Create PDF in Docker container
You can use GemBox.Pdf inside docker containers that are running .NET Docker images. Note that this tutorial assumes that you have Docker Desktop installed.
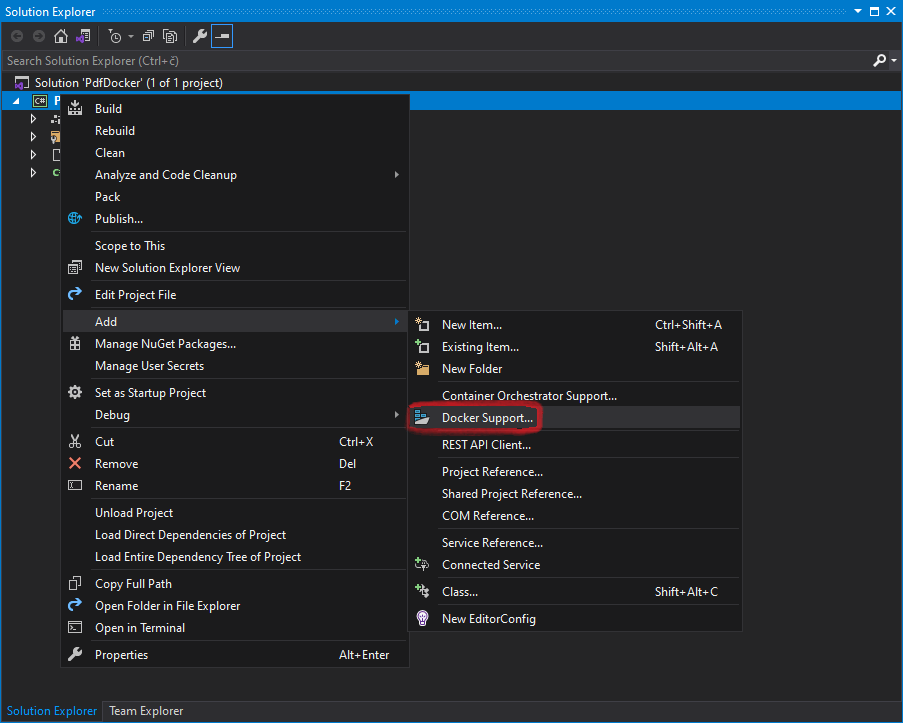
The following is a project file for the .NET Core application with added Docker support.
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<OutputType>Exe</OutputType>
<TargetFramework>net8.0</TargetFramework>
<DockerDefaultTargetOS>Linux</DockerDefaultTargetOS>
<DockerfileContext>.</DockerfileContext>
</PropertyGroup>
<ItemGroup>
<PackageReference Include="GemBox.Pdf" Version="*" />
<PackageReference Include="SkiaSharp.NativeAssets.Linux" Version="*" />
<PackageReference Include="HarfBuzzSharp.NativeAssets.Linux" Version="*" />
</ItemGroup>
</Project>
The following example shows how to create PDF files from Docker containers and configure Docker images with Dockerfile.
FROM mcr.microsoft.com/dotnet/runtime:8.0 AS base
WORKDIR /app
FROM mcr.microsoft.com/dotnet/sdk:8.0 AS build
WORKDIR /src
COPY ["PdfDocker.csproj", ""]
RUN dotnet restore "./PdfDocker.csproj"
COPY . .
WORKDIR "/src/."
RUN dotnet build "PdfDocker.csproj" -c Release -o /app/build
FROM build AS publish
RUN dotnet publish "PdfDocker.csproj" -c Release -o /app/publish /p:UseAppHost=false
FROM base AS final
# Update package sources to include supplemental packages (contrib archive area).
RUN sed -i 's/main/main contrib/g' /etc/apt/sources.list.d/debian.sources
# Downloads the package lists from the repositories.
RUN apt-get update
# Install font configuration.
RUN apt-get install -y fontconfig
# Install Microsoft TrueType core fonts.
RUN apt-get install -y ttf-mscorefonts-installer
# Or install Liberation TrueType fonts.
# RUN apt-get install -y fonts-liberation
# Or some other font package...
WORKDIR /app
COPY --from=publish /app/publish .
ENTRYPOINT ["dotnet", "PdfDocker.dll"]
using GemBox.Pdf;
using GemBox.Pdf.Content;
class Program
{
static void Main()
{
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Create new document.
using (var document = new PdfDocument())
{
// Add a page.
var page = document.Pages.Add();
// Write a text.
using (var formattedText = new PdfFormattedText())
{
formattedText.Append("Hello World!");
page.Content.DrawText(formattedText, new PdfPoint(100, 700));
}
// Save as PDF file.
document.Save("output.pdf");
}
}
}
Imports GemBox.Pdf
Imports GemBox.Pdf.Content
Module Program
Sub Main()
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Create new document.
Using document = New PdfDocument()
' Add a page.
Dim page = document.Pages.Add()
' Write a text.
Using formattedText = New PdfFormattedText()
formattedText.Append("Hello World!")
page.Content.DrawText(formattedText, New PdfPoint(100, 700))
End Using
' Save as PDF file.
document.Save("output.pdf")
End Using
End Sub
End Module
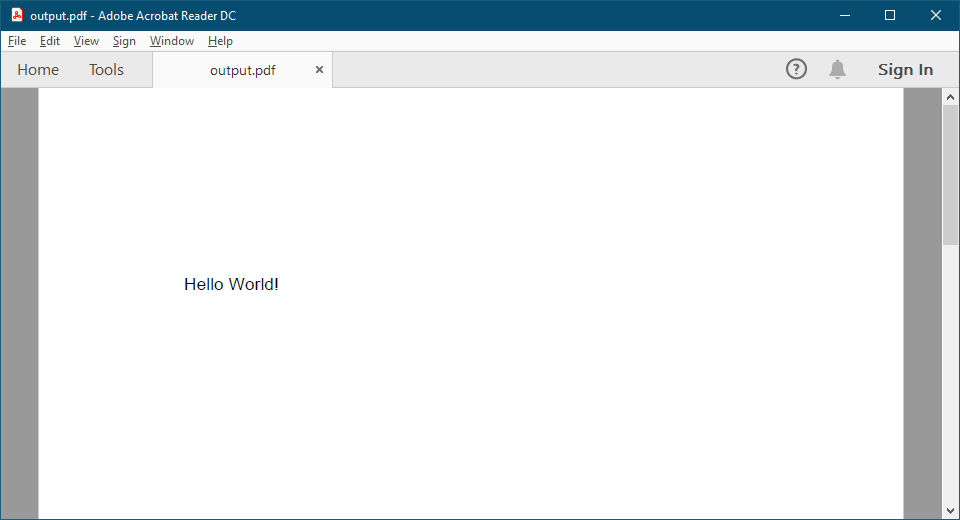
When creating PDF files, the font files need to be present in the container. The official Linux images for .NET won't have any fonts installed. So, you'll need to copy the necessary font files to the Linux container, or install a font package like ttf-mscorefonts-installer, or add them as custom fonts. You can use the full functionality of GemBox.Pdf on Unix systems, but with the following exceptions: These features currently have WPF dependencies which means they require a .NET Windows Desktop Runtime. However, we have plans for providing cross-platform support for them in future releases.Limitations on Linux or macOS
ConvertToImageSource
and ConvertToXpsDocument
methods.