Create PDF files in ASP.NET Core
GemBox.Pdf is a standalone .NET component that's ideal for web applications because of its fast performance and thread safety when working with multiple PdfDocument
objects.
With GemBox.Pdf, you can build web applications that target ASP.NET Core 2.0 and above. The following live demos show how you can create web apps that generate PDF files and download them to your browser.
To use GemBox.Pdf, simply install the GemBox.Pdf package with NuGet or add the following package reference in the project file.
<Project Sdk="Microsoft.NET.Sdk.Web">
<PropertyGroup>
<TargetFramework>net8.0</TargetFramework>
</PropertyGroup>
<ItemGroup>
<PackageReference Include="GemBox.Pdf" Version="*" />
</ItemGroup>
</Project>
Create PDF files in ASP.NET Core MVC
The following example shows how you can create an ASP.NET Core MVC application that generates a PDF file from web forms data and downloads it with a FileStreamResult
.
@model FileModel
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>GemBox.Pdf in ASP.NET Core MVC application</title>
<link rel="icon" href="~/favicon.ico" />
<link rel="stylesheet" href="~/lib/bootstrap/dist/css/bootstrap.min.css" />
<link rel="stylesheet" href="~/css/site.css" />
</head>
<body>
<header>
<nav class="navbar border-bottom box-shadow mb-3">
<div class="container">
<a class="navbar-brand" asp-controller="Home" asp-action="Index">Home</a>
</div>
</nav>
</header>
<div class="container">
<main class="pb-3 row">
<h1 class="display-4 p-3">File generator [Razor View]</h1>
<div class="col-lg-6">
<form asp-action="Download">
<div class="form-group">Header text: <textarea asp-for="Header" class="form-control"></textarea></div>
<div class="form-group">Body text: <textarea asp-for="Body" class="form-control" rows="8"></textarea></div>
<div class="form-group">Footer text: <textarea asp-for="Footer" class="form-control"></textarea></div>
<div class="form-group"><input type="submit" value="Create" class="btn btn-primary" /></div>
</form>
</div>
</main>
</div>
<footer class="footer border-top text-muted">
<div class="container">© GemBox Ltd. — All rights reserved.</div>
</footer>
</body>
</html>
using GemBox.Pdf;
using GemBox.Pdf.Content;
using Microsoft.AspNetCore.Mvc;
using PdfCoreMvc.Models;
using System.ComponentModel.DataAnnotations;
using System.Diagnostics;
using System.IO;
using System.Linq;
namespace PdfCoreMvc.Controllers
{
public class HomeController : Controller
{
// If using the Professional version, put your serial key below.
static HomeController() => ComponentInfo.SetLicense("FREE-LIMITED-KEY");
public IActionResult Index() => this.View(new FileModel());
public FileStreamResult Download(FileModel model)
{
// Create new document.
var document = new PdfDocument();
// Add page.
var page = document.Pages.Add();
// Write text.
using (var formattedText = new PdfFormattedText())
{
// Write header.
formattedText.TextAlignment = PdfTextAlignment.Center;
formattedText.FontSize = 18;
formattedText.MaxTextWidth = 400;
formattedText.Append(model.Header);
page.Content.DrawText(formattedText, new PdfPoint(90, 750));
// Write body.
formattedText.Clear();
formattedText.TextAlignment = PdfTextAlignment.Justify;
formattedText.FontSize = 14;
formattedText.Append(model.Body);
page.Content.DrawText(formattedText, new PdfPoint(90, 400));
// Write footer.
formattedText.Clear();
formattedText.TextAlignment = PdfTextAlignment.Right;
formattedText.FontSize = 10;
formattedText.MaxTextWidth = 100;
formattedText.Append(model.Footer);
page.Content.DrawText(formattedText, new PdfPoint(450, 40));
}
// Save PDF file.
var stream = new MemoryStream();
document.Save(stream);
stream.Position = 0;
// Download file.
