Reply and Forward Email in C# and VB.NET
To write a reply message, your email needs to contain two headers: In-Reply-To
and References
. These headers, also known as identification fields, are defined in RFC 2822.
Optionally, you can prefix the email's subject with "Re:" and include the original message in the reply email's body.
The following example shows how you can search for a specific message in the INBOX folder using ImapClient
. A reply email is created in C# and VB.NET with the required reply headers and a HTML body that includes the original message text, after which the message is sent using SmtpClient
.
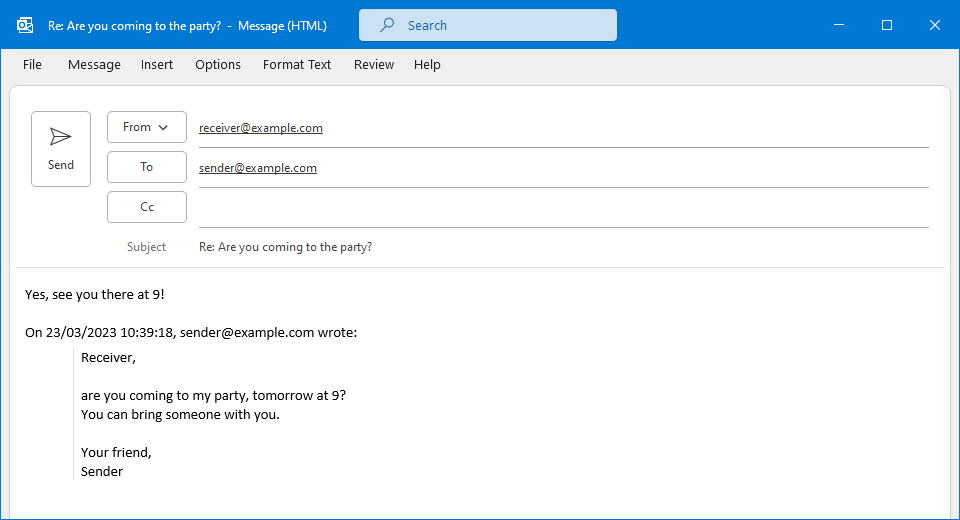
using System.Linq;
using GemBox.Email;
using GemBox.Email.Imap;
using GemBox.Email.Mime;
using GemBox.Email.Smtp;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Retrieve original message.
MailMessage originalMessage;
using (ImapClient imap = new ImapClient("<ADDRESS> (e.g. imap.gmail.com)"))
{
imap.Connect();
imap.Authenticate("<USERNAME>", "<PASSWORD>");
imap.SelectInbox();
string searchQuery = @"SUBJECT ""Are you coming to the party?""";
originalMessage = imap.GetMessage(imap.SearchMessageNumbers(searchQuery).First());
}
// Create reply email with sender and receiver addresses swapped.
MailMessage replyMessage = new MailMessage(
originalMessage.To[0],
originalMessage.From[0]);
// Add 'In-Reply-To' and 'References' header.
replyMessage.MimeEntity.Headers.Add(
new Header(HeaderId.InReplyTo, originalMessage.Id));
replyMessage.MimeEntity.Headers.Add(
new Header(HeaderId.References, originalMessage.Id));
// Set subject and body with appended original message.
replyMessage.Subject = $"Re: { originalMessage.Subject }";
replyMessage.BodyHtml = $@"<div>Yes, see you there at 9!</div>
<br><div>On {originalMessage.Date:G}, {originalMessage.From[0].Address} wrote:</div>
<blockquote style='border-left:1pt solid #E1E1E1;padding-left:5pt'>
{originalMessage.BodyHtml}
</blockquote>";
// Send reply email.
using (SmtpClient smtp = new SmtpClient("<ADDRESS> (e.g. smtp.gmail.com)"))
{
smtp.Connect();
smtp.Authenticate("<USERNAME>", "<PASSWORD>");
smtp.SendMessage(replyMessage);
}
}
}
Imports System.Linq
Imports GemBox.Email
Imports GemBox.Email.Imap
Imports GemBox.Email.Mime
Imports GemBox.Email.Smtp
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Retrieve original message.
Dim originalMessage As MailMessage
Using imap As New ImapClient("<ADDRESS> (e.g. imap.gmail.com)")
imap.Connect()
imap.Authenticate("<USERNAME>", "<PASSWORD>")
imap.SelectInbox()
Dim searchQuery As String = "SUBJECT ""Are you coming to the party?"""
originalMessage = imap.GetMessage(imap.SearchMessageNumbers(searchQuery).First())
End Using
' Create reply email with sender and receiver addresses swapped.
