Get, create or edit bookmarks
The following example shows how you can use GemBox.Pdf to remove existing bookmarks (PdfDocument.Outlines
) and create new ones in a PDF document.
using GemBox.Pdf;
using System;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
using (var document = PdfDocument.Load("%InputFileName%"))
{
// Remove all bookmarks.
document.Outlines.Clear();
// Get the number of pages.
int numberOfPages = document.Pages.Count;
for (int i = 0; i < numberOfPages; i += 10)
{
// Add a new outline item (bookmark) at the end of the document outline collection.
var bookmark = document.Outlines.AddLast(string.Format("PAGES {0}-{1}", i + 1, Math.Min(i + 10, numberOfPages)));
// Set the explicit destination on the new outline item (bookmark).
bookmark.SetDestination(document.Pages[i], PdfDestinationViewType.FitRectangle, 0, 0, 100, 100);
for (int j = 0; j < Math.Min(10, numberOfPages - i); j++)
// Add a new outline item (bookmark) at the end of parent outline item (bookmark) and set the explicit destination.
bookmark.Outlines.AddLast(string.Format("PAGE {0}", i + j + 1)).SetDestination(document.Pages[i + j], PdfDestinationViewType.FitPage);
}
document.PageMode = PdfPageMode.UseOutlines;
document.Save("Outlines.pdf");
}
}
}
Imports GemBox.Pdf
Imports System
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Using document = PdfDocument.Load("%InputFileName%")
' Remove all bookmarks.
document.Outlines.Clear()
' Get the number of pages.
Dim numberOfPages As Integer = document.Pages.Count
For i As Integer = 0 To numberOfPages - 1 Step 10
' Add a new outline item (bookmark) at the end of the document outline collection.
Dim bookmark = document.Outlines.AddLast(String.Format("PAGES {0}-{1}", i + 1, Math.Min(i + 10, numberOfPages)))
' Set the explicit destination on the New outline item (bookmark).
bookmark.SetDestination(document.Pages(i), PdfDestinationViewType.FitRectangle, 0, 0, 100, 100)
For j As Integer = 0 To Math.Min(10, numberOfPages - i) - 1
' Add a new outline item (bookmark) at the end of parent outline item (bookmark) and set the explicit destination.
bookmark.Outlines.AddLast(String.Format("PAGE {0}", i + j + 1)).SetDestination(document.Pages(i + j), PdfDestinationViewType.FitPage)
Next
Next
document.PageMode = PdfPageMode.UseOutlines
document.Save("Outlines.pdf")
End Using
End Sub
End Module
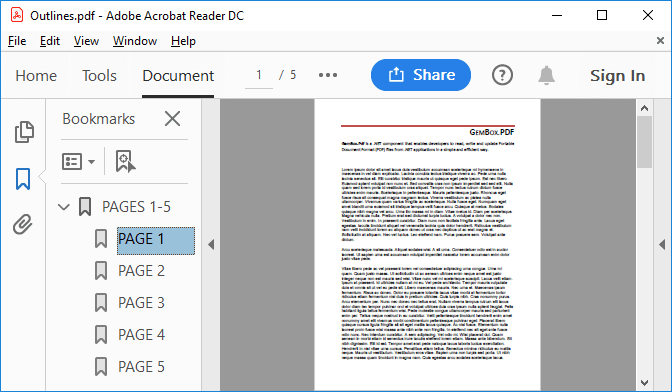