Create and write PDF files in C# and VB.NET
With GemBox.Pdf, you can write rich text in PDF from your C# or VB.NET application.
Rich text is represented by PdfFormattedText
class that allows you to:
- Append text and line breaks.
- Set various formatting options, such as alignment, font (family, style, weight, stretch, and size), language, color, and opacity.
- Set the maximum width of text or the maximum width of each line of text, and line height.
- Set the text formatting mode (implementation of the text layout and text shaping).
- Get the formatted text width and height.
The following example shows how you can easily create a PDF document and write rich text to its first page.
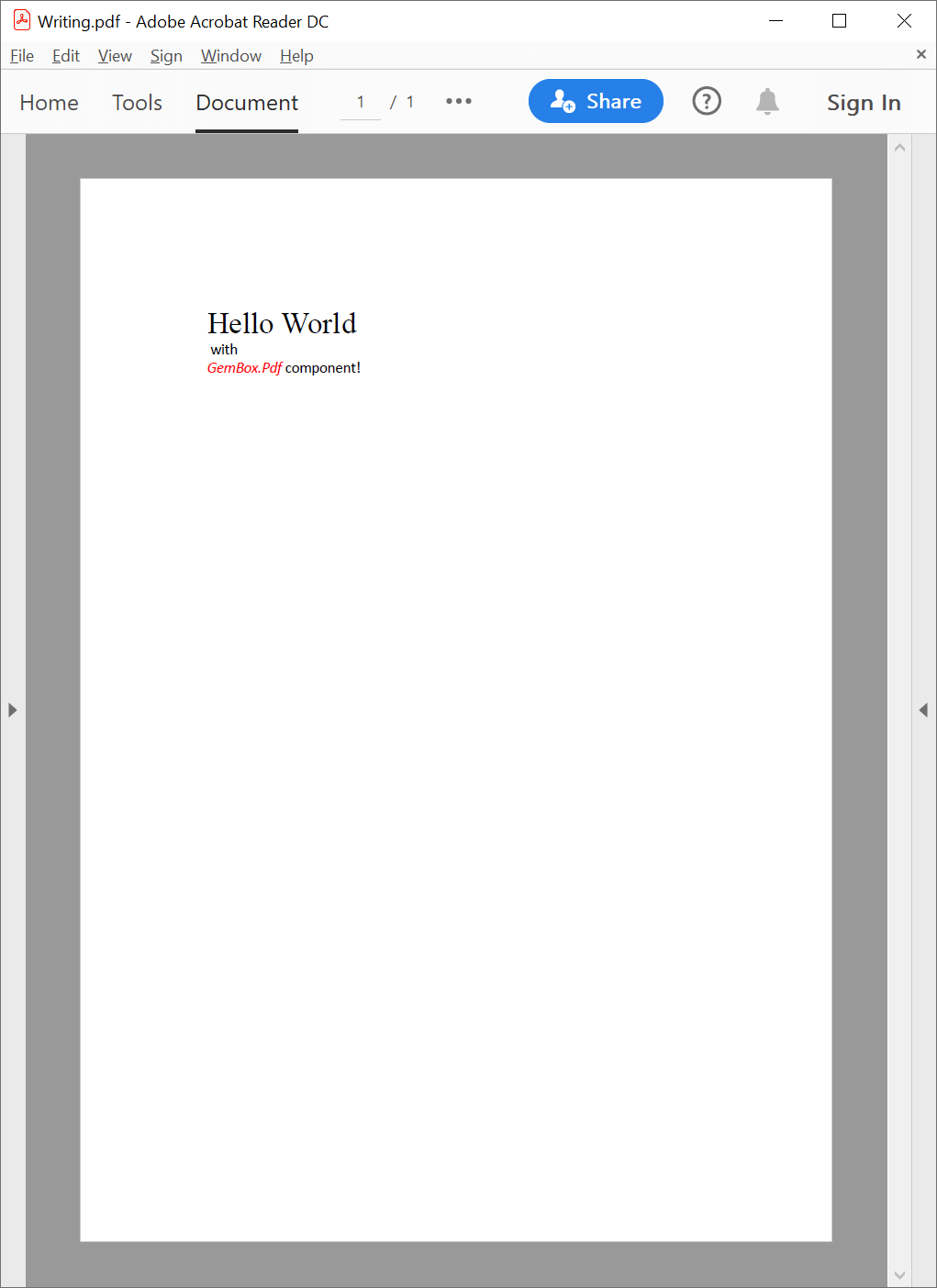
using GemBox.Pdf;
using GemBox.Pdf.Content;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
using (var document = new PdfDocument())
{
// Add a page.
var page = document.Pages.Add();
using (var formattedText = new PdfFormattedText())
{
// Set font family and size.
