("Cloning.%OutputFileType%")
End Sub
End Module
Notice that cloned drawings are still connected (if they are moved, connector is also moved), same as in the source presentation, and that hyperlinks are correctly cloned because the same CloneContext
instance is used in all cloning operations.