Crop and resize images in ASP.NET Core
GemBox.Imaging is a standalone .NET component that's ideal for web applications because of its cross-platform support and fast performance when working with multiple Image
objects.
With GemBox.Imaging you can build web applications that target ASP.NET Core 2.0 and above. The following live demos show how to create web apps that generate Word and PDF files and download them to your browser.
To use GemBox.Imaging, simply install the GemBox.Imaging package with NuGet or add the following package reference in the project file.
<Project Sdk="Microsoft.NET.Sdk.Web">
<PropertyGroup>
<TargetFramework>net8.0</TargetFramework>
</PropertyGroup>
<ItemGroup>
<PackageReference Include="GemBox.Imaging" Version="*" />
</ItemGroup>
</Project>
Crop image files in ASP.NET Core MVC
The following example shows how you can create an ASP.NET Core MVC application that:
- Imports data from a web form into an Image object.
- Crops the image with the given parameters.
- Downloads the cropped image file with a
FileStreamResult
.
@using ImagingCoreMvc.Models;
@model CropImageModel
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>GemBox.Imaging in ASP.NET Core MVC application</title>
<link rel="icon" href="~/favicon.ico" />
<link rel="stylesheet" href="~/lib/bootstrap/dist/css/bootstrap.min.css" />
<link rel="stylesheet" href="~/css/site.css" />
</head>
<body>
<header>
<nav class="navbar border-bottom box-shadow mb-3">
<div class="container">
<a class="navbar-brand" asp-controller="Home" asp-action="Index">Home</a>
</div>
</nav>
</header>
<div class="container">
<main class="pb-3 row">
<h1 class="display-4 p-3">Image cropper [Razor View]</h1>
<div class="col-lg-6">
<form asp-action="Download" enctype="multipart/form-data">
<div class="form-group">Image: <input asp-for="Image" class="form-control" /></div>
<div class="form-group">X position: <input asp-for="PosX" class="form-control" /></div>
<div class="form-group">Y position: <input asp-for="PosY" class="form-control" /></div>
<div class="form-group">Width: <input asp-for="Width" class="form-control" /></div>
<div class="form-group">Height: <input asp-for="Height" class="form-control" /></div>
<div class="form-group"><input type="submit" value="Crop" class="btn btn-primary" /></div>
</form>
</div>
</main>
</div>
<footer class="footer border-top text-muted">
<div class="container">© GemBox Ltd. — All rights reserved.</div>
</footer>
</body>
</html>
using GemBox.Imaging;
using ImagingCoreMvc.Models;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using System;
using System.Collections.Generic;
using System.Diagnostics;
using System.IO;
namespace ImagingCoreMvc.Controllers
{
public class HomeController : Controller
{
// If using the Professional version, put your serial key below.
static HomeController() => ComponentInfo.SetLicense("FREE-LIMITED-KEY");
public IActionResult Index() => this.View(new CropImageModel());
public FileStreamResult Download(CropImageModel model)
{
// Load the image.
using var imageStream = model.Image.OpenReadStream();
using var image = Image.Load(imageStream);
// Execute crop process.
image.Crop(model.PosX, model.PosY, model.Width, model.Height);
// Save image in specified file format.
var stream = new MemoryStream();
image.Save(stream, model.FileFormat);
stream.Position = 0;
// Download file.