// All text appended next uses the specified font family and size.
formattedText.FontFamily = new PdfFontFamily("Times New Roman");
formattedText.FontSize = 24;
formattedText.AppendLine("Hello World");
// Reset font family and size for all text appended next.
formattedText.FontFamily = new PdfFontFamily("Calibri");
formattedText.FontSize = 12;
formattedText.AppendLine(" with ");
// Set font style and color for all text appended next.
formattedText.FontStyle = PdfFontStyle.Italic;
formattedText.Color = PdfColor.FromRgb(1, 0, 0);
formattedText.Append("GemBox.Pdf");
// Reset font style and color for all text appended next.
formattedText.FontStyle = PdfFontStyle.Normal;
formattedText.Color = PdfColor.FromRgb(0, 0, 0);
formattedText.Append(" component!");
// Set the location of the bottom-left corner of the text.
// We want top-left corner of the text to be at location (100, 100)
// from the top-left corner of the page.
// NOTE: In PDF, location (0, 0) is at the bottom-left corner of the page
// and the positive y axis extends vertically upward.
double x = 100, y = page.CropBox.Top - 100 - formattedText.Height;
// Draw text to the page.
page.Content.DrawText(formattedText, new PdfPoint(x, y));
}
document.Save("Writing.%OutputFileType%");
}
}
}
Imports GemBox.Pdf
Imports GemBox.Pdf.Content
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Using document = New PdfDocument()
' Add a page.
Dim page = document.Pages.Add()
Using formattedText = New PdfFormattedText()
' Set font family and size.
' All text appended next uses the specified font family and size.
formattedText.FontFamily = New PdfFontFamily("Times New Roman")
formattedText.FontSize = 24
formattedText.AppendLine("Hello World")
' Reset font family and size for all text appended next.
formattedText.FontFamily = New PdfFontFamily("Calibri")
formattedText.FontSize = 12
formattedText.AppendLine(" with ")
' Set font style and color for all text appended next.
formattedText.FontStyle = PdfFontStyle.Italic
formattedText.Color = PdfColor.FromRgb(1, 0, 0)
formattedText.Append("GemBox.Pdf")
' Reset font style and color for all text appended next.
formattedText.FontStyle = PdfFontStyle.Normal
formattedText.Color = PdfColor.FromRgb(0, 0, 0)
formattedText.Append(" component!")
' Set the location of the bottom-left corner of the text.
' We want top-left corner of the text to be at location (100, 100)
' from the top-left corner of the page.
' NOTE: In PDF, location (0, 0) is at the bottom-left corner of the page
' and the positive y axis extends vertically upward.
Dim x As Double = 100, y As Double = page.CropBox.Top - 100 - formattedText.Height
' Draw text to the page.
page.Content.DrawText(formattedText, New PdfPoint(x, y))
End Using
document.Save("Writing.%OutputFileType%")
End Using
End Sub
End Module
Fonts used in the above example are system fonts and should be located in the directory designated by your operating system. To use non-system fonts (either from a custom directory or embedded in an assembly), see the Private Fonts example.
The text from the above example is formatted to an infinite width. The next example shows how to format text to a finite width, with various alignments, and demonstrates how to use text size information to draw text at various positions. The following example shows how to align and position text at various positions in the PDF page. The above example shows how to position text on a single page. To position text on multiple pages, see the Header and Footer example. The text from the above example is just translated to various locations. The next example shows how to apply other transformations, such as rotation or scaling. The following example shows how to apply various transformations, such as rotation or scaling, to text drawn on a PDF page. The above example shows how to transform text on a single page. To transform text on multiple pages, see the Watermarks example. Previous examples showed how to write text in the Latin script. The next example shows how to write text in other scripts, such as Arabic, Hebrew, various Indic, and other complex scripts. The following example shows how to write text from complex scripts, such as Arabic, Hebrew, various Indic, and other. Note that formatting Left-to-Right complex scripts, such as various Indic scripts, and Right-to-Left complex scripts, such as Arabic, works via WPF (by setting the The following example shows how to format each text line into a different width and how to draw text line to a next page if it doesn't fit to the current page.Text alignment and positioning
using GemBox.Pdf;
using GemBox.Pdf.Content;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
using (var document = new PdfDocument())
{
var page = document.Pages.Add();
double margin = 10;
using (var formattedText = new PdfFormattedText())
{
formattedText.TextAlignment = PdfTextAlignment.Left;
formattedText.MaxTextWidth = 100;
formattedText.Append("This text is left aligned, ").
