Read PDF interactive form fields in C# and VB.NET
The following example demonstrates how you can enumerate all interactive form fields and read their name, type, and value. In GemBox.Pdf, interactive form is represented with PdfInteractiveForm
class and all field types share the same PdfField
base class.
using GemBox.Pdf;
using GemBox.Pdf.Forms;
using System;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
using (var document = PdfDocument.Load("%InputFileName%"))
{
Console.WriteLine(" Field Name | Field Type | Field Value ");
Console.WriteLine(new string('-', 50));
foreach (var field in document.Form.Fields)
{
string value = (field.Value ?? string.Empty).ToString().Replace("\r", ", ");
Console.WriteLine($" {field.Name,-15} | {field.FieldType,-15} | {value}");
}
}
}
}
Imports GemBox.Pdf
Imports GemBox.Pdf.Forms
Imports System
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Using document = PdfDocument.Load("%InputFileName%")
Console.WriteLine(" Field Name | Field Type | Field Value ")
Console.WriteLine(New String("-"c, 50))
For Each field In document.Form.Fields
Dim value As String = (If(field.Value, String.Empty)).ToString().Replace(vbCr, ", ")
Console.WriteLine($" {field.Name,-15} | {field.FieldType,-15} | {value}")
Next
End Using
End Sub
End Module
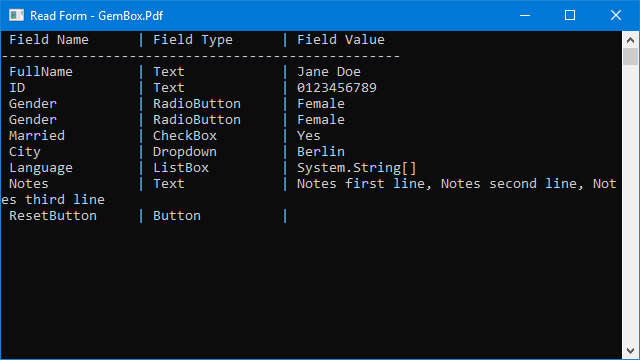
Note that multiple PdfField
instances can have the same name which means that these fields are grouped and represent the same interactive form field. The most common case is for PdfRadioButtonField
, each radio button of the same name represents a different value you can select within one interactive form field.
The following shows how you can read additional field properties in a PDF document and how to read exportable values or choices for fields with the same name.
using GemBox.Pdf;
using GemBox.Pdf.Forms;
using System;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
using (var document = PdfDocument.Load("%#FormFilled.pdf%"))
{
Console.WriteLine(" Field Name | Field Data ");
Console.WriteLine(new string('-', 50));
foreach (var field in document.Form.Fields)
{
switch (field.FieldType)
{
case PdfFieldType.RadioButton:
var radioButton = (PdfRadioButtonField)field;
Console.Write($" {radioButton.Name,12} [PdfRadioButtonField] | ");
Console.WriteLine($"{radioButton.Choice} [{(radioButton.Checked ? "Checked" : "Unchecked")}]");
break;
case PdfFieldType.CheckBox:
var checkBox = (PdfCheckBoxField)field;
Console.Write($" {checkBox.Name,15} [PdfCheckBoxField] | ");
Console.WriteLine($"{checkBox.ExportValue} [{(checkBox.Checked ? "Checked" : "Unchecked")}]");
break;
case PdfFieldType.Dropdown:
var dropdown = (PdfDropdownField)field;
Console.Write($" {dropdown.Name,15} [PdfDropdownField] | ");
Console.WriteLine(dropdown.SelectedItem);
break;
case PdfFieldType.ListBox:
var listBox = (PdfListBoxField)field;
Console.Write($" {listBox.Name,16} [PdfListBoxField] | ");
Console.WriteLine(string.Join(", ", listBox.SelectedItems));
break;
}
}
}
}
}
Imports GemBox.Pdf
Imports GemBox.Pdf.Forms
Imports System
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Using document = PdfDocument.Load("%#FormFilled.pdf%")
Console.WriteLine(" Field Name | Field Data ")
Console.WriteLine(New String("-"c, 50))
For Each field In document.Form.Fields
Select Case field.FieldType
Case PdfFieldType.RadioButton
Dim radioButton = CType(field, PdfRadioButtonField)
Console.Write($" {radioButton.Name,12} [PdfRadioButtonField] | ")
Console.WriteLine($"{radioButton.Choice} [{If(radioButton.Checked, "Checked", "Unchecked")}]")
Case PdfFieldType.CheckBox
Dim checkBox = CType(field, PdfCheckBoxField)
Console.Write($" {checkBox.Name,15} [PdfCheckBoxField] | ")
Console.WriteLine($"{checkBox.ExportValue} [{If(checkBox.Checked, "Checked", "Unchecked")}]")
Case PdfFieldType.Dropdown
Dim dropdown = CType(field, PdfDropdownField)
Console.Write($" {dropdown.Name,15} [PdfDropdownField] | ")
Console.WriteLine(dropdown.SelectedItem)
Case PdfFieldType.ListBox
Dim listBox = CType(field, PdfListBoxField)
Console.Write($" {listBox.Name,16} [PdfListBoxField] | ")
Console.WriteLine(String.Join(", ", listBox.SelectedItems))
End Select
Next
End Using
End Sub
End Module
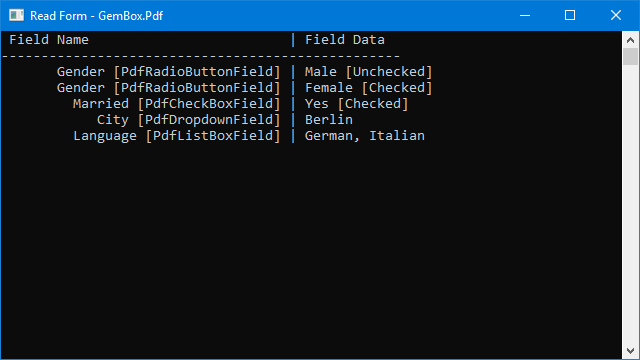