Printing PDF documents Programmatically
When working with many PDF documents, the best solution is to manage all the operations programmatically. That includes printing on a large scale since you can solve everything faster in just a few lines of code instead of doing everything manually. With the GemBox.Pdf API, you can print high-quality PDF documents in .NET, using C# and VB.NET.
In this article, you will learn how to print PDF files programmatically, send them to the default printer, or specify any other local or network printer connected to your machine. You will also learn how to do silent printing or provide a print dialog and print preview.
You can navigate through the following sections:
- Install and configure the GemBox.Pdf library
- How to print PDF documents in C# and VB.NET
- How to print multiple PDF files programmatically
- How to print specific pages of a PDF
- Print a PDF document using different paper trays in C# and VB.NET
- How to Print a PDF on Both Sides of Papers (Duplex)
- How to print multiple copies of a PDF in C# and VB.NET
- How to specify the printer name
- How to print a PDF in Black and White (Grayscale) in C# and VB.NET
Install and configure the GemBox.Pdf library
Before you start, you need to install GemBox.Pdf. The best way to do that is to install the NuGet Package by following these instructions:
Add the GemBox.Pdf component as a package using the following command from the NuGet Package Manager Console:
Install-Package GemBox.Pdf
After installing the GemBox.Pdf library, you must call the ComponentInfo.SetLicense method before using any other member of the library.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
In this tutorial, by using "FREE-LIMITED-KEY", you will be using GemBox's free mode. This mode allows you to use the library without purchasing a license, but with some limitations. If you purchased a license, you can replace "FREE-LIMITED-KEY" with your serial key.
You can check this page for a complete step-by-step guide to installing and setting up GemBox.Pdf in other ways.
How to print PDF documents in C# and VB.NET
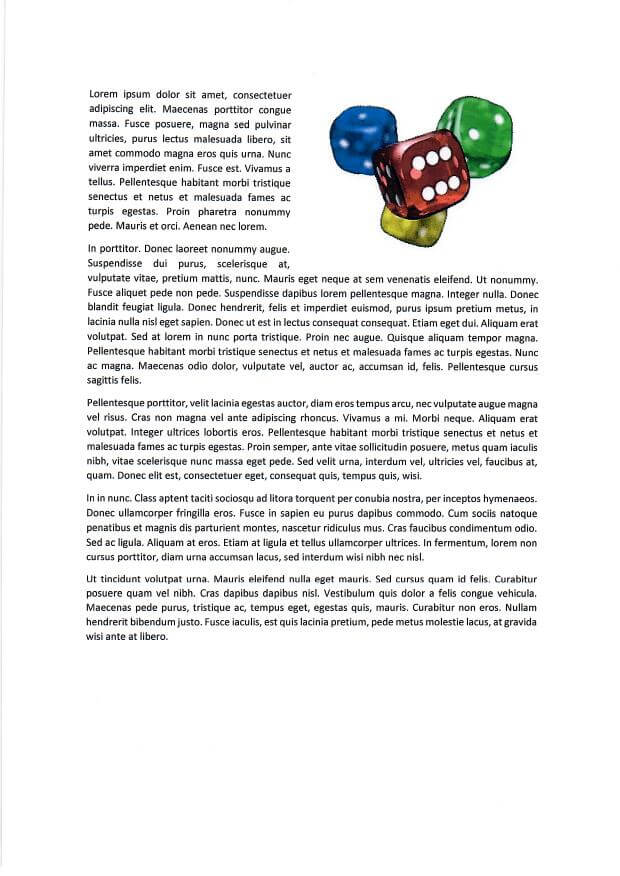
To automate the printing of PDF documents, you can follow the simple steps below:
- Load the input PDF document using the
PdfDocument
class. - Print the PDF file to a default printer with one of the
PdfDocument.Print
methods.
Verify the following code snippets to see how to print PDF files using C# and VB.NET:
How to print multiple PDF files programmatically
Depending on your work demands for PDF, it can be overwhelming and even impossible to handle each document, especially when you need to print dozens of different files simultaneously. As you can imagine, by extending the code presented in the previous section, you can easily print multiple files programmatically. To do that, simply follow these steps:
- Compose a list of the source file names you need to print.
- Iterate through the list of file names.
