Export PowerPoint in ASP.NET
The following example shows how you can use the GemBox.Presentation component to import web form data into a template presentation and export the resulting presentation to a browser in an ASP.NET application.
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Index.aspx.cs" Inherits="Index" %>
<!DOCTYPE html>
<html lang="en-US">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0, user-scalable=no">
<title>GemBox.Presentation Web Demo</title>
<style>
* {
font-family: Arial;
font-size: 10pt;
color: rgb(45,46,45);
}
h1 {
color: #0072bb;
font-size: 30pt;
text-align: center;
}
input[type="text"], textarea {
width: 100%;
}
.summaries {
font-size: 14pt;
}
.heading {
font-weight: bold;
font-size: 18pt;
color: rgb(209,90,62);
}
.title {
font-weight: bold;
font-size: 24pt;
}
hr {
display: block;
height: 1px;
border: 0;
border-top: 1px solid rgb(209,90,62);
margin: 1em 0;
padding: 0;
}
</style>
</head>
<body>
<h1>Create PowerPoint in ASP.NET</h1>
<form id="form1" runat="server">
<fieldset>
<legend>Presentation form:</legend>
<asp:TextBox ID="titleTextBox" runat="server" CssClass="title" Columns="50">ACME Corp - 4th Quarter Financial Results</asp:TextBox>
<hr>
<asp:TextBox ID="summaryHeadingTextBox" runat="server" CssClass="heading" Columns="30">4th Quarter Summary</asp:TextBox>
<br>
<br>
<br>
<asp:TextBox ID="summaryBulletsTextBox" runat="server" CssClass="summaries" TextMode="MultiLine" Wrap="False" Columns="70" Rows="5">3 new products/services in Research and Development.
Rollout planned for new division.
Campaigns targeting new markets.</asp:TextBox>
<hr>
<asp:TextBox ID="highlightsHeadingTextBox" runat="server" CssClass="heading" Columns="30">4th Quarter Financial Highlights</asp:TextBox>
<br>
<br>
<br>
<asp:GridView ID="highlightsGridView" runat="server" AutoGenerateColumns="False" BackColor="White" BorderColor="#CC9966" BorderStyle="None" BorderWidth="1px" CellPadding="4" AutoGenerateDeleteButton="True" AutoGenerateEditButton="True" Width="75%" OnRowCancelingEdit="highlightsGridView_RowCancelingEdit" OnRowDeleting="highlightsGridView_RowDeleting" OnRowEditing="highlightsGridView_RowEditing" OnRowUpdating="highlightsGridView_RowUpdating">
<Columns>
<asp:BoundField DataField="Name">
<ItemStyle Width="25%" />
</asp:BoundField>
<asp:BoundField DataField="Estimated" HeaderText="Estimated">
<ItemStyle Width="25%" />
</asp:BoundField>
<asp:BoundField DataField="Change" HeaderText="% Change">
<ItemStyle Width="25%" />
</asp:BoundField>
</Columns>
<FooterStyle BackColor="#FFFFCC" ForeColor="#330099" />
<HeaderStyle BackColor="#D15A3E" ForeColor="#2D2E2D" Font-Bold="False" />
<PagerStyle BackColor="#FFFFCC" ForeColor="#330099" HorizontalAlign="Center" />
<RowStyle BackColor="White" ForeColor="#2D2E2D" />
<SelectedRowStyle BackColor="#FFCC66" Font-Bold="True" ForeColor="#663399" />
<SortedAscendingCellStyle BackColor="#FEFCEB" />
<SortedAscendingHeaderStyle BackColor="#AF0101" />
<SortedDescendingCellStyle BackColor="#F6F0C0" />
<SortedDescendingHeaderStyle BackColor="#7E0000" />
</asp:GridView>
<asp:LinkButton ID="addRowLinkButton" runat="server" OnClick="addRowLinkButton_Click">Add Row</asp:LinkButton>
</fieldset>
<br>
<br>
<fieldset>
<legend>Output format:</legend>
<asp:DropDownList ID="outputFormatDropDownList" runat="server">
<asp:ListItem Value="pptx" Selected="True">PPTX</asp:ListItem>
<asp:ListItem Value="pdf">PDF</asp:ListItem>
</asp:DropDownList>
<br><br>
<asp:Button ID="generateButton" runat="server" Text="Generate" OnClick="generateButton_Click" />
</fieldset>
</form>
</body>
</html>
using GemBox.Presentation;
using System;
using System.Data;
using System.IO;
using System.Web.UI;
using System.Web.UI.WebControls;
public partial class Index : Page
{
protected void Page_Load(object sender, EventArgs e)
{
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
if (!this.Page.IsPostBack)
{
// Fill grid view with some default data.
