Read and write PDF properties
The following example shows how you can use GemBox.Pdf to set the Document Information Dictionary using the PdfDocument.Info
properties of an existing PDF document in your C# or VB.NET application.
using GemBox.Pdf;
using System;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
using (var document = PdfDocument.Load("%InputFileName%"))
{
// Get document properties.
var info = document.Info;
// Update document properties.
info.Title = "My Title";
info.Author = "My Author";
info.Subject = "My Subject";
info.Creator = "My Application";
// Update producer and date information, and disable their overriding.
info.Producer = "My Producer";
info.CreationDate = new DateTime(2023, 1, 1, 12, 0, 0);
info.ModificationDate = new DateTime(2023, 1, 1, 12, 0, 0);
document.SaveOptions.UpdateProducerInformation = false;
document.SaveOptions.UpdateDateInformation = false;
document.Save("Document Properties.pdf");
}
}
}
Imports GemBox.Pdf
Imports System
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Using document = PdfDocument.Load("%InputFileName%")
' Get document properties.
Dim info = document.Info
' Update document properties.
info.Title = "My Title"
info.Author = "My Author"
info.Subject = "My Subject"
info.Creator = "My Application"
' Update producer and date information, and disable their overriding.
info.Producer = "My Producer"
info.CreationDate = New DateTime(2023, 1, 1, 12, 0, 0)
info.ModificationDate = New DateTime(2023, 1, 1, 12, 0, 0)
document.SaveOptions.UpdateProducerInformation = False
document.SaveOptions.UpdateDateInformation = False
document.Save("Document Properties.pdf")
End Using
End Sub
End Module
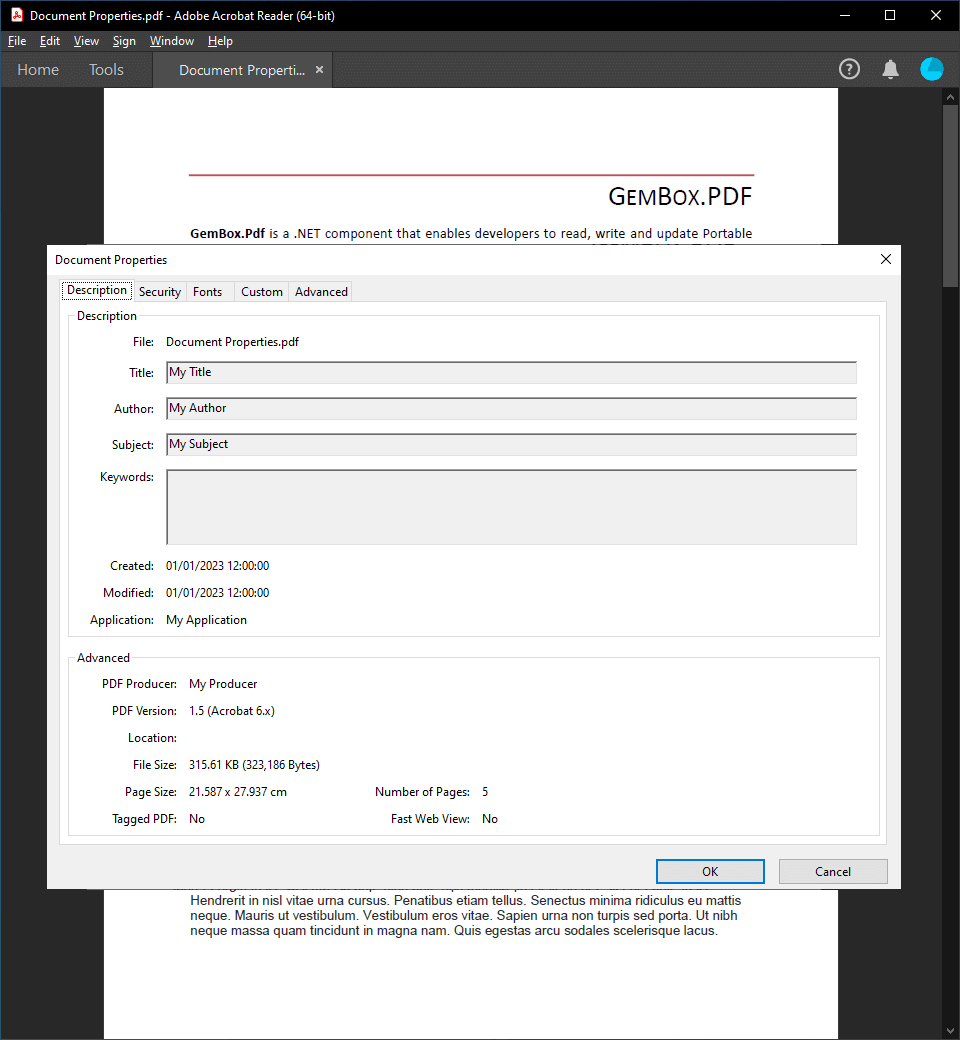
Custom Properties
Besides the properties available in PdfDocumentInformation
, you can also add custom properties. The following example shows how you can add or update custom properties in a PDF file.