- Load the input PDF file using
PdfDocument.Load(String)
. - Print the files on the list with
PdfDocument.Print(String)
.
The following code snippet shows how to print multiple PDF files using C# and VB.NET:
// Define file names
var fileNames = new string[]
{
"PrintFile01.pdf",
"PrintFile02.pdf",
"PrintFile03.pdf"
};
// Define the printer name or set to 'null' for the default one
string printerName = null;
// Iterate through the list of file names
foreach (var fileName in fileNames)
// Load a PDF file
using (var document = PdfDocument.Load(fileName))
// Print the document to specified printer
document.Print(printerName);
' Define file names
Dim fileNames = New String(2) _
{
"PrintFile01.pdf",
"PrintFile02.pdf",
"PrintFile03.pdf"
}
' Define the printer name or set to 'Nothing' for the default one
Dim printerName As String = Nothing
' Iterate through the list of file names
For Each fileName In fileNames
' Load a PDF file
Using document = PdfDocument.Load(fileName)
' Print the document to specifed printer
document.Print(printerName)
End Using
Next
How to print specific pages of a PDF
With GemBox.Pdf API, you can also print page ranges, which can be handy depending on the kind of job you are performing. Follow the steps below to print specific pages:
- Load the file you want to print the pages from, using the
PdfDocument
class. - Select the printer by specifying the printer name, or set it to
null
to use the default one. - Set specific pages to print by defining a range with the
FromPage
andToPage
parameters. - Print the pages using the
PdfDocument.Print(printerName, printOptions)
method.
The code snippet below shows how to print specific pages of the document using C# and VB.NET:
using (var document = PdfDocument.Load("%#LoremIpsum.pdf%"))
{
// Define the printer name
var printerName = "Microsoft Print to PDF";
// Define the range of pages to print (second to third page in this case)
// NOTE: page range is zero-based which means that page numbers start with 0
var printOptions = new PrintOptions()
{
FromPage = 1,
ToPage = 2
};
// Print the pages
document.Print(printerName, printOptions);
}
Using document = PdfDocument.Load("%#LoremIpsum.pdf%")
' Define the printer name
Dim printerName = "Microsoft Print to PDF"
' Define the range of pages to print (second to third page in this case)
' NOTE: page range Is zero - based which means that page numbers start with 0
Dim PrintOptions = New PrintOptions() With
{
.FromPage = 1,
.ToPage = 2
}
' Print the pages
document.Print(printerName, PrintOptions)
End Using
Print a PDF document using different paper trays in C# and VB.NET
The following tutorial explains how to print PDF documents choosing the printer's paper source (tray).
- Load the Pdf document you wish to print.
- Create options for printing.
- Set the paper source (tray) with the
printTicket.PageMediaSize()
method. - Print the PDF document to the default printer.
And below you can check how the code will be like:
using (PdfDocument document = PdfDocument.Load("Print.pdf"))
{
// Creating a print ticket with customized paper source (tray)
var printTicket = new System.Printing.PrintTicket
{
PageMediaSize = new System.Printing.PageMediaSize(System.Printing.PageMediaSizeName.ISOA5)
};
// Print PDF document to default printer (e.g. 'Microsoft Print to Pdf').
string printerName = null;
// Create options for printing
var printOptions = new PrintOptions(printTicket.GetXmlStream());
document.Print(printerName, printOptions);
}
Using document = PdfDocument.Load("Print.pdf")
' Creating a print ticket with customized paper source (tray)
Dim printTicket = New Printing.PrintTicket With
{
.PageMediaSize = New Printing.PageMediaSize(Printing.PageMediaSizeName.ISOA5)
}
' Print PDF document to default printer (e.g. 'Microsoft Print to Pdf').
Dim printerName As String = Nothing
' Create options for printing
Dim printOptions = New PrintOptions(printTicket.GetXmlStream())
document.Print(printerName, printOptions)
End Using
How to print a PDF file on Both Sides of Papers (Duplex)
If your printer offers duplex printing capabilities, you have the option to print a PDF on both sides of the paper. To perform this action, follow these steps:
- Load the desired PDF document using the
PdfDocument.Load()
class. - Create a print ticket and define print job settings, set the Collation, and use
Duplexing.TwoSidedLongEdge
orDuplexing.TwoSidedShortEdge
, depending on your needs. - Convert the print ticket to GemBox.Pdf.PrintOptions.