Append("placed in the top-left corner of the page and ").
Append("its width should not exceed 100 points.");
page.Content.DrawText(formattedText,
new PdfPoint(margin,
page.CropBox.Top - margin - formattedText.Height));
formattedText.Clear();
formattedText.TextAlignment = PdfTextAlignment.Center;
formattedText.MaxTextWidth = 200;
formattedText.Append("This text is center aligned, ").
Append("placed in the top-center part of the page ").
Append("and its width should not exceed 200 points.");
page.Content.DrawText(formattedText,
new PdfPoint((page.CropBox.Width - formattedText.MaxTextWidth) / 2,
page.CropBox.Top - margin - formattedText.Height));
formattedText.Clear();
formattedText.TextAlignment = PdfTextAlignment.Right;
formattedText.MaxTextWidth = 100;
formattedText.Append("This text is right aligned, ").
Append("placed in the top-right corner of the page ").
Append("and its width should not exceed 100 points.");
page.Content.DrawText(formattedText,
new PdfPoint(page.CropBox.Width - margin - formattedText.MaxTextWidth,
page.CropBox.Top - margin - formattedText.Height));
formattedText.Clear();
formattedText.TextAlignment = PdfTextAlignment.Left;
formattedText.MaxTextWidth = 100;
formattedText.Append("This text is left aligned, ").
Append("placed in the bottom-left corner of the page and ").
Append("its width should not exceed 100 points.");
page.Content.DrawText(formattedText,
new PdfPoint(margin,
margin));
formattedText.Clear();
formattedText.TextAlignment = PdfTextAlignment.Center;
formattedText.MaxTextWidth = 200;
formattedText.Append("This text is center aligned, ").
Append("placed in the bottom-center part of the page and ").
Append("its width should not exceed 200 points.");
page.Content.DrawText(formattedText,
new PdfPoint((page.CropBox.Width - formattedText.MaxTextWidth) / 2,
margin));
formattedText.Clear();
formattedText.TextAlignment = PdfTextAlignment.Right;
formattedText.MaxTextWidth = 100;
formattedText.Append("This text is right aligned, ").
Append("placed in the bottom-right corner of the page and ").
Append("its width should not exceed 100 points.");
page.Content.DrawText(formattedText,
new PdfPoint(page.CropBox.Width - margin - formattedText.MaxTextWidth,
margin));
formattedText.Clear();
formattedText.TextAlignment = PdfTextAlignment.Justify;
formattedText.MaxTextWidths = new double[] { 200, 150, 100 };
formattedText.Append("This text has justified alignment, ").
Append("is placed in the center of the page and ").
Append("its first line should not exceed 200 points, ").
Append("its second line should not exceed 150 points and ").
Append("its third and all other lines should not exceed 100 points.");
// Center the text based on the width of the most lines, which is formattedText.MaxTextWidths[2].
page.Content.DrawText(formattedText,
new PdfPoint((page.CropBox.Width - formattedText.MaxTextWidths[2]) / 2,
(page.CropBox.Height - formattedText.Height) / 2));
}
document.Save("Alignment and Positioning.%OutputFileType%");
}
}
}
Imports GemBox.Pdf
Imports GemBox.Pdf.Content
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Using document = New PdfDocument()
Dim page = document.Pages.Add()
Dim margin As Double = 10
Using formattedText = New PdfFormattedText()
formattedText.TextAlignment = PdfTextAlignment.Left
formattedText.MaxTextWidth = 100
formattedText.Append("This text is left aligned, ").
Append("placed in the top-left corner of the page and ").
Append("its width should not exceed 100 points.")
page.Content.DrawText(formattedText,
New PdfPoint(margin,
page.CropBox.Top - margin - formattedText.Height))
formattedText.Clear()
formattedText.TextAlignment = PdfTextAlignment.Center
formattedText.MaxTextWidth = 200
formattedText.Append("This text is center aligned, ").
Append("placed in the top-center part of the page ").
Append("and its width should not exceed 200 points.")
page.Content.DrawText(formattedText,
New PdfPoint((page.CropBox.Width - formattedText.MaxTextWidth) / 2,
page.CropBox.Top - margin - formattedText.Height))
formattedText.Clear()
formattedText.TextAlignment = PdfTextAlignment.Right
formattedText.MaxTextWidth = 100
formattedText.Append("This text is right aligned, ").
Append("placed in the top-right corner of the page ").