return File(stream, "application/pdf", "OutputFromView.pdf");
}
[ResponseCache(Duration = 0, Location = ResponseCacheLocation.None, NoStore = true)]
public IActionResult Error() =>
this.View(new ErrorViewModel() { RequestId = Activity.Current?.Id ?? HttpContext.TraceIdentifier });
}
}
namespace PdfCoreMvc.Models
{
public class FileModel
{
[DisplayFormat(ConvertEmptyStringToNull = false)]
public string Header { get; set; } = "Header text from ASP.NET Core MVC";
[DisplayFormat(ConvertEmptyStringToNull = false)]
public string Body { get; set; } = string.Join(" ",
Enumerable.Repeat("Lorem ipsum dolor sit amet, consectetuer adipiscing elit.", 4));
[DisplayFormat(ConvertEmptyStringToNull = false)]
public string Footer { get; set; } = "Page 1 of 1";
}
}
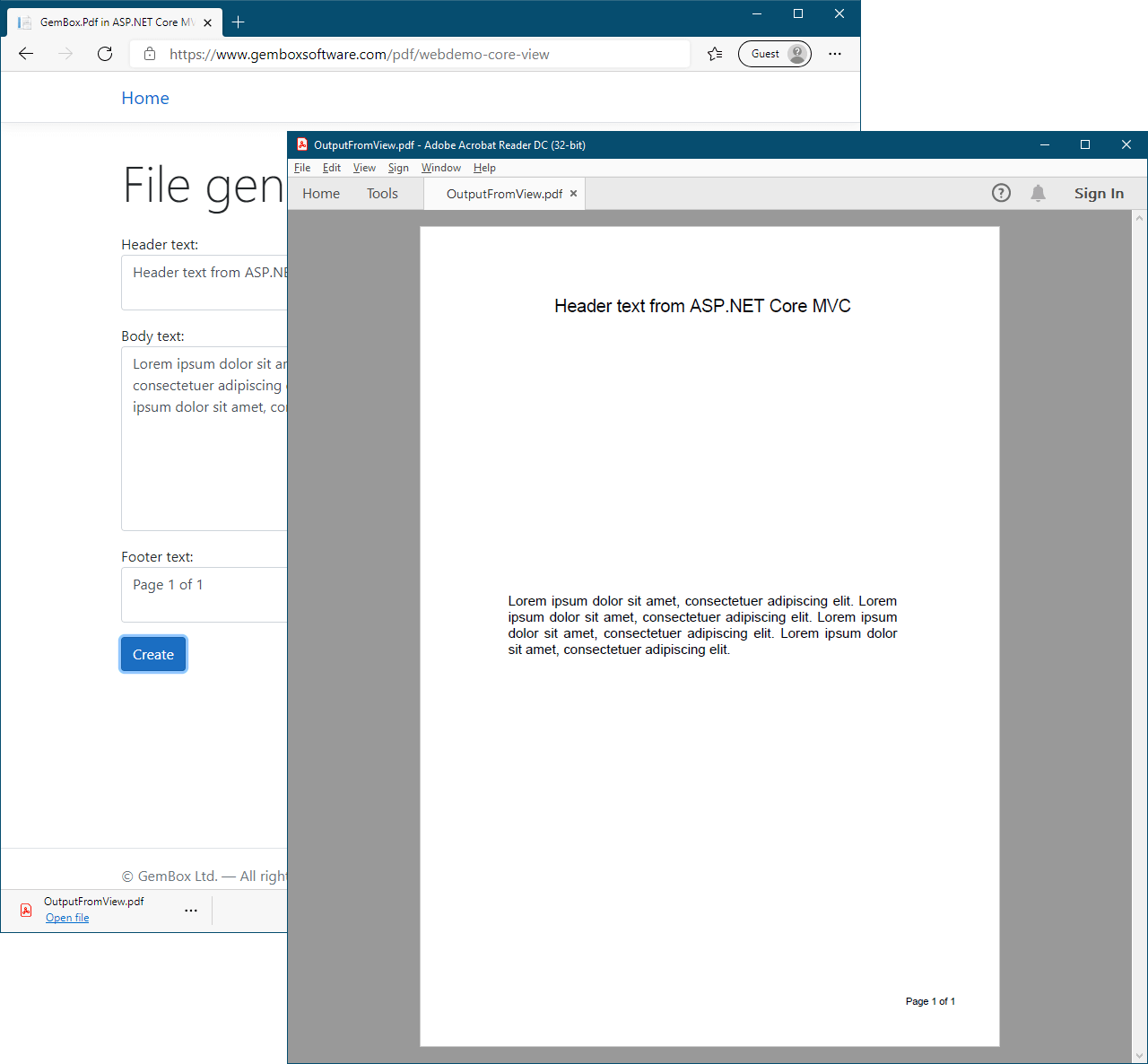
Create PDF files in ASP.NET Core Razor Pages
The following example shows how you can create an ASP.NET Core Razor Pages application that generates a PDF file from web forms data and downloads it with a FileContentResult
.
