Rotate and flip images in MAUI
GemBox.Imaging is a standalone .NET component with cross-platform support for processing images. With the help of MAUI, you can use it on platforms like Android, iOS, Linux, and macOS for reading, writing, editing, and converting PNG, JPEG, GIF, and TIFF files.
The following example shows how to rotate an image at 180 degrees and flip it vertically in a MAUI mobile application using the GemBox.Imaging .NET library.
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="ImagingMaui.MainPage">
<ScrollView>
<VerticalStackLayout
Spacing="25"
Padding="30,0"
VerticalOptions="Center">
<Label
Text="GemBox.Imaging Example"
FontSize="Large"
HorizontalOptions="Center" />
<Picker
x:Name="rotateFlipPicker"
Title="Rotation/Flipping"
ItemsSource="{Binding RotateFlipOptions}"
ItemDisplayBinding="{Binding Text}"
HorizontalOptions="Center" />
<Image
x:Name="sourceImage"
Source=""
HeightRequest="160"
WidthRequest="160"
HorizontalOptions="Center" />
<Button
x:Name="button"
Text="TRANSFORM IMAGE"
Clicked="Button_Clicked"
HorizontalOptions="Center" />
</VerticalStackLayout>
</ScrollView>
</ContentPage>
using GemBox.Imaging;
using ImagingMaui.Models;
using System;
using System.IO;
using System.Threading.Tasks;
namespace ImagingMaui
{
public partial class MainPage : ContentPage
{
private byte[] imageData;
public MainPage()
{
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
InitializeComponent();
BindingContext = new RotateFlipViewModel();
}
protected override void OnAppearing()
{
base.OnAppearing();
if (imageData == null)
GetDefaultImageAsync().Wait();
this.sourceImage.Source = ImageSource.FromStream(() => new MemoryStream(this.imageData));
}
private async Task GetDefaultImageAsync()
{
using var ms = new MemoryStream();
using (var stream = await FileSystem.OpenAppPackageFileAsync("fragonard_reader.jpg"))
await stream.CopyToAsync(ms);
this.imageData = ms.ToArray();
}
private string RotateImage(Stream stream)
{
var documentPath = Environment.GetFolderPath(Environment.SpecialFolder.MyDocuments);
var selectedRotationFlipping = (RotateFlipOption)rotateFlipPicker.SelectedItem;
var destinationFilePath = Path.Combine(documentPath, $"{selectedRotationFlipping.Text}.jpg");
using (var image = GemBox.Imaging.Image.Load(stream))
{
image.RotateFlip(selectedRotationFlipping.Type);
image.Save(destinationFilePath);
}
return destinationFilePath;
}
private async void Button_Clicked(object sender, EventArgs e)
{
this.button.IsEnabled = false;
try
{
var filePath = await Task.Run(() => RotateImage(new MemoryStream(this.imageData)));
await Launcher.OpenAsync(new OpenFileRequest(Path.GetFileName(filePath), new ReadOnlyFile(filePath)));
}
catch (Exception ex)
{
await DisplayAlert("Error", ex.Message, "Close");
}
this.button.IsEnabled = true;
}
}
}
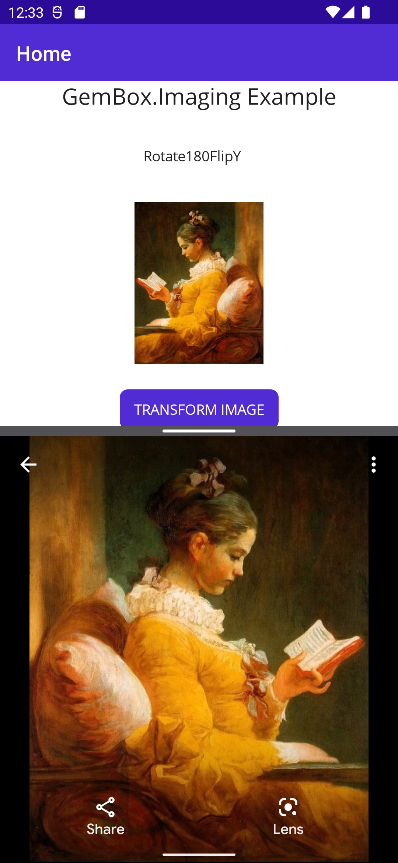