return File(stream, model.ContentType, $"Output{model.Extension}");
}
[ResponseCache(Duration = 0, Location = ResponseCacheLocation.None, NoStore = true)]
public IActionResult Error() =>
this.View(new ErrorViewModel() { RequestId = Activity.Current?.Id ?? HttpContext.TraceIdentifier });
}
}
namespace ImagingCoreMvc.Models
{
public class CropImageModel
{
public IFormFile Image { get; set; }
public int PosX { get; set; }
public int PosY { get; set; }
public int Width { get; set; }
public int Height { get; set; }
public string Extension => Path.GetExtension(Image.FileName).ToLowerInvariant();
public ImageFileFormat FileFormat => ImageMappingDictionary[this.Extension].FileFormat;
public string ContentType => ImageMappingDictionary[this.Extension].ContentType;
public static IDictionary<string, (ImageFileFormat FileFormat, string ContentType)> ImageMappingDictionary =>
new Dictionary<string, (ImageFileFormat, string)>(StringComparer.OrdinalIgnoreCase)
{
[".png"] = (ImageFileFormat.Png, "image/png"),
[".jpg"] = (ImageFileFormat.Jpeg, "image/jpeg"),
[".jpeg"] = (ImageFileFormat.Jpeg, "image/jpeg"),
[".gif"] = (ImageFileFormat.Gif, "image/gif"),
[".tif"] = (ImageFileFormat.Tiff, "image/tiff"),
[".tiff"] = (ImageFileFormat.Tiff, "image/tiff")
};
}
}
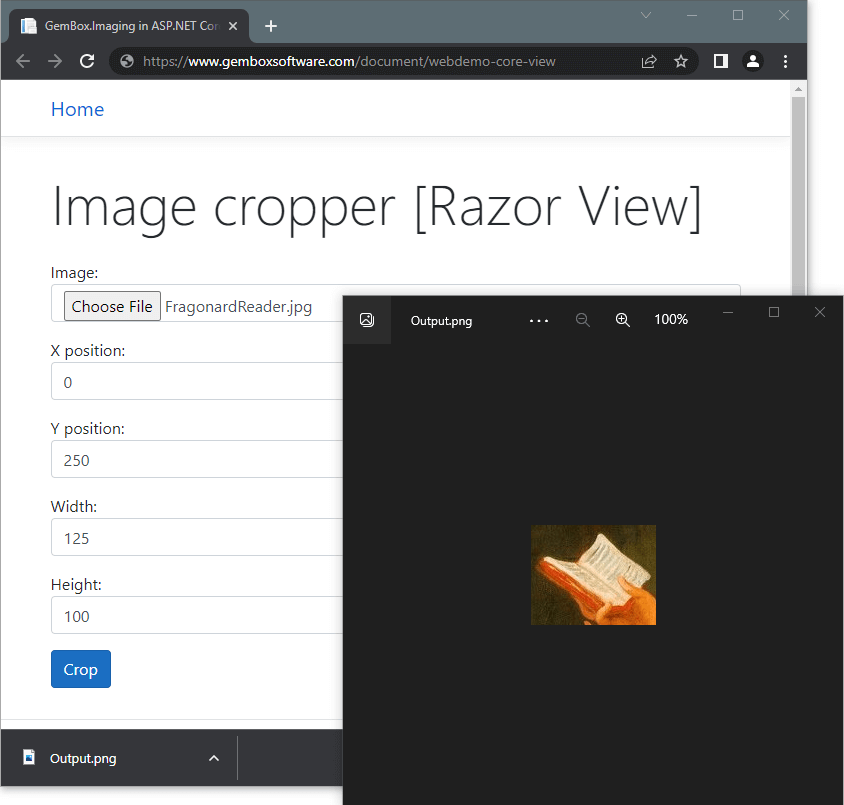
Crop image files in ASP.NET Core Razor Pages
The following example shows how you can create an ASP.NET Core Razor Pages application that:
- Imports data from a web form into an Image object.
- Crops the image with the given parameters.
- Downloads the cropped image file with a
FileStreamResult
.
