Send Email Messages Using Exchange Server and EWS protocol
The example below shows how you can use the GemBox.Email component to connect to the Exchange Server and send an email from your console application by using C# or VB.NET code.
using GemBox.Email;
using GemBox.Email.Exchange;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Create a new email message.
MailMessage message = new MailMessage(
new MailAddress("sender@example.com", "Sender"),
new MailAddress("first.receiver@example.com", "First receiver"),
new MailAddress("second.receiver@example.com", "Second receiver"));
// Add additional receivers.
message.Cc.Add(
new MailAddress("third.receiver@example.com", "Third receiver"),
new MailAddress("fourth.receiver@example.com", "Fourth receiver"));
// Add subject and body.
message.Subject = "Send Email in C# and VB.NET";
message.BodyText = "Hi 👋,\n" +
"This message was created and sent with GemBox.Email.\n" +
"Read more about it on https://www.gemboxsoftware.com/email";
// Create a new Exchange client and send an email message.
var exchangeClient = new ExchangeClient("<HOST> (e.g. https://outlook.office365.com/EWS/Exchange.asmx)");
exchangeClient.Authenticate("<USERNAME>", "<PASSWORD>");
exchangeClient.SendMessage(message);
}
}
Imports GemBox.Email
Imports GemBox.Email.Exchange
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Create a new email message.
Dim message As New MailMessage(
New MailAddress("sender@example.com", "Sender"),
New MailAddress("first.receiver@example.com", "First receiver"),
New MailAddress("second.receiver@example.com", "Second receiver"))
' Add additional receivers.
message.Cc.Add(
New MailAddress("third.receiver@example.com", "Third receiver"),
New MailAddress("fourth.receiver@example.com", "Fourth receiver"))
' Add subject and body.
message.Subject = "Send Email in C# and VB.NET"
message.BodyText = "Hi 👋," & vbLf &
"This message was created and sent with GemBox.Email." & vbLf &
"Read more about it on https://www.gemboxsoftware.com/email"
' Create a new Exchange client and send an email message.
Dim exchangeClient = New ExchangeClient("<HOST> (e.g. https://outlook.office365.com/EWS/Exchange.asmx)")
exchangeClient.Authenticate("<USERNAME>", "<PASSWORD>")
exchangeClient.SendMessage(message)
End Sub
End Module
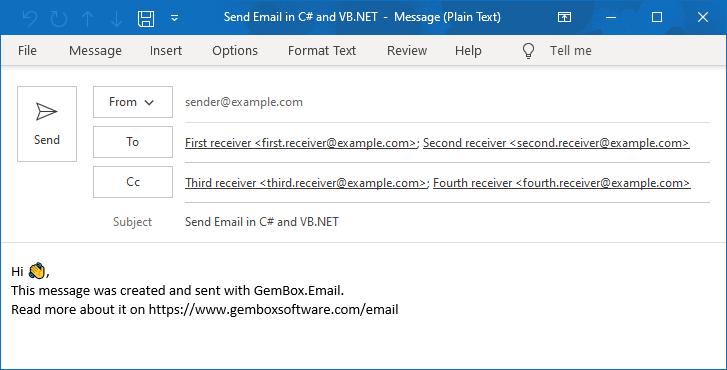
GemBox.Email supports communication with the Microsoft Exchange Server, when using the Exchange Web Service (EWS) protocol. The following example shows how you can use GemBox.Email to send email with attachments in EWS.Send an email with an attachment in EWS
using GemBox.Email;
using GemBox.Email.Exchange;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Create new email message.
MailMessage message = new MailMessage("sender@example.com", "receiver@example.com");
// Add subject.
message.Subject = "Send HTML Email with Image and Attachment";
// Add HTML body with CID embedded image.
string cid = "image001@gembox.com";
message.BodyHtml = "<html><body>" +
"<p>Hi 👋,</p>" +
"<p>This message was created and sent with:</p>" +
"<p><img src='cid:" + cid + "'/></p>" +
"<p>Read more about it on <a href='https://www.gemboxsoftware.com/email'>GemBox.Email Overview</a> page.</p>" +
"</body></html>";
// Add image as an inline attachment.
message.Attachments.Add(new Attachment("%#GemBoxEmailLogo.png%") { ContentId = cid });
// Add PDF as an attachment.
message.Attachments.Add(new Attachment("%#GemBoxSampleFile.pdf%"));
// Create a new Exchange client and send the email message.
var exchangeClient = new ExchangeClient("<HOST> (e.g. https://outlook.office365.com/EWS/Exchange.asmx)");
exchangeClient.Authenticate("<USERNAME>", "<PASSWORD>");
exchangeClient.SendMessage(message);
}
}
Imports GemBox.Email
Imports GemBox.Email.Exchange
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Create new email message.
Dim message As New MailMessage("sender@example.com", "receiver@example.com")
' Add subject.
message.Subject = "Send HTML Email with Image and Attachment"
' Add HTML body with CID embedded image.
Dim cid As String = "image001@gembox.com"
message.BodyHtml = "<html><body>" &
"<p>Hi 👋,</p>" &
"<p>This message was created and sent with:</p>" &
"<p><img src='cid:" & cid & "'/></p>" &
"<p>Read more about it on <a href='https://www.gemboxsoftware.com/email'>GemBox.Email Overview</a> page.</p>" &
"</body></html>"
' Add image as inline attachment.
message.Attachments.Add(New Attachment("%#GemBoxEmailLogo.png%") With {.ContentId = cid})
' Add file as attachment.
message.Attachments.Add(New Attachment("%#GemBoxSampleFile.pdf%"))
' Create a new Exchange client and send the email message.
Dim exchangeClient = New ExchangeClient("<HOST> (e.g. https://outlook.office365.com/EWS/Exchange.asmx)")
exchangeClient.Authenticate("<USERNAME>", "<PASSWORD>")
exchangeClient.SendMessage(message)
End Sub
End Module