Find and Replace Text in PowerPoint Slides
With GemBox.Presentation, you can find the text using string or regular expression and replace it with new content, as shown in the following example.
using GemBox.Presentation;
using System.Linq;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var presentation = PresentationDocument.Load("%#FindAndReplace.pptx%");
var slide = presentation.Slides[0];
slide.TextContent.Replace("companyName", "Acme Corporation");
var items = new string[][]
{
new string[] { "January", "$14.2M", "$1.6M", "$12.5M", "$2.3M" },
new string[] { "February", "$15.2M", "$0.6M", "$11.3M", "$4.3M" },
new string[] { "March", "$17.2M", "$0M", "$12.1M", "$52.3M" },
new string[] { "April", "$7.2M", "$1.6M", "$7.2M", "$0M" },
new string[] { "May", "$3.2M", "$1.6M", "$0M", "$3.2M" }
};
var table = slide.Content.Drawings.OfType<GraphicFrame>().First().Table;
// Clone the row with custom text tags (e.g. '@month', '@revenue', etc.).
for (int i = 0; i < items.Length - 1; i++)
table.Rows.AddClone(table.Rows[1]);
// Replace custom text tags with data.
for (int i = 0; i < items.Length; i++)
{
var row = table.Rows[i + 1];
var item = items[i];
row.TextContent.Replace("@month", item[0]);
row.TextContent.Replace("@revenue", item[1]);
row.TextContent.Replace("@cashExpense", item[2]);
row.TextContent.Replace("@operatingIncome", item[3]);
row.TextContent.Replace("@operatingExpense", item[4]);
}
// Find all text content with "0M" value in the table.
var zeroValueRanges = table.TextContent.Find("0M");
presentation.Save("Find And Replace.%OutputFileType%");
}
}
Imports GemBox.Presentation
Imports System.Linq
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim presentation = PresentationDocument.Load("%#FindAndReplace.pptx%")
Dim slide = presentation.Slides(0)
slide.TextContent.Replace("companyName", "Acme Corporation")
Dim items As String()() =
{
new String() {"January", "$14.2M", "$1.6M", "$12.5M", "$2.3M"},
new String() {"February", "$15.2M", "$0.6M", "$11.3M", "$4.3M"},
new String() {"March", "$17.2M", "$0M", "$12.1M", "$52.3M"},
new String() {"April", "$7.2M", "$1.6M", "$7.2M", "$0M"},
new String() {"May", "$3.2M", "$1.6M", "$0M", "$3.2M"}
}
Dim table = slide.Content.Drawings.OfType(Of GraphicFrame).First().Table
' Clone the row with custom text tags (e.g. '@month', '@revenue', etc.).
For i = 0 to items.Length - 2
table.Rows.AddClone(table.Rows(1))
Next
' Replace custom text tags with data.
for i = 0 to items.Length - 1
Dim row = table.Rows(i + 1)
Dim item = items(i)
row.TextContent.Replace("@month", item(0))
row.TextContent.Replace("@revenue", item(1))
row.TextContent.Replace("@cashExpense", item(2))
row.TextContent.Replace("@operatingIncome", item(3))
row.TextContent.Replace("@operatingExpense", item(4))
Next
' Find all text content with "0M" value in the table.
Dim zeroValueRanges = table.TextContent.Find("0M")
presentation.Save("Find And Replace.%OutputFileType%")
End Sub
End Module
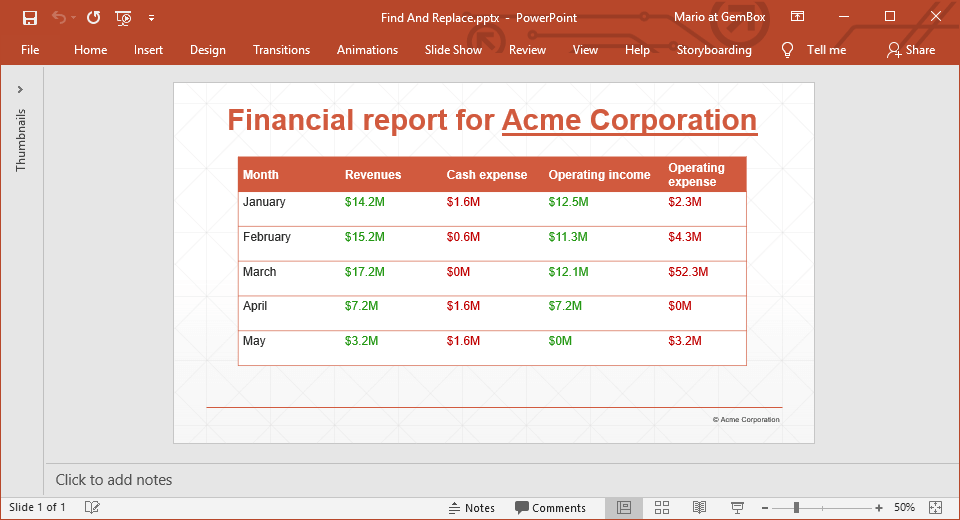
The text content of a presentation document is represented with a TextRange
class, which is exposed on the following content model classes:
PresentationDocument
SlideObject
, including all derived types, andSlideCollection
Drawing
, including all derived types, andDrawingCollection
Table
TableRow
andTableRowCollection
TableCell
andTableCellCollection
TextParagraph
andTextParagraphCollection
TextElement
, including all derived types, andTextElementCollection
You can also use the TextRange
class to achieve the following tasks:
- Deleting the text content with
TextRange.Delete
method. - Replacing the whole text content with
TextRange.LoadText
methods. - Inserting additional text on the content's
Start
orEnd
position withTextPosition.LoadText
methods. - Getting the text representation with
TextRange.ToString
method. - Searching the text content for specific text or regex with
TextRange.Find
methods.