using GemBox.Pdf;
using GemBox.Pdf.Objects;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
using (var document = PdfDocument.Load("%InputFileName%"))
{
// Get document properties dictionary.
var infoDictionary = document.Info.GetOrAddDictionary();
// Create or update custom properties.
infoDictionary[PdfName.Create("Custom Name 1")] = PdfString.Create("My Value 1");
infoDictionary[PdfName.Create("Custom Name 2")] = PdfString.Create("My Value 2");
document.Save("Custom Properties.pdf");
}
}
}
Imports GemBox.Pdf
Imports GemBox.Pdf.Objects
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Using document = PdfDocument.Load("%InputFileName%")
' Get document properties dictionary.
Dim infoDictionary = document.Info.GetOrAddDictionary()
' Create or update custom properties.
infoDictionary(PdfName.Create("Custom Name 1")) = PdfString.Create("My Value 1")
infoDictionary(PdfName.Create("Custom Name 2")) = PdfString.Create("My Value 2")
document.Save("Custom Properties.pdf")
End Using
End Sub
End Module
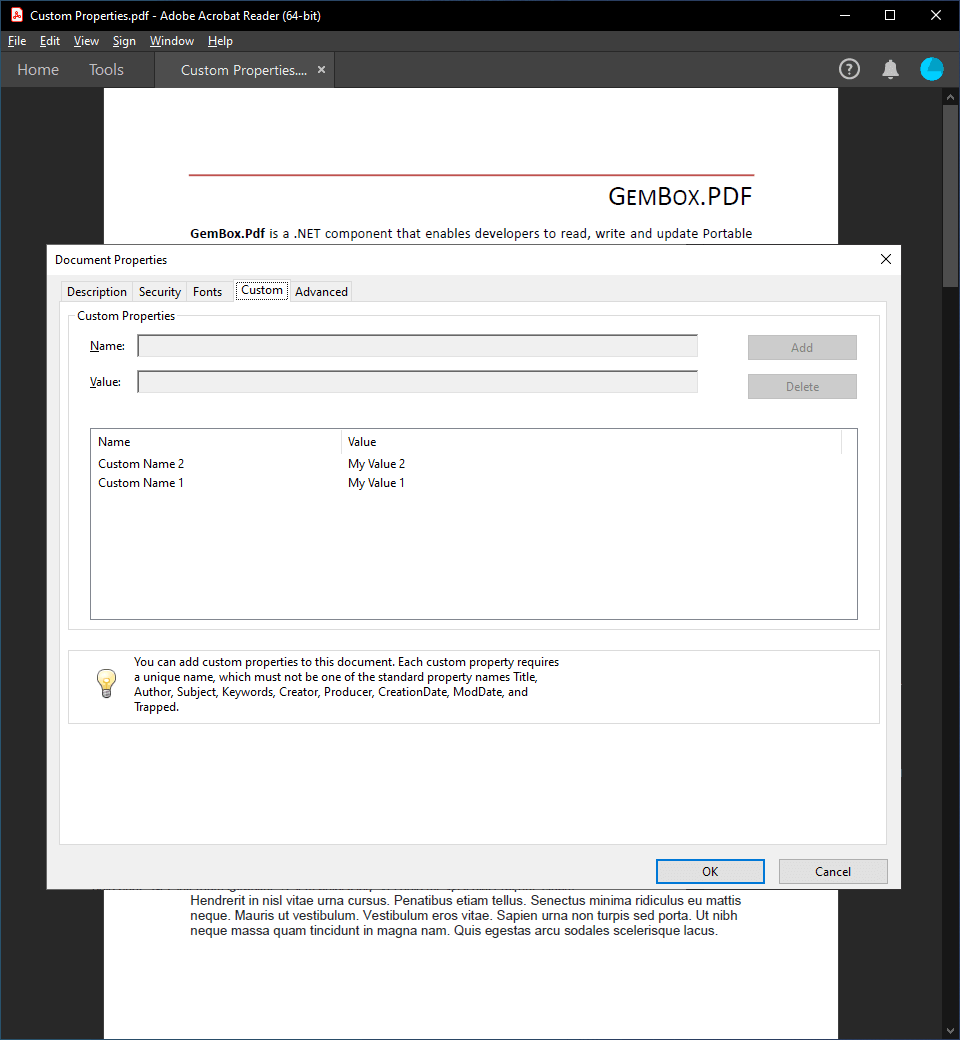
XMP Metadata
The following example shows how to create XMP metadata in a PDF file.
using GemBox.Pdf;
using System;
using System.Xml.Linq;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
using (var document = PdfDocument.Load("%InputFileName%"))
{
var metadata = document.Metadata;
var xmp = XNamespace.Get("http://ns.adobe.com/xap/1.0/");
var dc = XNamespace.Get("http://purl.org/dc/elements/1.1/");
var rdf = XNamespace.Get("http://www.w3.org/1999/02/22-rdf-syntax-ns#");
var xml = XNamespace.Xml;
metadata.Add(new XElement(xmp + "CreatorTool", "GemBox.Pdf for .NET"));
metadata.Add(new XElement(xmp + "CreateDate", DateTime.Now));
// Define the document title in multiple languages.
metadata.Add(new XElement(dc + "title",