@page
@model IndexModel
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>GemBox.Pdf in ASP.NET Core Razor Pages application</title>
<link rel="icon" href="~/favicon.ico" />
<link rel="stylesheet" href="~/lib/bootstrap/dist/css/bootstrap.min.css" />
<link rel="stylesheet" href="~/css/site.css" />
</head>
<body>
<header>
<nav class="navbar border-bottom box-shadow mb-3">
<div class="container">
<a class="navbar-brand" asp-page="/Index">Home</a>
</div>
</nav>
</header>
<div class="container">
<main class="pb-3 row">
<h1 class="display-4 p-3">File generator [Razor Page]</h1>
<div class="col-lg-6">
<form method="post">
<div class="form-group">Header text: <textarea asp-for="File.Header" class="form-control"></textarea></div>
<div class="form-group">Body text: <textarea asp-for="File.Body" class="form-control" rows="8"></textarea></div>
<div class="form-group">Footer text: <textarea asp-for="File.Footer" class="form-control"></textarea></div>
<div class="form-group"><input type="submit" value="Create" class="btn btn-primary" /></div>
</form>
</div>
</main>
</div>
<footer class="footer border-top text-muted">
<div class="container">© GemBox Ltd. — All rights reserved.</div>
</footer>
</body>
</html>
using GemBox.Pdf;
using GemBox.Pdf.Content;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.RazorPages;
using PdfCorePages.Models;
using System.ComponentModel.DataAnnotations;
using System.IO;
using System.Linq;
namespace PdfCorePages.Pages
{
public class IndexModel : PageModel
{
[BindProperty]
public new FileModel File { get; set; }
// If using the Professional version, put your serial key below.
static IndexModel() => ComponentInfo.SetLicense("FREE-LIMITED-KEY");
public IndexModel() => this.File = new FileModel();
public void OnGet() { }
public FileContentResult OnPost()
{
// Create new document.
var document = new PdfDocument();
// Add page.
var page = document.Pages.Add();
// Write text.
using (var formattedText = new PdfFormattedText())
{
// Write header.
formattedText.TextAlignment = PdfTextAlignment.Center;
formattedText.FontSize = 18;
formattedText.MaxTextWidth = 400;
formattedText.Append(this.File.Header);
page.Content.DrawText(formattedText, new PdfPoint(90, 750));
// Write body.
formattedText.Clear();
formattedText.TextAlignment = PdfTextAlignment.Justify;
formattedText.FontSize = 14;
formattedText.Append(this.File.Body);
page.Content.DrawText(formattedText, new PdfPoint(90, 400));
// Write footer.
formattedText.Clear();
formattedText.TextAlignment = PdfTextAlignment.Right;
formattedText.FontSize = 10;
formattedText.MaxTextWidth = 100;
formattedText.Append(this.File.Footer);
page.Content.DrawText(formattedText, new PdfPoint(450, 40));
}
// Save PDF file.
var stream = new MemoryStream();
document.Save(stream);
stream.Position = 0;
// Download file.
return File(stream.ToArray(), "application/pdf", "OutputFromPage.pdf");
}
}
}
namespace PdfCorePages.Models
{
public class FileModel
{
