Performance Testing with Large PowerPoint Files
The following example shows how you can test the performance of the GemBox.Presentation component with large presentation files using the Free version of the component.
using BenchmarkDotNet.Attributes;
using BenchmarkDotNet.Engines;
using BenchmarkDotNet.Jobs;
using BenchmarkDotNet.Running;
using GemBox.Presentation;
using System.Collections.Generic;
using System.IO;
[SimpleJob(RuntimeMoniker.Net80)]
[SimpleJob(RuntimeMoniker.Net48)]
public class Program
{
private PresentationDocument presentation;
private readonly Consumer consumer = new Consumer();
public static void Main()
{
BenchmarkRunner.Run<Program>();
}
[GlobalSetup]
public void SetLicense()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// If using Free version and example exceeds its limitations, use Trial or Time Limited version:
// https://www.gemboxsoftware.com/presentation/examples/free-trial-professional/901
this.presentation = PresentationDocument.Load("RandomSlides.pptx");
}
[Benchmark]
public PresentationDocument Reading()
{
return PresentationDocument.Load("RandomSlides.pptx");
}
[Benchmark]
public void Writing()
{
using (var stream = new MemoryStream())
this.presentation.Save(stream, new PptxSaveOptions());
}
[Benchmark]
public void Iterating()
{
this.LoopThroughAllDrawings().Consume(this.consumer);
}
public IEnumerable<Drawing> LoopThroughAllDrawings()
{
foreach (var slide in this.presentation.Slides)
foreach (var drawing in slide.Content.Drawings.All())
yield return drawing;
}
}
Imports BenchmarkDotNet.Attributes
Imports BenchmarkDotNet.Engines
Imports BenchmarkDotNet.Jobs
Imports BenchmarkDotNet.Running
Imports GemBox.Presentation
Imports System.Collections.Generic
Imports System.IO
<SimpleJob(RuntimeMoniker.Net80)>
<SimpleJob(RuntimeMoniker.Net48)>
Public Class Program
Private presentation As PresentationDocument
Private ReadOnly consumer As Consumer = New Consumer()
Public Shared Sub Main()
BenchmarkRunner.Run(Of Program)()
End Sub
<GlobalSetup>
Public Sub SetLicense()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' If using Free version and example exceeds its limitations, use Trial or Time Limited version:
' https://www.gemboxsoftware.com/presentation/examples/free-trial-professional/901
Me.presentation = PresentationDocument.Load("RandomSlides.pptx")
End Sub
<Benchmark>
Public Function Reading() As PresentationDocument
Return PresentationDocument.Load("RandomSlides.pptx")
End Function
<Benchmark>
Public Sub Writing()
Using stream = New MemoryStream()
Me.presentation.Save(stream, New PptxSaveOptions())
End Using
End Sub
<Benchmark>
Public Sub Iterating()
Me.LoopThroughAllDrawings().Consume(Me.consumer)
End Sub
Public Iterator Function LoopThroughAllDrawings() As IEnumerable(Of Object)
For Each slide In Me.presentation.Slides
For Each drawing In slide.Content.Drawings.All()
Yield drawing
Next
Next
End Function
End Class
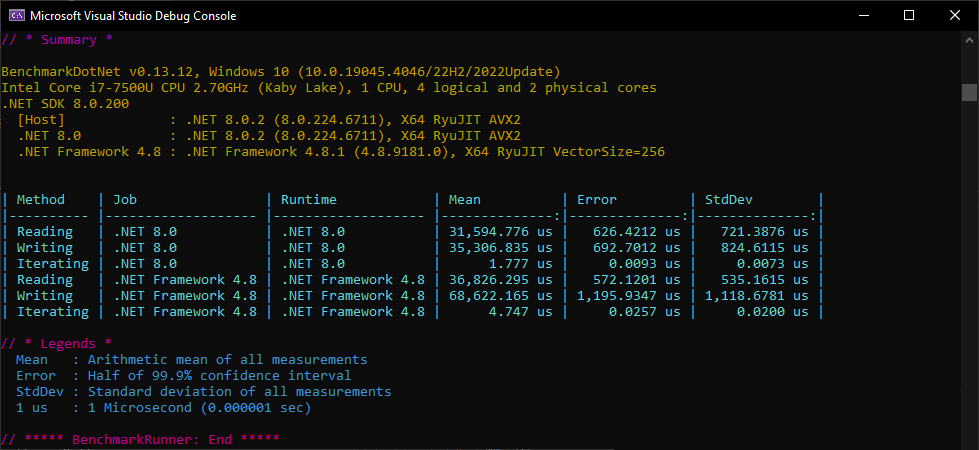
If the Free version limits are exceeded, the component will continue to work in Trial mode if the FreeLimitReached
event is handled as shown in the example.
For more information about Trial mode limitations, see the Evaluation and Licensing page.