Merge PDF files
The following example shows how to merge (concatenate) PDF files using the GemBox.Pdf component.
using GemBox.Pdf;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// List of source file names.
var fileNames = new string[]
{
"%#MergeFile01.pdf%",
"%#MergeFile02.pdf%",
"%#MergeFile03.pdf%"
};
using (var document = new PdfDocument())
{
// Merge multiple PDF files into single PDF by loading source documents
// and cloning all their pages to destination document.
foreach (var fileName in fileNames)
using (var source = PdfDocument.Load(fileName))
document.Pages.Kids.AddClone(source.Pages);
document.Save("Merge Files.pdf");
}
}
}
Imports GemBox.Pdf
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' List of source file names.
Dim fileNames = New String() _
{
"%#MergeFile01.pdf%",
"%#MergeFile02.pdf%",
"%#MergeFile03.pdf%"
}
Using document = New PdfDocument()
' Merge multiple PDF files into single PDF by loading source documents
' and cloning all their pages to destination document.
For Each fileName In fileNames
Using source = PdfDocument.Load(fileName)
document.Pages.Kids.AddClone(source.Pages)
End Using
Next
document.Save("Merge Files.pdf")
End Using
End Sub
End Module
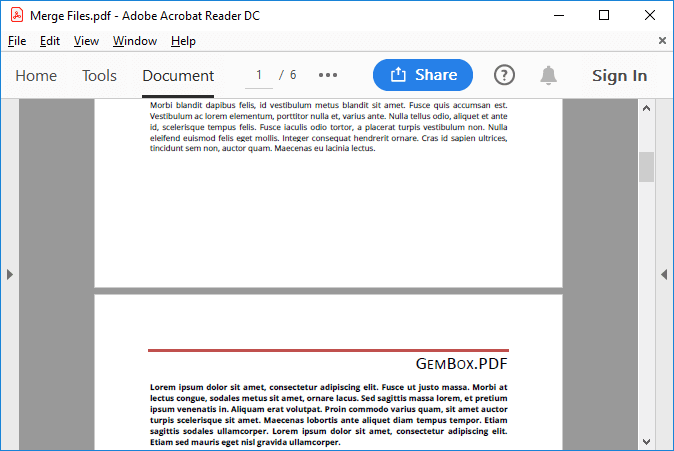
In the example above, you can see that the source PDF files are combined to clone the pages to a new PdfDocument
, and then save it to a PDF file.
If you want to clone a PDF page to an existing The following example shows how you can merge many PDF files into a single file by utilizing the The Also, with the With GemBox.Pdf, you can work efficiently with large PDF files or many PDF files. By using the lazy loading and incremental update features, you can utilize less memory when processing the documents.PdfDocument
, we recommend seeing the Cloning example.Merge a large number of PDF files
PdfDocument
's lazy loading and incremental update.using GemBox.Pdf;
using System.IO;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var files = Directory.EnumerateFiles("Merge Many Pdfs");
int fileCounter = 0;
int chunkSize = 50;
using (var document = new PdfDocument())
{
// Create output PDF file that will have large number of PDF files merged into it.
document.Save("Merged Files.pdf");
foreach (var file in files)
{
using (var source = PdfDocument.Load(file))
document.Pages.Kids.AddClone(source.Pages);
++fileCounter;
if (fileCounter % chunkSize == 0)
{
// Save the new pages that were added after the document was last saved.
document.Save();
// Clear previously parsed pages and thus free memory necessary for merging additional pages.
document.Unload();
}
}
// Save the last chunk of merged files.
document.Save();
}
}
}
Imports GemBox.Pdf
Imports System.IO
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim files = Directory.EnumerateFiles("Merge Many Pdfs")
Dim fileCounter As Integer = 0
Dim chunkSize As Integer = 50
Using document = New PdfDocument()
' Create output PDF file that will have large number of PDF files merged into it.
document.Save("Merged Files.pdf")
For Each file In files
Using source = PdfDocument.Load(file)
document.Pages.Kids.AddClone(source.Pages)
End Using
fileCounter += 1
If fileCounter Mod chunkSize = 0 Then
' Save the new pages that were added after the document was last saved.
document.Save()
' Clear previously parsed pages and thus free memory necessary for merging additional pages.
document.Unload()
End If
Next
' Save the last chunk of merged files.
document.Save()
End Using
End Sub
End Module
PdfPage
objects are loaded when requested for the first time and with PdfDocument.Unload
method, you can clear previously loaded pages.PdfDocument.Save
method you can save the changes made to the current document in the exact location from where the document was loaded or last saved.