Append("and its width should not exceed 100 points.")
page.Content.DrawText(formattedText,
New PdfPoint(page.CropBox.Width - margin - formattedText.MaxTextWidth,
page.CropBox.Top - margin - formattedText.Height))
formattedText.Clear()
formattedText.TextAlignment = PdfTextAlignment.Left
formattedText.MaxTextWidth = 100
formattedText.Append("This text is left aligned, ").
Append("placed in the bottom-left corner of the page and ").
Append("its width should not exceed 100 points.")
page.Content.DrawText(formattedText,
New PdfPoint(margin,
margin))
formattedText.Clear()
formattedText.TextAlignment = PdfTextAlignment.Center
formattedText.MaxTextWidth = 200
formattedText.Append("This text is center aligned, ").
Append("placed in the bottom-center part of the page and ").
Append("its width should not exceed 200 points.")
page.Content.DrawText(formattedText,
New PdfPoint((page.CropBox.Width - formattedText.MaxTextWidth) / 2,
margin))
formattedText.Clear()
formattedText.TextAlignment = PdfTextAlignment.Right
formattedText.MaxTextWidth = 100
formattedText.Append("This text is right aligned, ").
Append("placed in the bottom-right corner of the page and ").
Append("its width should not exceed 100 points.")
page.Content.DrawText(formattedText,
New PdfPoint(page.CropBox.Width - margin - formattedText.MaxTextWidth,
margin))
formattedText.Clear()
formattedText.TextAlignment = PdfTextAlignment.Justify
formattedText.MaxTextWidths = New Double() {200, 150, 100}
formattedText.Append("This text has justified alignment, ").
Append("is placed in the center of the page and ").
Append("its first line should not exceed 200 points, ").
Append("its second line should not exceed 150 points and ").
Append("its third and all other lines should not exceed 100 points.")
' Center the text based on the width of the most lines, which is formattedText.MaxTextWidths(2).
page.Content.DrawText(formattedText,
New PdfPoint((page.CropBox.Width - formattedText.MaxTextWidths(2)) / 2,
(page.CropBox.Height - formattedText.Height) / 2))
End Using
document.Save("Alignment and Positioning.%OutputFileType%")
End Using
End Sub
End Module
Text transformations
using GemBox.Pdf;
using GemBox.Pdf.Content;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
using (var document = new PdfDocument())
{
var page = document.Pages.Add();
using (var formattedText = new PdfFormattedText())
{
var text = "Rotated by 30 degrees around origin.";
formattedText.Opacity = 0.2;
formattedText.Append(text);
var origin = new PdfPoint(50, 650);
page.Content.DrawText(formattedText, origin);
formattedText.Clear();
formattedText.Opacity = 1;
formattedText.Append(text);
var transform = PdfMatrix.Identity;
transform.Translate(origin.X, origin.Y);
transform.Rotate(30);
page.Content.DrawText(formattedText, transform);
formattedText.Clear();
text = "Rotated by 30 degrees around center.";
formattedText.Opacity = 0.2;
formattedText.Append(text);
origin = new PdfPoint(300, 650);
page.Content.DrawText(formattedText, origin);
formattedText.Clear();
formattedText.Opacity = 1;
formattedText.Append(text);
transform = PdfMatrix.Identity;
transform.Translate(origin.X, origin.Y);
transform.Rotate(30, formattedText.Width / 2, formattedText.Height / 2);
page.Content.DrawText(formattedText, transform);
formattedText.Clear();
text = "Scaled horizontally by 0.5 around origin.";
formattedText.Opacity = 0.2;
formattedText.Append(text);
origin = new PdfPoint(50, 500);
page.Content.DrawText(formattedText, origin);
formattedText.Clear();
formattedText.Opacity = 1;
formattedText.Append(text);
transform = PdfMatrix.Identity;
transform.Translate(origin.X, origin.Y);
transform.Scale(0.5, 1);
page.Content.DrawText(formattedText, transform);
formattedText.Clear();
text = "Scaled horizontally by 0.5 around center.";
formattedText.Opacity = 0.2;
formattedText.Append(text);
origin = new PdfPoint(300, 500);
page.Content.DrawText(formattedText, origin);
formattedText.Clear();
formattedText.Opacity = 1;
formattedText.Append(text);
transform = PdfMatrix.Identity;
transform.Translate(origin.X, origin.Y);
transform.Scale(0.5, 1, formattedText.Width / 2, formattedText.Height / 2);
page.Content.DrawText(formattedText, transform);
formattedText.Clear();
text = "Scaled vertically by 2 around origin.";
