Create PDF files from MAUI mobile app
GemBox.Pdf is a standalone .NET component with cross-platform support for processing PDFs. With the help of MAUI, you can use it on platforms like Android, iOS, Linux, and macOS for reading, writing, editing, and converting PDF files.
The following example shows how to create a PDF file in a mobile application.
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="PdfMaui.MainPage">
<ScrollView>
<VerticalStackLayout
Spacing="25"
Padding="30"
VerticalOptions="Center">
<Label Text="GemBox.Pdf Example"
HorizontalOptions="Center"
FontSize="Large"
Margin="0,0,0,30" />
<Label HorizontalOptions="Center"
Text="Document page count" />
<Label HorizontalOptions="Center"
FontSize="Large"
FontAttributes="Bold"
BindingContext="{x:Reference pages}"
Text="{Binding Value}" />
<Stepper x:Name="pages"
HorizontalOptions="Center"
Minimum="1"
Maximum="10"
Value="2" />
<ActivityIndicator x:Name="activity" />
<Button x:Name="button"
Text="Create document"
Clicked="Button_Clicked"/>
</VerticalStackLayout>
</ScrollView>
</ContentPage>
using GemBox.Pdf;
using GemBox.Pdf.Content;
namespace PdfMaui
{
public partial class MainPage : ContentPage
{
static MainPage()
{
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
}
public MainPage()
{
InitializeComponent();
}
private async Task<string> CreateDocumentAsync()
{
using var document = new PdfDocument();
for (int i = 0; i < pages.Value; ++i)
document.Pages.Add();
using (var formattedText = new PdfFormattedText())
{
// Set font family and size.
// All text appended next uses the specified font family and size.
formattedText.FontFamily = new PdfFontFamily("Times New Roman");
formattedText.FontSize = 24;
formattedText.AppendLine("Hello World");
document.Pages[0].Content.DrawText(formattedText, new PdfPoint(100, 100));
}
var image = PdfImage.Load(await FileSystem.OpenAppPackageFileAsync("dices.png"));
document.Pages[0].Content.DrawImage(image, new PdfPoint(100, 200));
var filePath = Path.Combine(Environment.GetFolderPath(Environment.SpecialFolder.MyDocuments), "Example.pdf");
await Task.Run(() => document.Save(filePath));
return filePath;
}
private async void Button_Clicked(object sender, EventArgs e)
{
button.IsEnabled = false;
activity.IsRunning = true;
try
{
var filePath = await CreateDocumentAsync();
await Launcher.OpenAsync(new OpenFileRequest(Path.GetFileName(filePath), new ReadOnlyFile(filePath)));
}
catch (Exception ex)
{
await DisplayAlert("Error", ex.Message, "Close");
}
activity.IsRunning = false;
button.IsEnabled = true;
}
}
}
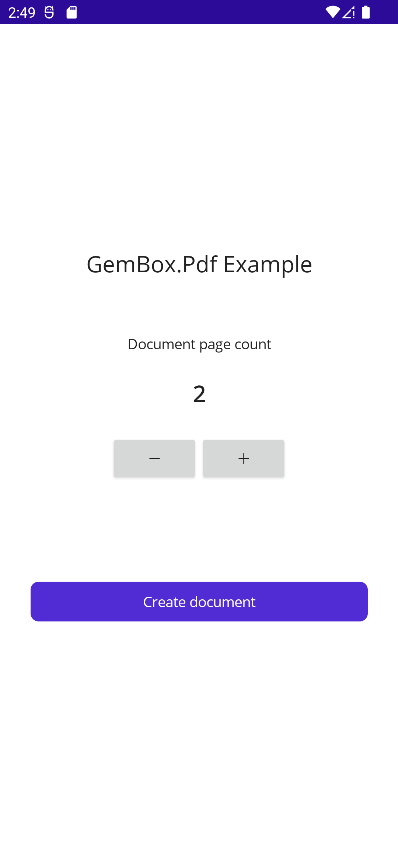
Limitations on Android or iOS
You can use the full functionality of GemBox.Pdf in MAUI applications, but with the following exceptions:
- Printing documents.
- Saving documents to XPS and image formats.
- Calling
ConvertToImageSource
andConvertToXpsDocument
methods.
These features currently depend on WPF and require a .NET Windows Desktop Runtime. However, we plan to add cross-platform support for them in future releases.