Read image files in a Docker container
GemBox.Imaging is a cross-platform imaging library for .NET so you can use it inside docker containers that are running .NET Docker images. To run this example locally, you must first install Docker Desktop.
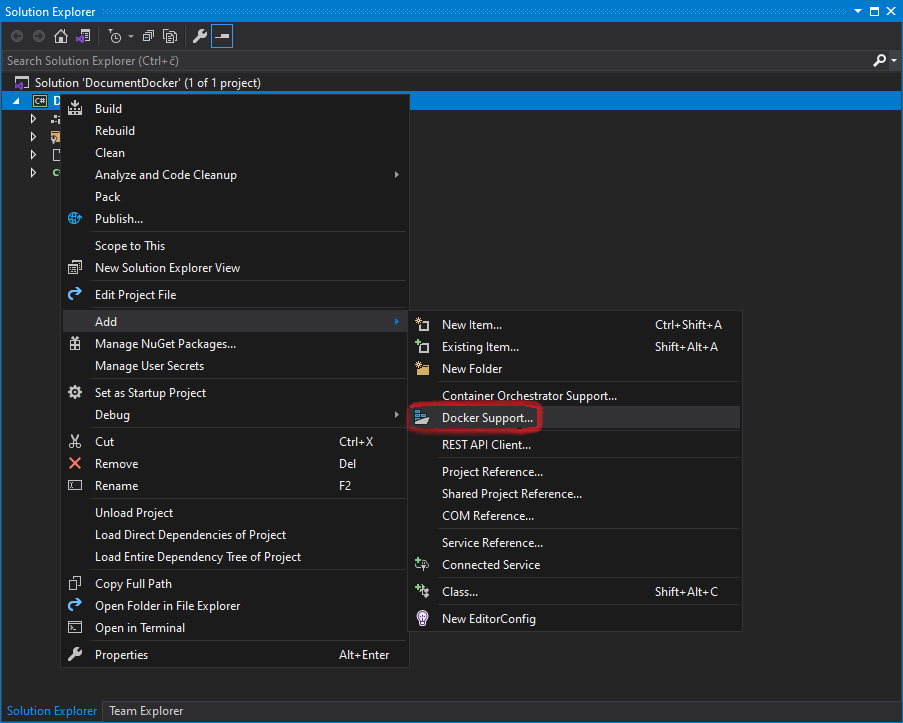
The following code presents a project file for the .NET Core application with added Docker support.
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<OutputType>Exe</OutputType>
<TargetFramework>net8.0</TargetFramework>
<DockerDefaultTargetOS>Linux</DockerDefaultTargetOS>
<DockerfileContext>.</DockerfileContext>
</PropertyGroup>
<ItemGroup>
<PackageReference Include="GemBox.Imaging" Version="*" />
<PackageReference Include="SkiaSharp.NativeAssets.Linux" Version="*" />
</ItemGroup>
</Project>
To configure or customize a Docker image, you'll need to edit the Dockerfile.
The example below shows how you can read image files from Docker containers and configure Docker images with Dockerfile.
FROM mcr.microsoft.com/dotnet/runtime:8.0 AS base
WORKDIR /app
FROM mcr.microsoft.com/dotnet/sdk:8.0 AS build
WORKDIR /src
COPY ["ImagingDocker.csproj", ""]
RUN dotnet restore "./ImagingDocker.csproj"
COPY . .
WORKDIR "/src/."
RUN dotnet build "ImagingDocker.csproj" -c Release -o /app/build
FROM build AS publish
RUN dotnet publish "ImagingDocker.csproj" -c Release -o /app/publish /p:UseAppHost=false
FROM base AS final
WORKDIR /app
COPY --from=publish /app/publish .
ENTRYPOINT ["dotnet", "ImagingDocker.dll"]
using GemBox.Imaging;
using System;
class Program
{
static void Main()
{
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
using (var image = Image.Load("FragonardReader.jpg"))
{
// Display image information.
Console.WriteLine($"Image size: {image.Width}x{image.Height}");
}
}
}
Imports GemBox.Imaging
Imports System
Module Program
Sub Main()
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Using image As Image = Image.Load("FragonardReader.jpg")
' Display image information.
Console.WriteLine($"Image size: {image.Width}x{image.Height}")
End Using
End Sub
End Module