var dataTable = new DataTable();
dataTable.Columns.Add("Name", typeof(string));
dataTable.Columns.Add("Estimated", typeof(string));
dataTable.Columns.Add("Change", typeof(string));
dataTable.Rows.Add("Revenues", "$14.2M", "(0.5%)");
dataTable.Rows.Add("Cash Expense", "$1.6M", "0.7%");
dataTable.Rows.Add("Operating Expense", "$12.5M", "0.3%");
dataTable.Rows.Add("Operating Income", "$2.3M", "(0.2%)");
this.Session["highlightsDataTable"] = dataTable;
this.SetDataBinding();
}
}
private static void UpdatePresentation(PresentationDocument presentation, string title, string summaryHeading, string summaryBullets, string highlightsHeading, DataTable highlightsDataTable)
{
// Populate the first slide with data.
var slide = presentation.Slides[0];
var shape = (Shape)slide.Content.Drawings[0];
if (!string.IsNullOrEmpty(title))
shape.Text.Paragraphs[0].AddRun(title.Replace('\v', ' ').Replace('\r', ' ').Replace('\n', ' '));
// Populate the second slide with data.
slide = presentation.Slides[1];
shape = (Shape)slide.Content.Drawings[0];
if (!string.IsNullOrEmpty(summaryHeading))
shape.Text.Paragraphs[0].AddRun(summaryHeading.Replace('\v', ' ').Replace('\r', ' ').Replace('\n', ' '));
shape = (Shape)slide.Content.Drawings[1];
shape.Text.Paragraphs.Clear();
var summaryBulletLines = summaryBullets.Split(new string[] { "\r\n" }, StringSplitOptions.RemoveEmptyEntries);
foreach (var summaryBulletLine in summaryBulletLines)
shape.Text.AddParagraph().AddRun(summaryBulletLine.Replace('\v', ' ').Replace('\r', ' ').Replace('\n', ' '));
// Populate the third slide with data.
slide = presentation.Slides[2];
shape = (Shape)slide.Content.Drawings[0];
if (!string.IsNullOrEmpty(highlightsHeading))
shape.Text.Paragraphs[0].AddRun(highlightsHeading.Replace('\v', ' ').Replace('\r', ' ').Replace('\n', ' '));
var frame = (GraphicFrame)slide.Content.Drawings[1];
var table = frame.Table;
for (int i = table.Rows.Count - 1; i > 0; --i)
table.Rows.RemoveAt(i);
foreach (DataRow highlightDataRow in highlightsDataTable.Rows)
{
var row = table.Rows.AddNew(table.Rows[0].Height);
var cell = row.Cells.AddNew();
var value = (string)highlightDataRow["Name"];
if (!string.IsNullOrEmpty(value))
cell.Text.AddParagraph().AddRun(value.Replace('\v', ' ').Replace('\r', ' ').Replace('\n', ' '));
cell = row.Cells.AddNew();
value = (string)highlightDataRow["Estimated"];
if (!string.IsNullOrEmpty(value))
cell.Text.AddParagraph().AddRun(value.Replace('\v', ' ').Replace('\r', ' ').Replace('\n', ' '));
cell = row.Cells.AddNew();
value = (string)highlightDataRow["Change"];
if (!string.IsNullOrEmpty(value))
cell.Text.AddParagraph().AddRun(value.Replace('\v', ' ').Replace('\r', ' ').Replace('\n', ' '));
}
}
protected void generateButton_Click(object sender, EventArgs e)
{
string path = Path.Combine(Request.PhysicalApplicationPath, "Template.pptx");
// Load template presentation.
var presentation = PresentationDocument.Load(path);
// Populate the template presentation with data.
UpdatePresentation(presentation, this.titleTextBox.Text, this.summaryHeadingTextBox.Text, this.summaryBulletsTextBox.Text, this.highlightsHeadingTextBox.Text, (DataTable)Session["highlightsDataTable"]);
// Stream the presentation to the browser.