formattedText.Opacity = 0.2;
formattedText.Append(text);
origin = new PdfPoint(50, 400);
page.Content.DrawText(formattedText, origin);
formattedText.Clear();
formattedText.Opacity = 1;
formattedText.Append(text);
transform = PdfMatrix.Identity;
transform.Translate(origin.X, origin.Y);
transform.Scale(1, 2);
page.Content.DrawText(formattedText, transform);
formattedText.Clear();
text = "Scaled vertically by 2 around center.";
formattedText.Opacity = 0.2;
formattedText.Append(text);
origin = new PdfPoint(300, 400);
page.Content.DrawText(formattedText, origin);
formattedText.Clear();
formattedText.Opacity = 1;
formattedText.Append(text);
transform = PdfMatrix.Identity;
transform.Translate(origin.X, origin.Y);
transform.Scale(1, 2, formattedText.Width / 2, formattedText.Height / 2);
page.Content.DrawText(formattedText, transform);
formattedText.Clear();
text = "Rotated by 30 degrees around origin and ";
var text2 = "scaled horizontally by 0.5 and ";
var text3 = "vertically by 2 around origin.";
formattedText.Opacity = 0.2;
formattedText.AppendLine(text).AppendLine(text2).Append(text3);
origin = new PdfPoint(50, 200);
page.Content.DrawText(formattedText, origin);
formattedText.Clear();
formattedText.Opacity = 1;
formattedText.AppendLine(text).AppendLine(text2).Append(text3);
transform = PdfMatrix.Identity;
transform.Translate(origin.X, origin.Y);
transform.Rotate(30);
transform.Scale(0.5, 2);
page.Content.DrawText(formattedText, transform);
formattedText.Clear();
text = "Rotated by 30 degrees around center and ";
text2 = "scaled horizontally by 0.5 and ";
text3 = "vertically by 2 around center.";
formattedText.Opacity = 0.2;
formattedText.AppendLine(text).AppendLine(text2).Append(text3);
origin = new PdfPoint(300, 200);
page.Content.DrawText(formattedText, origin);
formattedText.Clear();
formattedText.Opacity = 1;
formattedText.AppendLine(text).AppendLine(text2).Append(text3);
transform = PdfMatrix.Identity;
transform.Translate(origin.X, origin.Y);
transform.Rotate(30, formattedText.Width / 2, formattedText.Height / 2);
transform.Scale(0.5, 2, formattedText.Width / 2, formattedText.Height / 2);
page.Content.DrawText(formattedText, transform);
}
document.Save("Transformations.%OutputFileType%");
}
}
}
Imports GemBox.Pdf
Imports GemBox.Pdf.Content
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Using document = New PdfDocument()
Dim page = document.Pages.Add()
Using formattedText = New PdfFormattedText()
Dim text = "Rotated by 30 degrees around origin."
formattedText.Opacity = 0.2
formattedText.Append(text)
Dim origin = New PdfPoint(50, 650)
page.Content.DrawText(formattedText, origin)
formattedText.Clear()
formattedText.Opacity = 1
formattedText.Append(text)
Dim transform = PdfMatrix.Identity
transform.Translate(origin.X, origin.Y)
transform.Rotate(30)
page.Content.DrawText(formattedText, transform)
formattedText.Clear()
text = "Rotated by 30 degrees around center."
formattedText.Opacity = 0.2
formattedText.Append(text)
origin = New PdfPoint(300, 650)
page.Content.DrawText(formattedText, origin)
formattedText.Clear()
formattedText.Opacity = 1
formattedText.Append(text)
transform = PdfMatrix.Identity
transform.Translate(origin.X, origin.Y)
transform.Rotate(30, formattedText.Width / 2, formattedText.Height / 2)
page.Content.DrawText(formattedText, transform)
formattedText.Clear()
text = "Scaled horizontally by 0.5 around origin."
formattedText.Opacity = 0.2
formattedText.Append(text)
origin = New PdfPoint(50, 500)
page.Content.DrawText(formattedText, origin)
formattedText.Clear()
formattedText.Opacity = 1
formattedText.Append(text)
transform = PdfMatrix.Identity
transform.Translate(origin.X, origin.Y)
transform.Scale(0.5, 1)
page.Content.DrawText(formattedText, transform)
formattedText.Clear()
text = "Scaled horizontally by 0.5 around center."
formattedText.Opacity = 0.2
formattedText.Append(text)
origin = New PdfPoint(300, 500)
page.Content.DrawText(formattedText, origin)
formattedText.Clear()
formattedText.Opacity = 1
formattedText.Append(text)
transform = PdfMatrix.Identity
transform.Translate(origin.X, origin.Y)
transform.Scale(0.5, 1, formattedText.Width / 2, formattedText.Height / 2)
page.Content.DrawText(formattedText, transform)
formattedText.Clear()
text = "Scaled vertically by 2 around origin."