@page
@model IndexModel
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>GemBox.Imaging in ASP.NET Core Razor Pages application</title>
<link rel="icon" href="~/favicon.ico" />
<link rel="stylesheet" href="~/lib/bootstrap/dist/css/bootstrap.min.css" />
<link rel="stylesheet" href="~/css/site.css" />
</head>
<body>
<header>
<nav class="navbar border-bottom box-shadow mb-3">
<div class="container">
<a class="navbar-brand" asp-controller="Home" asp-action="Index">Home</a>
</div>
</nav>
</header>
<div class="container">
<main class="pb-3 row">
<h1 class="display-4 p-3">Image cropper [Razor Page]</h1>
<div class="col-lg-6">
<form method="post" enctype="multipart/form-data">
<div class="form-group">Image: <input asp-for="Model.Image" class="form-control" /></div>
<div class="form-group">X position: <input asp-for="Model.PosX" class="form-control" /></div>
<div class="form-group">Y position: <input asp-for="Model.PosY" class="form-control" /></div>
<div class="form-group">Width: <input asp-for="Model.Width" class="form-control" /></div>
<div class="form-group">Height: <input asp-for="Model.Height" class="form-control" /></div>
<div class="form-group"><input type="submit" value="Crop" class="btn btn-primary" /></div>
</form>
</div>
</main>
</div>
<footer class="footer border-top text-muted">
<div class="container">© GemBox Ltd. — All rights reserved.</div>
</footer>
</body>
</html>
using GemBox.Imaging;
using ImagingCorePages.Models;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.RazorPages;
using System;
using System.Collections.Generic;
using System.IO;
namespace ImagingCorePages.Pages
{
public class IndexModel : PageModel
{
[BindProperty]
public CropImageModel Model { get; set; }
// If using the Professional version, put your serial key below.
static IndexModel() => ComponentInfo.SetLicense("FREE-LIMITED-KEY");
public IndexModel() => this.Model = new CropImageModel();
public void OnGet() { }
public FileStreamResult OnPost()
{
// Load the image.
using var imageStream = this.Model.Image.OpenReadStream();
using var image = Image.Load(imageStream);
// Execute crop process.
image.Crop(this.Model.PosX, this.Model.PosY, this.Model.Width, this.Model.Height);
// Save image in specified file format.
var stream = new MemoryStream();
image.Save(stream, this.Model.FileFormat);
stream.Position = 0;
// Download file.
return File(stream, this.Model.ContentType, $"Output{this.Model.Extension}");
}
}
}
namespace ImagingCorePages.Models
{
public class CropImageModel
{
public IFormFile Image { get; set; }
public int PosX { get; set; }
public int PosY { get; set; }
public int Width { get; set; }
public int Height { get; set; }
public string Extension => Path.GetExtension(Image.FileName).ToLowerInvariant();
public ImageFileFormat FileFormat => ImageMappingDictionary[this.Extension].FileFormat;
public string ContentType => ImageMappingDictionary[this.Extension].ContentType;