new XElement(rdf + "Alt",
new XElement(rdf + "li", new XAttribute(xml + "lang", "x-default"), "My Title"),
new XElement(rdf + "li", new XAttribute(xml + "lang", "en"), "My Title"),
new XElement(rdf + "li", new XAttribute(xml + "lang", "es"), "Mi Título"),
new XElement(rdf + "li", new XAttribute(xml + "lang", "fr"), "Mon Titre"))));
document.Save("XMP Metadata.pdf");
}
}
}
Imports GemBox.Pdf
Imports System
Imports System.Xml.Linq
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Using document = PdfDocument.Load("%InputFileName%")
Dim metadata = document.Metadata
Dim xmp = XNamespace.Get("http://ns.adobe.com/xap/1.0/")
Dim dc = XNamespace.Get("http://purl.org/dc/elements/1.1/")
Dim rdf = XNamespace.Get("http://www.w3.org/1999/02/22-rdf-syntax-ns#")
Dim xml = XNamespace.Xml
metadata.Add(New XElement(xmp + "CreatorTool", "GemBox.Pdf for .NET"))
metadata.Add(New XElement(xmp + "CreateDate", DateTime.Now))
' Define the document title in multiple languages.
metadata.Add(New XElement(dc + "title",
New XElement(rdf + "Alt",
New XElement(rdf + "li", New XAttribute(xml + "lang", "x-default"), "My Title"),
New XElement(rdf + "li", New XAttribute(xml + "lang", "en"), "My Title"),
New XElement(rdf + "li", New XAttribute(xml + "lang", "es"), "Mi Título"),
New XElement(rdf + "li", New XAttribute(xml + "lang", "fr"), "Mon Titre"))))
document.Save("XMP Metadata.pdf")
End Using
End Sub
End Module
Besides the document itself, any individual document component (e.g., PdfObject
) may specify its metadata information. For example, PdfPage.Metadata
, PdfImage.Metadata
, and PdfOutline.Metadata
.
Note that if the same information is defined with Document Information Dictionary and XMP metadata, only the metadata information will be displayed in Adobe's Document Properties dialog.