formattedText.Opacity = 0.2
formattedText.Append(text)
origin = New PdfPoint(50, 400)
page.Content.DrawText(formattedText, origin)
formattedText.Clear()
formattedText.Opacity = 1
formattedText.Append(text)
transform = PdfMatrix.Identity
transform.Translate(origin.X, origin.Y)
transform.Scale(1, 2)
page.Content.DrawText(formattedText, transform)
formattedText.Clear()
text = "Scaled vertically by 2 around center."
formattedText.Opacity = 0.2
formattedText.Append(text)
origin = New PdfPoint(300, 400)
page.Content.DrawText(formattedText, origin)
formattedText.Clear()
formattedText.Opacity = 1
formattedText.Append(text)
transform = PdfMatrix.Identity
transform.Translate(origin.X, origin.Y)
transform.Scale(1, 2, formattedText.Width / 2, formattedText.Height / 2)
page.Content.DrawText(formattedText, transform)
formattedText.Clear()
text = "Rotated by 30 degrees around origin and "
Dim text2 = "scaled horizontally by 0.5 and "
Dim text3 = "vertically by 2 around origin."
formattedText.Opacity = 0.2
formattedText.AppendLine(text).AppendLine(text2).Append(text3)
origin = New PdfPoint(50, 200)
page.Content.DrawText(formattedText, origin)
formattedText.Clear()
formattedText.Opacity = 1
formattedText.AppendLine(text).AppendLine(text2).Append(text3)
transform = PdfMatrix.Identity
transform.Translate(origin.X, origin.Y)
transform.Rotate(30)
transform.Scale(0.5, 2)
page.Content.DrawText(formattedText, transform)
formattedText.Clear()
text = "Rotated by 30 degrees around center and "
text2 = "scaled horizontally by 0.5 and "
text3 = "vertically by 2 around center."
formattedText.Opacity = 0.2
formattedText.AppendLine(text).AppendLine(text2).Append(text3)
origin = New PdfPoint(300, 200)
page.Content.DrawText(formattedText, origin)
formattedText.Clear()
formattedText.Opacity = 1
formattedText.AppendLine(text).AppendLine(text2).Append(text3)
transform = PdfMatrix.Identity
transform.Translate(origin.X, origin.Y)
transform.Rotate(30, formattedText.Width / 2, formattedText.Height / 2)
transform.Scale(0.5, 2, formattedText.Width / 2, formattedText.Height / 2)
page.Content.DrawText(formattedText, transform)
End Using
document.Save("Transformations.%OutputFileType%")
End Using
End Sub
End Module
Text from complex scripts
using GemBox.Pdf;
using GemBox.Pdf.Content;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
using (var document = new PdfDocument())
{
var page = document.Pages.Add();
using (var formattedText = new PdfFormattedText())
{
formattedText.Language = new PdfLanguage("en-US");
formattedText.Font = new PdfFont("Calibri", 12);
formattedText.AppendLine("An example of a fully vocalised (vowelised or vowelled) Arabic ").
Append("from the Basmala: ");
formattedText.Language = new PdfLanguage("ar-SA");
formattedText.Font = new PdfFont("Arial", 24);
formattedText.Append("بِسْمِ ٱللَّٰهِ ٱلرَّحْمَٰنِ ٱلرَّحِيمِ");
formattedText.Language = new PdfLanguage("en-US");
formattedText.Font = new PdfFont("Calibri", 12);
formattedText.AppendLine(", which means: ").
Append("In the name of God, the All-Merciful, the Especially-Merciful.");
page.Content.DrawText(formattedText, new PdfPoint(50, 750));
formattedText.Clear();
formattedText.Append("An example of Hebrew: ");
formattedText.Language = new PdfLanguage("he-IL");
formattedText.Font = new PdfFont("Arial", 24);
formattedText.Append("מה קורה");
formattedText.Language = new PdfLanguage("en-US");
formattedText.Font = new PdfFont("Calibri", 12);
formattedText.AppendLine(", which means: What's going on, ").