string fileName = "Presentation." + this.outputFormatDropDownList.SelectedValue;
presentation.Save(this.Response, fileName);
}
protected void addRowLinkButton_Click(object sender, EventArgs e)
{
var dataTable = (DataTable)Session["highlightsDataTable"];
dataTable.Rows.Add("", "", "");
this.SetDataBinding();
}
protected void highlightsGridView_RowEditing(object sender, GridViewEditEventArgs e)
{
this.highlightsGridView.EditIndex = e.NewEditIndex;
this.SetDataBinding();
}
protected void highlightsGridView_RowUpdating(object sender, GridViewUpdateEventArgs e)
{
int i;
int rowIndex = e.RowIndex;
var dataTable = (DataTable)Session["highlightsDataTable"];
for (i = 0; i < dataTable.Columns.Count; i++)
{
var editTextBox = this.highlightsGridView.Rows[rowIndex].Cells[i + 1].Controls[0] as System.Web.UI.WebControls.TextBox;
if (editTextBox != null)
dataTable.Rows[rowIndex][i] = editTextBox.Text;
}
this.highlightsGridView.EditIndex = -1;
this.SetDataBinding();
}
protected void highlightsGridView_RowCancelingEdit(object sender, GridViewCancelEditEventArgs e)
{
this.highlightsGridView.EditIndex = -1;
this.SetDataBinding();
}
protected void highlightsGridView_RowDeleting(object sender, GridViewDeleteEventArgs e)
{
var dataTable = (DataTable)Session["highlightsDataTable"];
dataTable.Rows[e.RowIndex].Delete();
this.SetDataBinding();
}
private void SetDataBinding()
{
var dataTable = (DataTable)Session["highlightsDataTable"];
DataView dataView = dataTable.DefaultView;
this.highlightsGridView.DataSource = dataView;
dataView.AllowDelete = true;
this.highlightsGridView.DataBind();
}
}
Imports GemBox.Presentation
Imports System
Imports System.Data
Imports System.IO
Imports System.Web.UI
Imports System.Web.UI.WebControls
Partial Public Class Index
Inherits Page
Protected Sub Page_Load(sender As Object, e As EventArgs)
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
If Not Me.Page.IsPostBack Then
' Fill grid view with some default data.
Dim dataTable = New DataTable()
dataTable.Columns.Add("Name", GetType(String))
dataTable.Columns.Add("Estimated", GetType(String))
dataTable.Columns.Add("Change", GetType(String))
dataTable.Rows.Add("Revenues", "$14.2M", "(0.5%)")
dataTable.Rows.Add("Cash Expense", "$1.6M", "0.7%")
dataTable.Rows.Add("Operating Expense", "$12.5M", "0.3%")
dataTable.Rows.Add("Operating Income", "$2.3M", "(0.2%)")
Me.Session("highlightsDataTable") = dataTable
Me.SetDataBinding()
End If
End Sub
Private Shared Sub UpdatePresentation(presentation As PresentationDocument, title As String, summaryHeading As String, summaryBullets As String, highlightsHeading As String, highlightsDataTable As DataTable)
' Populate the first slide with data.
Dim slide = presentation.Slides(0)
Dim shape = DirectCast(slide.Content.Drawings(0), Shape)
If Not String.IsNullOrEmpty(title) Then
shape.Text.Paragraphs(0).AddRun(title.Replace(ControlChars.VerticalTab, " "c).Replace(ControlChars.Cr, " "c).Replace(ControlChars.Lf, " "c))
End If
' Populate the second slide with data.
slide = presentation.Slides(1)
shape = DirectCast(slide.Content.Drawings(0), Shape)
If Not String.IsNullOrEmpty(summaryHeading) Then
shape.Text.Paragraphs(0).AddRun(summaryHeading.Replace(ControlChars.VerticalTab, " "c).Replace(ControlChars.Cr, " "c).Replace(ControlChars.Lf, " "c))
End If
shape = DirectCast(slide.Content.Drawings(1), Shape)
shape.Text.Paragraphs.Clear()
Dim summaryBulletLines = summaryBullets.Split(New String() {vbCr & vbLf}, StringSplitOptions.RemoveEmptyEntries)
For Each summaryBulletLine In summaryBulletLines
shape.Text.AddParagraph().AddRun(summaryBulletLine.Replace(ControlChars.VerticalTab, " "c).Replace(ControlChars.Cr, " "c).Replace(ControlChars.Lf, " "c))
Next
' Populate the third slide with data.
slide = presentation.Slides(2)
shape = DirectCast(slide.Content.Drawings(0), Shape)
If Not String.IsNullOrEmpty(highlightsHeading) Then
shape.Text.Paragraphs(0).AddRun(highlightsHeading.Replace(ControlChars.VerticalTab, " "c).Replace(ControlChars.Cr, " "c).Replace(ControlChars.Lf, " "c))
End If
Dim frame = DirectCast(slide.Content.Drawings(1), GraphicFrame)
Dim table = frame.Table
For i As Integer = table.Rows.Count - 1 To 1 Step -1
table.Rows.RemoveAt(i)
Next
For Each highlightDataRow As DataRow In highlightsDataTable.Rows
Dim row = table.Rows.AddNew(table.Rows(0).Height)
Dim cell = row.Cells.AddNew()
Dim value = DirectCast(highlightDataRow("Name"), String)
If Not String.IsNullOrEmpty(value) Then
cell.Text.AddParagraph().AddRun(value.Replace(ControlChars.VerticalTab, " "c).Replace(ControlChars.Cr, " "c).Replace(ControlChars.Lf, " "c))
End If
cell = row.Cells.AddNew()
value = DirectCast(highlightDataRow("Estimated"), String)
If Not String.IsNullOrEmpty(value) Then
cell.Text.AddParagraph().AddRun(value.Replace(ControlChars.VerticalTab, " "c).Replace(ControlChars.Cr, " "c).Replace(ControlChars.Lf, " "c))
End If
cell = row.Cells.AddNew()
value = DirectCast(highlightDataRow("Change"), String)
If Not String.IsNullOrEmpty(value) Then
cell.Text.AddParagraph().AddRun(value.Replace(ControlChars.VerticalTab, " "c).Replace(ControlChars.Cr, " "c).Replace(ControlChars.Lf, " "c))
End If
Next
End Sub
Protected Sub generateButton_Click(sender As Object, e As EventArgs)
Dim path_ As String = Path.Combine(Request.PhysicalApplicationPath, "Template.pptx")
' Load template presentation.
