Edit PDF files using incremental updates
The following example shows how you can load a PDF document, add a page with text, and save the changes using the incremental update capability.
using GemBox.Pdf;
using GemBox.Pdf.Content;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Load a PDF document from a file.
using (var document = PdfDocument.Load("%InputFileName%"))
{
// Add a page.
var page = document.Pages.Add();
// Write a text.
using (var formattedText = new PdfFormattedText())
{
formattedText.Append("Hello World again!");
page.Content.DrawText(formattedText, new PdfPoint(100, 700));
}
// Save all the changes made to the current PDF document using an incremental update.
document.Save();
}
}
}
Imports GemBox.Pdf
Imports GemBox.Pdf.Content
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Load a PDF document from a file.
Using document = PdfDocument.Load("%InputFileName%")
' Add a page.
Dim page = document.Pages.Add()
' Write a text.
Using formattedText = New PdfFormattedText()
formattedText.Append("Hello World again!")
page.Content.DrawText(formattedText, New PdfPoint(100, 700))
End Using
' Save all the changes made to the current PDF document using an incremental update.
document.Save()
End Using
End Sub
End Module
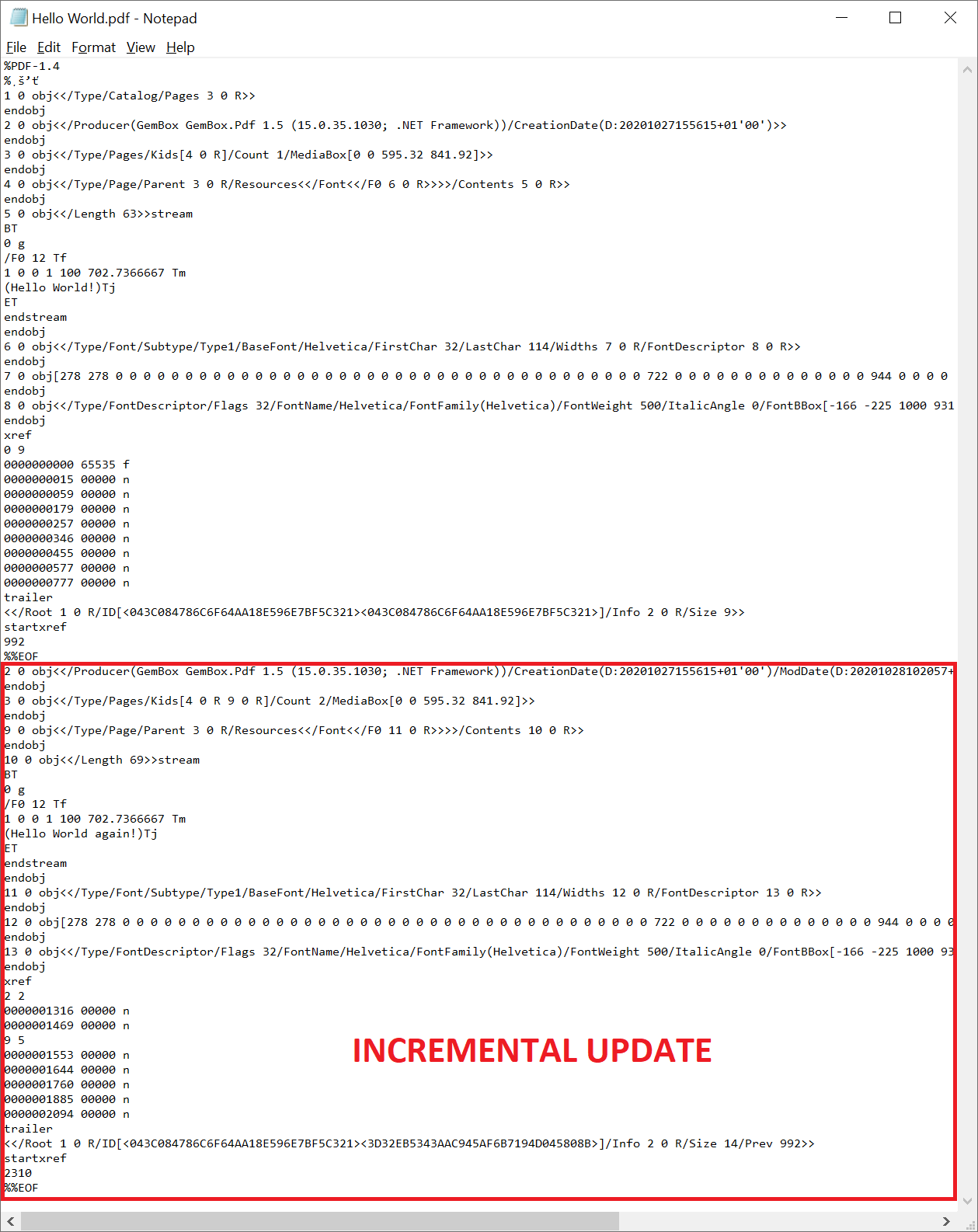
The PDF format allows modifications to be appended to a file, leaving the original data intact. The addendum appended when a file is incrementally updated contains only those objects that were actually added or modified.
Because the original contents of the document are still present in the file, it is possible to undo saved changes by deleting one or more addenda. The ability to recover the exact contents of an original document is critical when digitally signing a PDF file with multiple signatures.
For more information about PDF incremental update in GemBox.Pdf, see File Structure help page.