[DisplayFormat(ConvertEmptyStringToNull = false)]
public string Header { get; set; } = "Header text from ASP.NET Core Razor Pages";
[DisplayFormat(ConvertEmptyStringToNull = false)]
public string Body { get; set; } = string.Join(" ",
Enumerable.Repeat("Lorem ipsum dolor sit amet, consectetuer adipiscing elit.", 4));
[DisplayFormat(ConvertEmptyStringToNull = false)]
public string Footer { get; set; } = "Page 1 of 1";
}
}
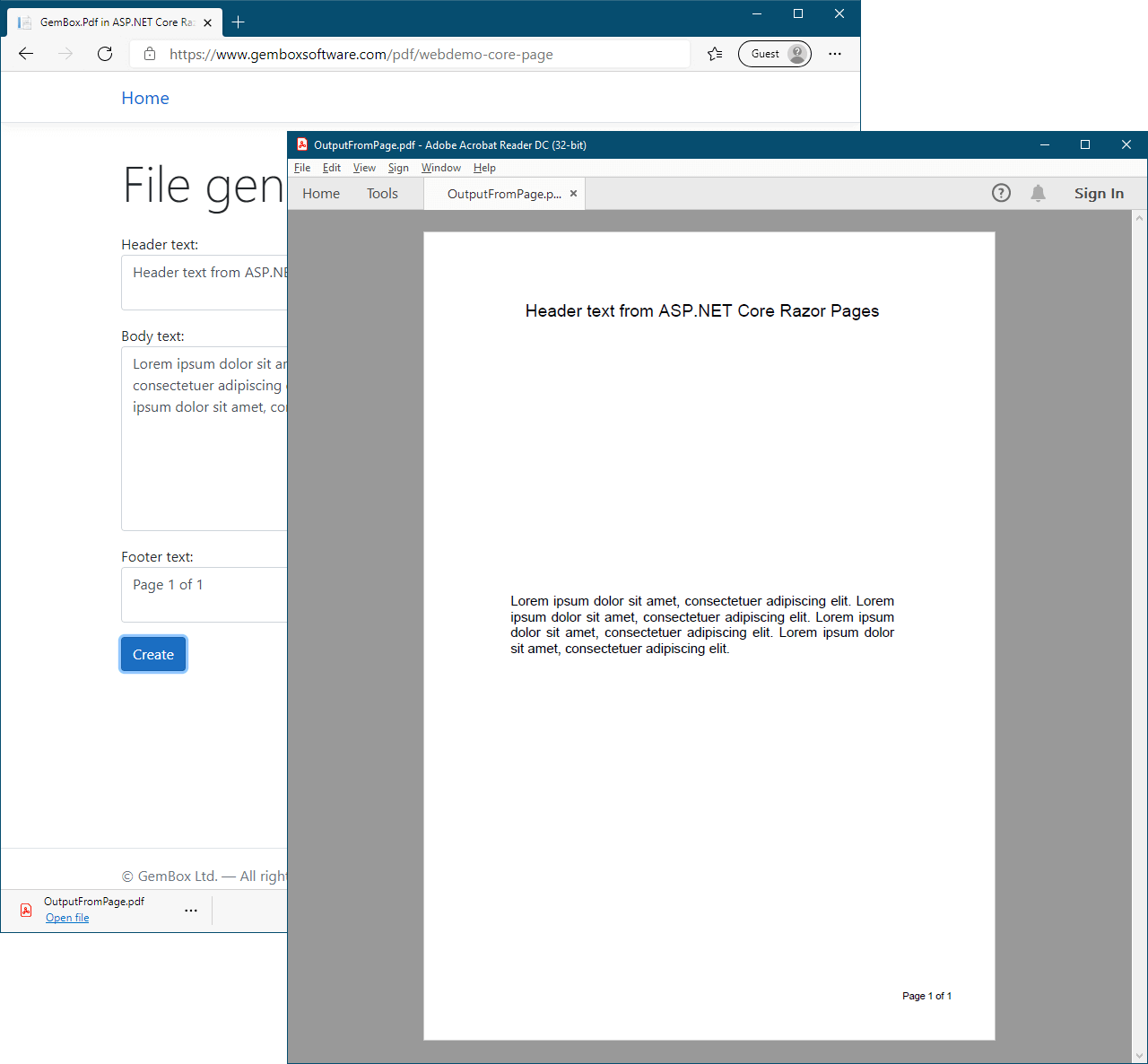
Host and deploy ASP.NET Core
GemBox.Pdf is licensed per individual developer, and the licenses include a royalty-free deployment. You can feel free to build an unlimited number of applications and deploy or distribute them to an unlimited number of services, servers, or end-user machines with no extra cost.
GemBox.Pdf licenses are compatible with SaaS or PaaS solutions, as long as they don't offer similar or competing functionality to our component, or expose our features through an API for use by an unlicensed third party. For more information, please check the EULA.
Create PNG, JPG, or XPS files on Windows
GemBox.Pdf supports saving to XPS and image formats (like PNG and JPG) on applications that target .NET 6.0 or above.
However, these features currently work only on Windows. In other words, besides .NET Core Runtime, you also need a .NET Windows Desktop Runtime installed on the server.
To export an XPS or image file in an ASP.NET Core application, you need to use a Windows-specific TFM in the project file.
<Project Sdk="Microsoft.NET.Sdk.Web">
<PropertyGroup>
<TargetFramework>net8.0-windows</TargetFramework>
</PropertyGroup>
<ItemGroup>
<PackageReference Include="GemBox.Pdf" Version="*" />
</ItemGroup>
</Project>
Also, enable the following compatibility switch with the AppContext
class.
public class Startup
{
public Startup(IConfiguration configuration)
{
Configuration = configuration;
// Add compatibility switch.
AppContext.SetSwitch("Switch.System.Windows.Media.ShouldRenderEvenWhenNoDisplayDevicesAreAvailable", true);
}
public IConfiguration Configuration { get; }
// This method gets called by the runtime. Use this method to add services to the container.
public void ConfigureServices(IServiceCollection services)
{
// ...
}
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
// ...
}
}
Or you can enable that compatibility switch by adding the following runtimeconfig.template.json file to your project.
{
"configProperties": {
"Switch.System.Windows.Media.ShouldRenderEvenWhenNoDisplayDevicesAreAvailable": true
}
}
Limitations on Linux or macOS
You can use the full functionality of GemBox.Pdf on Unix systems, but with the following exceptions:
- Printing documents.
- Saving documents to XPS or image formats.
- Calling
ConvertToImageSource
andConvertToXpsDocument
methods.
These features currently have WPF dependencies which means they require a .NET Windows Desktop Runtime. However, we do have plans for providing cross-platform support for them in future releases.