Append("and ");
formattedText.Language = new PdfLanguage("he-IL");
formattedText.Font = new PdfFont("Arial", 24);
formattedText.Append("תודה לכולם");
formattedText.Language = new PdfLanguage("en-US");
formattedText.Font = new PdfFont("Calibri", 12);
formattedText.Append(", which means: Thank you all.");
page.Content.DrawText(formattedText, new PdfPoint(50, 650));
formattedText.Clear();
formattedText.LineHeight = 50;
formattedText.Append("An example of Thai: ");
formattedText.Language = new PdfLanguage("th-TH");
formattedText.Font = new PdfFont("Leelawadee UI", 16);
formattedText.AppendLine("ภัำ");
formattedText.Language = new PdfLanguage("en-US");
formattedText.Font = new PdfFont("Calibri", 12);
formattedText.Append("An example of Tamil: ");
formattedText.Language = new PdfLanguage("ta-IN");
formattedText.Font = new PdfFont("Nirmala UI", 16);
formattedText.AppendLine("போது");
formattedText.Language = new PdfLanguage("en-US");
formattedText.Font = new PdfFont("Calibri", 12);
formattedText.Append("An example of Bengali: ");
formattedText.Language = new PdfLanguage("be-IN");
formattedText.Font = new PdfFont("Nirmala UI", 16);
formattedText.AppendLine("আবেদনকারীর মাতার পিতার বর্তমান স্থায়ী ঠিকানা নমিনি নাম");
formattedText.Language = new PdfLanguage("en-US");
formattedText.Font = new PdfFont("Calibri", 12);
formattedText.Append("An example of Gujarati: ");
formattedText.Language = new PdfLanguage("gu-IN");
formattedText.Font = new PdfFont("Nirmala UI", 16);
formattedText.AppendLine("કાર્બન કેમેસ્ટ્રી");
formattedText.Language = new PdfLanguage("en-US");
formattedText.Font = new PdfFont("Calibri", 12);
formattedText.Append("An example of Osage: ");
formattedText.Language = new PdfLanguage("osa");
formattedText.Font = new PdfFont("Gadugi", 16);
formattedText.Append("𐓏𐓘𐓻𐓘𐓻𐓟 𐒻𐓟");
page.Content.DrawText(formattedText, new PdfPoint(50, 350));
}
document.Save("Complex scripts.%OutputFileType%");
}
}
}
Imports GemBox.Pdf
Imports GemBox.Pdf.Content
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Using document = New PdfDocument()
Dim page = document.Pages.Add()
Using formattedText = New PdfFormattedText()
formattedText.Language = New PdfLanguage("en-US")
formattedText.Font = New PdfFont("Calibri", 12)
formattedText.AppendLine("An example of a fully vocalised (vowelised or vowelled) Arabic ").
Append("from the Basmala: ")
formattedText.Language = New PdfLanguage("ar-SA")
formattedText.Font = New PdfFont("Arial", 24)
formattedText.Append("بِسْمِ ٱللَّٰهِ ٱلرَّحْمَٰنِ ٱلرَّحِيمِ")
formattedText.Language = New PdfLanguage("en-US")
formattedText.Font = New PdfFont("Calibri", 12)
formattedText.AppendLine(", which means: ").
Append("In the name of God, the All-Merciful, the Especially-Merciful.")
page.Content.DrawText(formattedText, New PdfPoint(50, 750))
formattedText.Clear()
formattedText.Append("An example of Hebrew: ")
formattedText.Language = New PdfLanguage("he-IL")
formattedText.Font = New PdfFont("Arial", 24)
formattedText.Append("מה קורה")
formattedText.Language = New PdfLanguage("en-US")
formattedText.Font = New PdfFont("Calibri", 12)
formattedText.AppendLine(", which means: What's going on, ").
Append("and ")
formattedText.Language = New PdfLanguage("he-IL")
formattedText.Font = New PdfFont("Arial", 24)
formattedText.Append("תודה לכולם")
formattedText.Language = New PdfLanguage("en-US")
formattedText.Font = New PdfFont("Calibri", 12)
formattedText.Append(", which means: Thank you all.")