Dim presentation = PresentationDocument.Load(path_)
' Populate the template presentation with data.
UpdatePresentation(presentation, Me.titleTextBox.Text, Me.summaryHeadingTextBox.Text, Me.summaryBulletsTextBox.Text, Me.highlightsHeadingTextBox.Text, DirectCast(Session("highlightsDataTable"), DataTable))
' Stream the presentation to the browser.
Dim fileName As String = "Presentation." + Me.outputFormatDropDownList.SelectedValue
presentation.Save(Me.Response, fileName)
End Sub
Protected Sub addRowLinkButton_Click(sender As Object, e As EventArgs)
Dim dataTable = DirectCast(Session("highlightsDataTable"), DataTable)
dataTable.Rows.Add("", "", "")
Me.SetDataBinding()
End Sub
Protected Sub highlightsGridView_RowEditing(sender As Object, e As GridViewEditEventArgs)
Me.highlightsGridView.EditIndex = e.NewEditIndex
Me.SetDataBinding()
End Sub
Protected Sub highlightsGridView_RowUpdating(sender As Object, e As GridViewUpdateEventArgs)
Dim i As Integer
Dim rowIndex As Integer = e.RowIndex
Dim dataTable = DirectCast(Session("highlightsDataTable"), DataTable)
For i = 0 To dataTable.Columns.Count - 1
Dim editTextBox = TryCast(Me.highlightsGridView.Rows(rowIndex).Cells(i + 1).Controls(0), System.Web.UI.WebControls.TextBox)
If editTextBox IsNot Nothing Then
dataTable.Rows(rowIndex)(i) = editTextBox.Text
End If
Next
Me.highlightsGridView.EditIndex = -1
Me.SetDataBinding()
End Sub
Protected Sub highlightsGridView_RowCancelingEdit(sender As Object, e As GridViewCancelEditEventArgs)
Me.highlightsGridView.EditIndex = -1
Me.SetDataBinding()
End Sub
Protected Sub highlightsGridView_RowDeleting(sender As Object, e As GridViewDeleteEventArgs)
Dim dataTable = DirectCast(Session("highlightsDataTable"), DataTable)
dataTable.Rows(e.RowIndex).Delete()
Me.SetDataBinding()
End Sub
Private Sub SetDataBinding()
Dim dataTable = DirectCast(Session("highlightsDataTable"), DataTable)
Dim dataView As DataView = dataTable.DefaultView
Me.highlightsGridView.DataSource = dataView
dataView.AllowDelete = True
Me.highlightsGridView.DataBind()
End Sub
Private Shared Function InlineAssignHelper(Of T)(ByRef target As T, value As T) As T
target = value
Return value
End Function
End Class
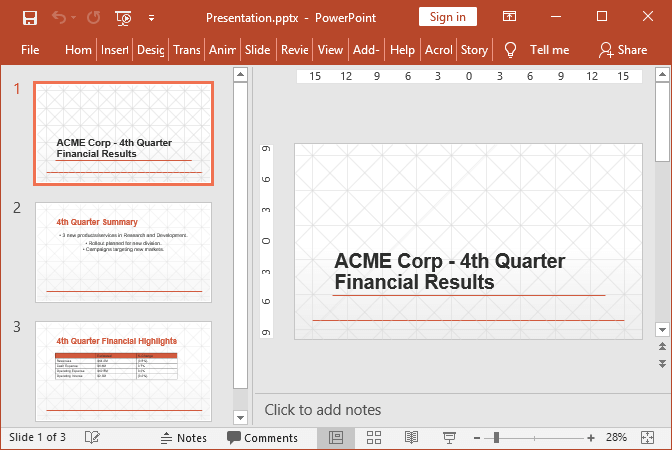
GemBox.Presentation does not use Office Automation, nor any other library - only .NET Framework, which, combined with it superior performance, makes it ideal for web applications, like ASP.NET. GemBox.Presentation supports reading and writing a presentation from / to a stream, so the presentation can easily be uploaded or streamed to a browser.