- Print the document to the default printer.
using (var document = PdfDocument.Load("LoremIpsum.pdf"))
{
var printTicket = new PrintTicket
{
Collation = Collation.Collated,
Duplexing = Duplexing.TwoSidedLongEdge
};
var printOptions = new PrintOptions(printTicket.GetXmlStream());
document.Print(null, printOptions);
}
Using document = PdfDocument.Load("LoremIpsum.pdf")
Dim printTicket = New PrintTicket With
{
.Collation = Collation.Collated,
.Duplexing = Duplexing.TwoSidedLongEdge
}
Dim printOptions = New PrintOptions(printTicket.GetXmlStream())
document.Print(Nothing, printOptions)
End Using
Note that when working with PrintTicket
instances, you don't have to fetch the DefaultPrintTicket
from the desired printer's PrintQueue
. GemBox.Pdf will do that internally and merge it with the PrintTicket
you provided in your code. That's why, as shown in the example above, it's not necessary to specify all the options but only the ones you want to change.
How to print multiple copies of a PDF in C# and VB.NET
This tutorial explains how to print multiple copies of a PDF document:
- Load a PDF document using the
PdfDocument.Load()
class. - Create a print ticket and define print job settings, setting the desired
CopyCount
. - Call the
document.Print()
method to print the document.
using (var document = PdfDocument.Load("LoremIpsum.pdf"))
{
var printTicket = new PrintTicket
{
CopyCount = 2,
};
var printOptions = new PrintOptions(printTicket.GetXmlStream());
document.Print(null, printOptions);
}
Using document = PdfDocument.Load("LoremIpsum.pdf")
Dim printTicket = New PrintTicket With
{
.CopyCount = 2,
}
Dim PrintOptions = New PrintOptions(printTicket.GetXmlStream())
document.Print(Nothing, PrintOptions)
End Using
How to specify the printer name
If you have more than one printer, you can direct the printing of the PDF document to a designated printer by following these steps:
- Use the PdfDocument class to load the PDF document.
- Indicate the desired printer by assigning the name to the printerName string.
- Initiate the printing process by calling the document.Print(printerName) method.
using (var document = PdfDocument.Load("ExampleDocument.pdf"))
{
// Define the printer name
var printerName = "Microsoft Print to PDF";
// Print the pages
document.Print(printerName);
}
Using document = PdfDocument.Load("LoremIpsum.pdf")
' Define the printer name
Dim printerName = "Microsoft Print to PDF"
' Print the pages
document.Print(printerName, PrintOptions)
End Using
How to print a PDF in Black and White (Grayscale) in C# and VB.NET
The following steps outline how to programmatically print a PDF in black and white using C# and VB.NET:
- Load the desired PDF document.
- Create options for printing and a new printTicket.
- Set the output color of the document you want to print.
- Initiate the printing process setting up as the default printer.
using (PdfDocument document = PdfDocument.Load("Print.pdf"))
{
// Creating a print ticket with customized output color
var printTicket = new System.Printing.PrintTicket
{
OutputColor = System.Printing.OutputColor.Grayscale
};
// Print PDF document to default printer (e.g. 'Microsoft Print to Pdf').
string printerName = null;
// Create options for printing
var printOptions = new PrintOptions(printTicket.GetXmlStream());
document.Print(printerName, printOptions);
}
Using document = PdfDocument.Load("Print.pdf")
' Creating a print ticket with customized output color
Dim printTicket = New Printing.PrintTicket With
{
.OutputColor = Printing.OutputColor.Grayscale
}
' Print PDF document to default printer (e.g. 'Microsoft Print to Pdf').
Dim printerName As String = Nothing
' Create options for printing
Dim printOptions = New PrintOptions(printTicket.GetXmlStream())
document.Print(printerName, printOptions)
End Using
Conclusion
Now you know how to optimize your work by printing PDF files programmatically. Besides the actions for printing described in this article, you can use the GemBox.Pdf API to read, write, merge, and split PDF files and execute other low-level object manipulations in a very straightforward and quick way.
For more information regarding the GemBox.Pdf API, check the documentation pages. You can also see the Print PDF files in C# and VB.NET example for more information on printing PDF documents in WPF applications.