page.Content.DrawText(formattedText, New PdfPoint(50, 650))
formattedText.Clear()
formattedText.LineHeight = 50
formattedText.Append("An example of Thai: ")
formattedText.Language = New PdfLanguage("th-TH")
formattedText.Font = New PdfFont("Leelawadee UI", 16)
formattedText.AppendLine("ภัำ")
formattedText.Language = New PdfLanguage("en-US")
formattedText.Font = New PdfFont("Calibri", 12)
formattedText.Append("An example of Tamil: ")
formattedText.Language = New PdfLanguage("ta-IN")
formattedText.Font = New PdfFont("Nirmala UI", 16)
formattedText.AppendLine("போது")
formattedText.Language = New PdfLanguage("en-US")
formattedText.Font = New PdfFont("Calibri", 12)
formattedText.Append("An example of Bengali: ")
formattedText.Language = New PdfLanguage("be-IN")
formattedText.Font = New PdfFont("Nirmala UI", 16)
formattedText.AppendLine("আবেদনকারীর মাতার পিতার বর্তমান স্থায়ী ঠিকানা নমিনি নাম")
formattedText.Language = New PdfLanguage("en-US")
formattedText.Font = New PdfFont("Calibri", 12)
formattedText.Append("An example of Gujarati: ")
formattedText.Language = New PdfLanguage("gu-IN")
formattedText.Font = New PdfFont("Nirmala UI", 16)
formattedText.AppendLine("કાર્બન કેમેસ્ટ્રી")
formattedText.Language = New PdfLanguage("en-US")
formattedText.Font = New PdfFont("Calibri", 12)
formattedText.Append("An example of Osage: ")
formattedText.Language = New PdfLanguage("osa")
formattedText.Font = New PdfFont("Gadugi", 16)
formattedText.Append("𐓏𐓘𐓻𐓘𐓻𐓟 𐒻𐓟")
page.Content.DrawText(formattedText, New PdfPoint(50, 350))
End Using
document.Save("Complex scripts.%OutputFileType%")
End Using
End Sub
End Module
PdfFormattedText.TextFormattingMode
property to PdfTextFormattingMode.WPF
, which is set by default when running on Windows) and via HarfBuzz text-shaping engine (by setting the PdfFormattedText.TextFormattingMode
property to PdfTextFormattingMode.HarfBuzz
, which is set by default when running on non-Windows operating systems and the HarfBuzzSharp package is referenced by the project). Also note that on non-Windows operating system your project should also reference an appropriate HarfBuzzSharp.NativeAssets.* NuGet package depending on the platform on which your application is running.Formatting text lines and breaking text across pages
using GemBox.Pdf;
using GemBox.Pdf.Content;
using System.IO;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
using (var document = new PdfDocument())
{
var page = document.Pages.Add();
double margins = 50;
double maxWidth = page.CropBox.Width - margins * 2;
double halfWidth = maxWidth / 2;
double maxHeight = page.CropBox.Height - margins * 2;
double heightOffset = maxHeight + margins;
using (var formattedText = new PdfFormattedText())
{
foreach (string line in File.ReadAllLines("%#LoremIpsum.txt%"))
formattedText.AppendLine(line);
double y = 0;
int lineIndex = 0, charIndex = 0;
bool useHalfWidth = false;
while (charIndex < formattedText.Length)
{
// Switch every 10 lines between full and half width.
if (lineIndex % 10 == 0)
useHalfWidth = !useHalfWidth;
var line = formattedText.FormatLine(charIndex, useHalfWidth ? halfWidth : maxWidth);
y += line.Height;
// If line cannot fit on the current page, write it on a new page.
bool lineCannotFit = y > maxHeight;
if (lineCannotFit)
{
page = document.Pages.Add();
y = line.Height;
}
page.Content.DrawText(line, new PdfPoint(margins, heightOffset - y));
++lineIndex;
charIndex += line.Length;
}
}
document.Save("Lines.%OutputFileType%");
}
}
}
Imports GemBox.Pdf
Imports GemBox.Pdf.Content
Imports System.IO
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Using document = New PdfDocument()
Dim page = document.Pages.Add()
Dim margins As Double = 50
Dim maxWidth As Double = page.CropBox.Width - margins * 2
Dim halfWidth As Double = maxWidth / 2
Dim maxHeight As Double = page.CropBox.Height - margins * 2
Dim heightOffset As Double = maxHeight + margins
Using formattedText = New PdfFormattedText()
For Each line In File.ReadAllLines("%#LoremIpsum.txt%")
formattedText.AppendLine(line)
Next
Dim y As Double = 0
Dim lineIndex As Integer = 0, charIndex As Integer = 0
Dim useHalfWidth As Boolean = False
While charIndex < formattedText.Length
' Switch every 10 lines between full and half width.
If lineIndex Mod 10 = 0 Then useHalfWidth = Not useHalfWidth
Dim line = formattedText.FormatLine(charIndex, If(useHalfWidth, halfWidth, maxWidth))
y += line.Height
' If line cannot fit on the current page, write it on a new page.
Dim lineCannotFit As Boolean = y > maxHeight
If lineCannotFit Then
page = document.Pages.Add()
y = line.Height
End If
page.Content.DrawText(line, New PdfPoint(margins, heightOffset - y))
lineIndex += 1
charIndex += line.Length
End While
End Using
document.Save("Lines.%OutputFileType%")
End Using
End Sub
End Module