public static IDictionary<string, (ImageFileFormat FileFormat, string ContentType)> ImageMappingDictionary =>
new Dictionary<string, (ImageFileFormat, string)>(StringComparer.OrdinalIgnoreCase)
{
[".png"] = (ImageFileFormat.Png, "image/png"),
[".jpg"] = (ImageFileFormat.Jpeg, "image/jpeg"),
[".jpeg"] = (ImageFileFormat.Jpeg, "image/jpeg"),
[".gif"] = (ImageFileFormat.Gif, "image/gif"),
[".tif"] = (ImageFileFormat.Tiff, "image/tiff"),
[".tiff"] = (ImageFileFormat.Tiff, "image/tiff")
};
}
}
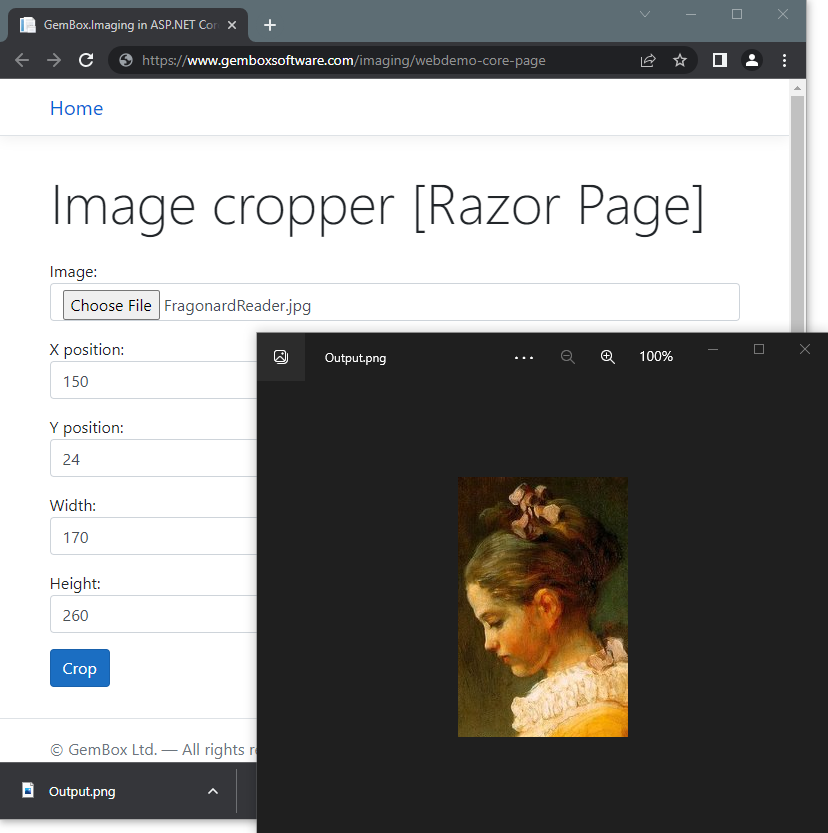
Resize Image Files in ASP.NET Core MVC
Experience the simplicity of resizing image files in an ASP.NET Core MVC application. This example guides you through:
- Importing data from a web form into an Image object.
- Resizing the image with specified parameters.
- Downloading the resized image file with a FileStreamResult.
@using ImagingCoreMvc.Models;
@model ResizeImageModel
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>GemBox.Imaging in ASP.NET Core MVC application</title>
<link rel="icon" href="~/favicon.ico" />
<link rel="stylesheet" href="~/lib/bootstrap/dist/css/bootstrap.min.css" />
<link rel="stylesheet" href="~/css/site.css" />
</head>
<body>
<header>
<nav class="navbar border-bottom box-shadow mb-3">
<div class="container">
<a class="navbar-brand" asp-controller="Home" asp-action="Index">Home</a>
</div>
</nav>
</header>
<div class="container">
<main class="pb-3 row">
<h1 class="display-4 p-3">Image resizer [Razor View]</h1>
<div class="col-lg-6">
<form asp-action="Download" enctype="multipart/form-data">
<div class="form-group">Image: <input asp-for="Image" class="form-control" /></div>
<div class="form-group">Width: <input asp-for="Width" class="form-control" /></div>
<div class="form-group">Height: <input asp-for="Height" class="form-control" /></div>
<div class="form-group"><input type="submit" value="Resize" class="btn btn-primary" /></div>
</form>
</div>
</main>
</div>
<footer class="footer border-top text-muted">
<div class="container">© GemBox Ltd. — All rights reserved.</div>
</footer>
</body>
</html>
using GemBox.Imaging;
using ImagingCoreMvc.Models;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using System;
using System.Collections.Generic;
using System.Diagnostics;
using System.IO;
namespace ImagingCoreMvc.Controllers
{
public class HomeController : Controller
{
// If using the Professional version, put your serial key below.
static HomeController() => ComponentInfo.SetLicense("FREE-LIMITED-KEY");
public IActionResult Index() => this.View(new ResizeImageModel());
public FileStreamResult Download(ResizeImageModel model)
{
// Load the image.
using var imageStream = model.Image.OpenReadStream();
using var image = Image.Load(imageStream);
// Execute resize process.
image.Resize(model.Width, model.Height);
// Save image in specified file format.
var stream = new MemoryStream();
image.Save(stream, model.FileFormat);
stream.Position = 0;
// Download file.
