Convert PDF files to image formats
The following example shows how to convert a PDF page to a JPEG image of a specified size using the GemBox.Pdf .NET library.
using GemBox.Pdf;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Load a PDF document.
using (var document = PdfDocument.Load("%InputFileName%"))
{
// Create image save options.
var imageOptions = new ImageSaveOptions(ImageSaveFormat.Jpeg)
{
PageNumber = 0, // Select the first PDF page.
Width = 1240 // Set the image width and keep the aspect ratio.
};
// Save a PDF document to a JPEG file.
document.Save("Output.jpg", imageOptions);
}
}
}
Imports GemBox.Pdf
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Load a PDF document.
Using document = PdfDocument.Load("%InputFileName%")
' Create image save options.
Dim imageOptions As New ImageSaveOptions(ImageSaveFormat.Jpeg) With
{
.PageNumber = 0, ' Select the first PDF page.
.Width = 1240 ' Set the image width and keep the aspect ratio.
}
' Save a PDF document to a JPEG file.
document.Save("Output.jpg", imageOptions)
End Using
End Sub
End Module
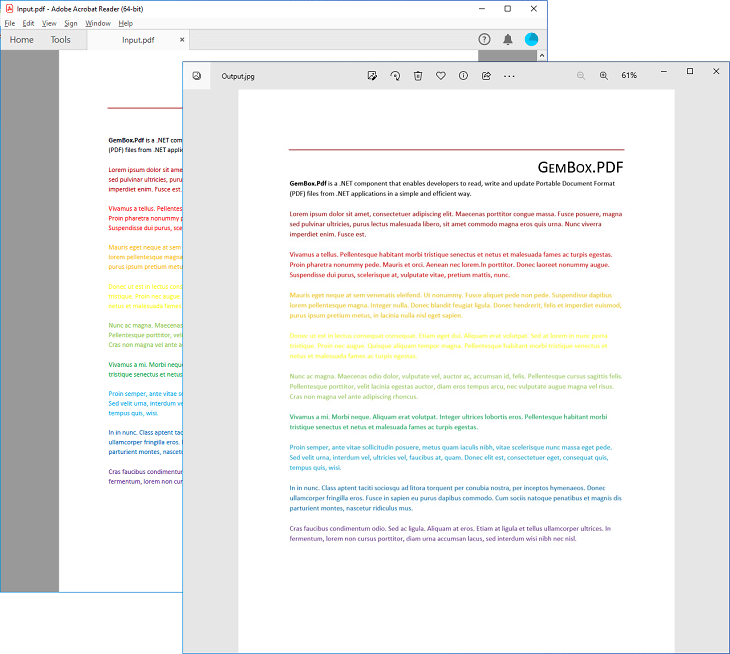
With GemBox.Pdf you can save your document to image formats such as PNG, JPEG, BMP, WMP, GIF, and TIFF. The following example shows how you can save each page from a PDF document as a separate PNG or JPEG image. Note that image formats that support transparency, like PNG, will have pages rendered with a transparent background, and those that don't support transparency, like JPEG, will have a white background. Also, if you want to save just a part of the page to an image, you can specify the desired area using the The following example shows to can convert all PDF pages to a multi-page TIFF image.Convert a PDF file to multiple images
using GemBox.Pdf;
using GemBox.Pdf.Content;
using System.IO;
using System.IO.Compression;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Load a PDF document.
using (var document = PdfDocument.Load("%InputFileName%"))
{
var imageOptions = new ImageSaveOptions(ImageSaveFormat.Png);
// Create a ZIP file for storing PNG files.
using (var archiveStream = File.OpenWrite("Output.zip"))
using (var archive = new ZipArchive(archiveStream, ZipArchiveMode.Create))
{
// Iterate through the PDF pages.
for (int pageIndex = 0; pageIndex < document.Pages.Count; pageIndex++)
{
// Add a white background color to the page.
var page = document.Pages[pageIndex];
var elements = page.Content.Elements;
var background = elements.AddPath(elements.First);
background.AddRectangle(0, 0, page.Size.Width, page.Size.Height);
background.Format.Fill.IsApplied = true;
background.Format.Fill.Color = PdfColor.FromRgb(1, 1, 1);
// Create a ZIP entry for each page.
var entry = archive.CreateEntry($"Page {pageIndex + 1}.png");
// Save each page as a PNG image to the ZIP entry.
using (var imageStream = new MemoryStream())
using (var entryStream = entry.Open())
{
imageOptions.PageNumber = pageIndex;
document.Save(imageStream, imageOptions);
imageStream.Position = 0;
imageStream.CopyTo(entryStream);
}
}
}
}
}
}
Imports GemBox.Pdf
Imports GemBox.Pdf.Content
Imports System.IO
Imports System.IO.Compression
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Load a PDF document.
Using document = PdfDocument.Load("%InputFileName%")
Dim imageOptions = New ImageSaveOptions(ImageSaveFormat.Png)
' Create a ZIP file for storing PNG files.
Using archiveStream = File.OpenWrite("Output.zip")
Using archive = New ZipArchive(archiveStream, ZipArchiveMode.Create)
' Iterate through the PDF pages.
For pageIndex As Integer = 0 To document.Pages.Count - 1
' Add a white background color to the page.
Dim page = document.Pages(pageIndex)
Dim elements = page.Content.Elements
Dim background = elements.AddPath(elements.First)
background.AddRectangle(0, 0, page.Size.Width, page.Size.Height)
background.Format.Fill.IsApplied = True
background.Format.Fill.Color = PdfColor.FromRgb(1, 1, 1)
' Create a ZIP entry for each page.
Dim entry = archive.CreateEntry($"Page {pageIndex + 1}.png")
' Save each page as a PNG image to the ZIP entry.
Using imageStream = New MemoryStream()
Using entryStream = entry.Open()
imageOptions.PageNumber = pageIndex
document.Save(imageStream, imageOptions)
imageStream.Position = 0
imageStream.CopyTo(entryStream)
End Using
End Using
Next
End Using
End Using
End Using
End Sub
End Module
PdfPage.SetCropBox
or PdfPage.SetMediaBox
methods.Convert a PDF file to a multi-frame image
using GemBox.Pdf;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Load a PDF document.
using (var document = PdfDocument.Load("%InputFileName%"))
{
// Max integer value indicates that all document pages should be saved.
var imageOptions = new ImageSaveOptions(ImageSaveFormat.Tiff)
{
PageCount = int.MaxValue
};
// Save the TIFF file with multiple frames, each frame represents a single PDF page.
document.Save("Output.tiff", imageOptions);
}
}
}
Imports GemBox.Pdf
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Load a PDF document.
Using document = PdfDocument.Load("%InputFileName%")
' Max integer value indicates that all document pages should be saved.
Dim imageOptions As New ImageSaveOptions(ImageSaveFormat.Tiff) With
{
.PageCount = Integer.MaxValue
}
' Save the TIFF file with multiple frames, each frame represents a single PDF page.
document.Save("Output.tiff", imageOptions)
End Using
End Sub
End Module