return File(stream, model.ContentType, $"Resized{model.Extension}");
}
[ResponseCache(Duration = 0, Location = ResponseCacheLocation.None, NoStore = true)]
public IActionResult Error() =>
this.View(new ErrorViewModel() { RequestId = Activity.Current?.Id ?? HttpContext.TraceIdentifier });
}
}
namespace ImagingCoreMvc.Models
{
public class ResizeImageModel
{
public IFormFile Image { get; set; }
public int? Width { get; set; }
public int? Height { get; set; }
public string Extension => Path.GetExtension(Image.FileName).ToLowerInvariant();
public ImageFileFormat FileFormat => ImageMappingDictionary[this.Extension].FileFormat;
public string ContentType => ImageMappingDictionary[this.Extension].ContentType;
public static IDictionary<string, (ImageFileFormat FileFormat, string ContentType)> ImageMappingDictionary =>
new Dictionary<string, (ImageFileFormat, string)>(StringComparer.OrdinalIgnoreCase)
{
[".png"] = (ImageFileFormat.Png, "image/png"),
[".jpg"] = (ImageFileFormat.Jpeg, "image/jpeg"),
[".jpeg"] = (ImageFileFormat.Jpeg, "image/jpeg"),
[".gif"] = (ImageFileFormat.Gif, "image/gif"),
[".tif"] = (ImageFileFormat.Tiff, "image/tiff"),
[".tiff"] = (ImageFileFormat.Tiff, "image/tiff")
};
}
}
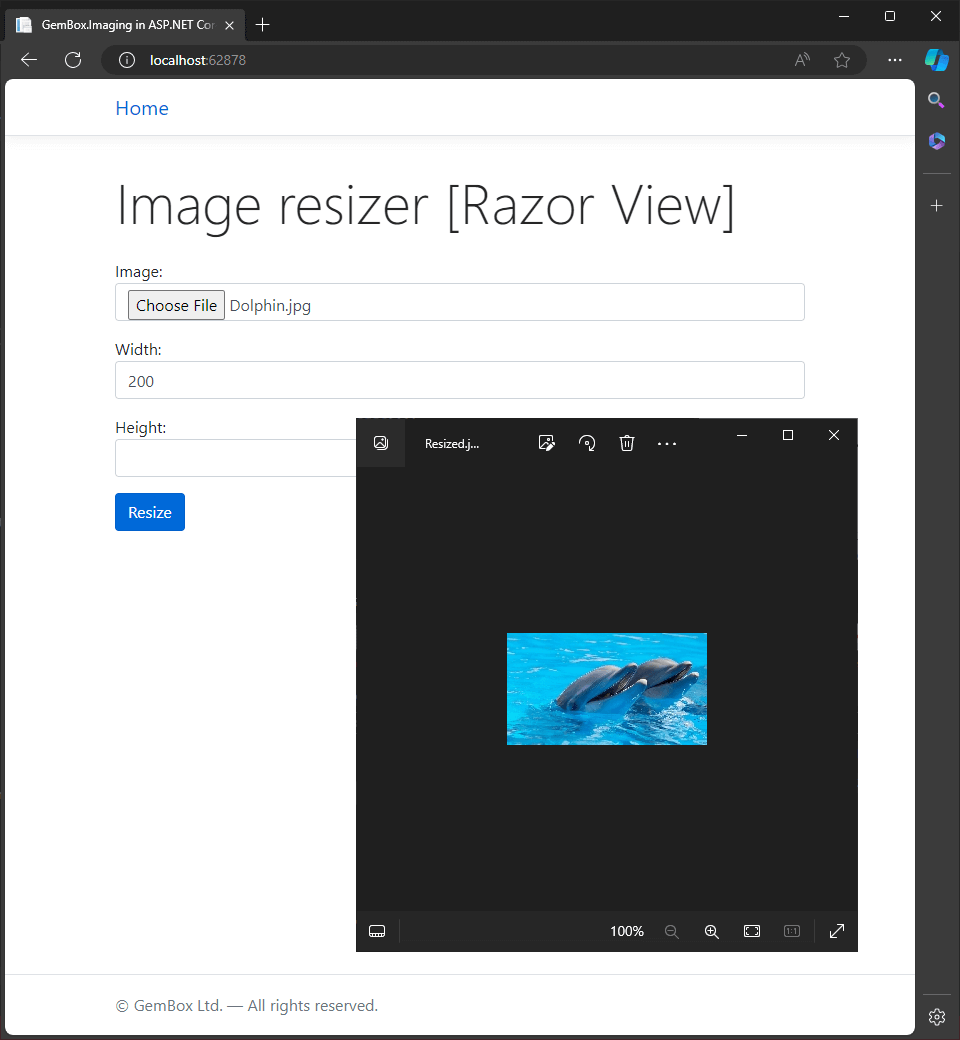
Resize Image Files in ASP.NET Core Razor Pages
Explore the straightforward process of resizing image files in an ASP.NET Core Razor Pages application. This example outlines:
- Importing data from a web form into an Image object.
- Resizing the image with specified parameters.
- Downloading the resized image file with a FileStreamResult.
@page
@model IndexModel
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>GemBox.Imaging in ASP.NET Core MVC application</title>
<link rel="icon" href="~/favicon.ico" />
<link rel="stylesheet" href="~/lib/bootstrap/dist/css/bootstrap.min.css" />
<link rel="stylesheet" href="~/css/site.css" />
</head>
<body>
<header>
<nav class="navbar border-bottom box-shadow mb-3">
<div class="container">
<a class="navbar-brand" asp-controller="Home" asp-action="Index">Home</a>
</div>
</nav>
</header>
<div class="container">
<main class="pb-3 row">
<h1 class="display-4 p-3">Image resizer [Razor Page]</h1>
<div class="col-lg-6">
<form method="post" enctype="multipart/form-data">
<div class="form-group">Image: <input asp-for="Model.Image" class="form-control" /></div>
<div class="form-group">Width: <input asp-for="Model.Width" class="form-control" /></div>
<div class="form-group">Height: <input asp-for="Model.Height" class="form-control" /></div>
<div class="form-group"><input type="submit" value="Resize" class="btn btn-primary" /></div>
</form>
</div>
</main>
</div>
<footer class="footer border-top text-muted">
<div class="container">© GemBox Ltd. — All rights reserved.</div>
</footer>
</body>
</html>
using GemBox.Imaging;
using ImagingCorePages.Models;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.RazorPages;
using System;
using System.Collections.Generic;
using System.IO;
namespace ImagingCorePages.Pages
{
public class IndexModel : PageModel
{
[BindProperty]
public ResizeImageModel Model { get; set; }
// If using the Professional version, put your serial key below.
static IndexModel() => ComponentInfo.SetLicense("FREE-LIMITED-KEY");
public IndexModel() => this.Model = new ResizeImageModel();
public void OnGet() { }
public FileStreamResult OnPost()
{
// Load the image.
using var imageStream = this.Model.Image.OpenReadStream();
using var image = Image.Load(imageStream);
// Execute resize process.
image.Resize(this.Model.Width, this.Model.Height);
// Save image in specified file format.
var stream = new MemoryStream();
image.Save(stream, this.Model.FileFormat);
stream.Position = 0;
// Download file.
return File(stream, this.Model.ContentType, $"Resized{this.Model.Extension}");
}
}
}
namespace ImagingCorePages.Models
{
public class ResizeImageModel
{
public IFormFile Image { get; set; }
public int? Width { get; set; }
public int? Height { get; set; }
public string Extension => Path.GetExtension(Image.FileName).ToLowerInvariant();
public ImageFileFormat FileFormat => ImageMappingDictionary[this.Extension].FileFormat;
public string ContentType => ImageMappingDictionary[this.Extension].ContentType;
public static IDictionary<string, (ImageFileFormat FileFormat, string ContentType)> ImageMappingDictionary =>
new Dictionary<string, (ImageFileFormat, string)>(StringComparer.OrdinalIgnoreCase)
{
[".png"] = (ImageFileFormat.Png, "image/png"),
[".jpg"] = (ImageFileFormat.Jpeg, "image/jpeg"),
[".jpeg"] = (ImageFileFormat.Jpeg, "image/jpeg"),
[".gif"] = (ImageFileFormat.Gif, "image/gif"),
[".tif"] = (ImageFileFormat.Tiff, "image/tiff"),
[".tiff"] = (ImageFileFormat.Tiff, "image/tiff")
};
}
}
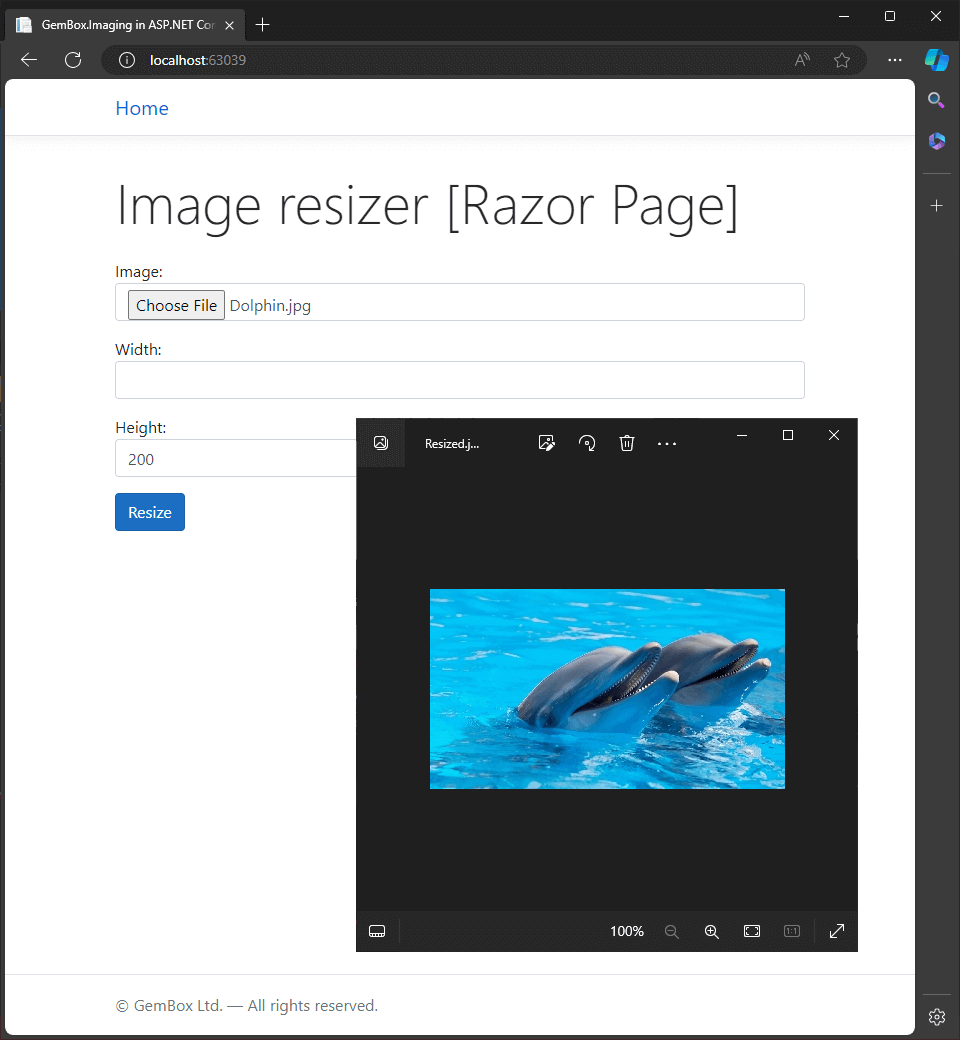
Host and deploy ASP.NET Core
GemBox.Imaging is licensed per individual developer, and the licenses include a royalty-free deployment. You can feel free to build an unlimited number of applications and deploy or distribute them to an unlimited number of services, servers, or end-user machines with no extra cost.
GemBox.Imaging licenses are compatible with SaaS or PaaS solutions as long as they don't offer functionality similar to or competing with our component or expose our features through an API for use by an unlicensed third party. For more information, please check the (EULA).