Dim replyMessage As New MailMessage(
originalMessage.To(0),
originalMessage.From(0))
' Add 'In-Reply-To' and 'References' header.
replyMessage.MimeEntity.Headers.Add(
New Header(HeaderId.InReplyTo, originalMessage.Id))
replyMessage.MimeEntity.Headers.Add(
New Header(HeaderId.References, originalMessage.Id))
' Set subject and body with appended original message.
replyMessage.Subject = $"Re: { originalMessage.Subject }"
replyMessage.BodyHtml += $"<div>Yes, see you there at 9!</div>
<br><div>On {originalMessage.Date:G}, {originalMessage.From(0).Address} wrote:</div>
<blockquote style='border-left:1pt solid #E1E1E1;padding-left:5pt'>
{originalMessage.BodyHtml}
</blockquote>"
' Send reply email.
Using smtp As New SmtpClient("<ADDRESS> (e.g. smtp.gmail.com)")
smtp.Connect()
smtp.Authenticate("<USERNAME>", "<PASSWORD>")
smtp.SendMessage(replyMessage)
End Using
End Sub
End Module
Even though there is no standard that defines the format for quoting the original message, for a HTML message, the common approach is to use the <blockquote>
tag (as shown in above example) with some styling, and for a plain text message the common approach is to quote every line of text by prefixing it with the '>'
character.
If the email has a reply chain or multiple reply messages, then a References
header should contain those message IDs separated with the space character.
Also, you can use the The difference between the forward and reply is that the forwarded message is sent to another recipient while the reply is sent to the sender of the original email. Also, typically when you forward a message you include all the attachments from the original message. But when you reply to a message the attachments are not included because you would be sending the same attachments back to the person who sent them to you. The following example shows how you can create a forward email in C# and VB.NET that includes an original email's subject prefixed with "Fw:", HTML body append at its end, and attachments.MailMessage.ReplyTo
collection to specify the list of addresses for the email reply. In other words, when clicking to a reply in your email client, the MailMessage.ReplyTo
addresses should be used as the recipient addresses instead of the usual MailMessage.From
addresses.Forward an Email
using System.Linq;
using GemBox.Email;
using GemBox.Email.Imap;
using GemBox.Email.Mime;
using GemBox.Email.Smtp;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Retrieve original message.
MailMessage originalMessage;
using (ImapClient imap = new ImapClient("<ADDRESS> (e.g. imap.gmail.com)"))
{
imap.Connect();
imap.Authenticate("<USERNAME>", "<PASSWORD>");
imap.SelectInbox();
string searchQuery = @"SUBJECT ""Are you coming to the party?""";
originalMessage = imap.GetMessage(imap.SearchMessageNumbers(searchQuery).First());
}
// Create forward email.
MailMessage forwardMessage = new MailMessage(
"receiver@example.com",
"anoter.receiver@example.com");
// Set subject and body with appended original message.
forwardMessage.Subject = $"Fw: {originalMessage.Subject}";
forwardMessage.BodyHtml = $@"<div>Are you interested in coming with me to this party?</div>
<br><div>On {originalMessage.Date:G}, {originalMessage.From[0].Address} wrote:</div>
<blockquote style='border-left:1pt solid #E1E1E1;padding-left:5pt'>
{originalMessage.BodyHtml}
</blockquote>";
// Add original attachments.
foreach (var attachment in originalMessage.Attachments)
forwardMessage.Attachments.Add(attachment);
// Send forward email.
using (SmtpClient smtp = new SmtpClient("<ADDRESS> (e.g. smtp.gmail.com)"))
{
smtp.Connect();
smtp.Authenticate("<USERNAME>", "<PASSWORD>");
smtp.SendMessage(forwardMessage);
}
}
}
Imports System.Linq
Imports GemBox.Email
Imports GemBox.Email.Imap
Imports GemBox.Email.Mime
Imports GemBox.Email.Smtp
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Retrieve original message.
Dim originalMessage As MailMessage
Using imap As New ImapClient("<ADDRESS> (e.g. imap.gmail.com)")
imap.Connect()
imap.Authenticate("<USERNAME>", "<PASSWORD>")
imap.SelectInbox()
Dim searchQuery As String = "SUBJECT ""Are you coming to the party?"""
originalMessage = imap.GetMessage(imap.SearchMessageNumbers(searchQuery).First())
End Using
' Create forward email.
Dim forwardMessage As New MailMessage(
"receiver@example.com",
"anoter.receiver@example.com");
' Set subject and body with appended original message.
forwardMessage.Subject = $"Fw: {originalMessage.Subject}"
forwardMessage.BodyHtml += $"<div>Are you interested in coming with me to this party?</div>
<br><div>On {originalMessage.Date:G}, {originalMessage.From(0).Address} wrote:</div>
<blockquote style='border-left:1pt solid #E1E1E1;padding-left:5pt'>
{originalMessage.BodyHtml}
</blockquote>"
' Add original attachments.
For Each attachment In originalMessage.Attachments
forwardMessage.Attachments.Add(attachment)
Next
' Send forward email.
Using smtp As New SmtpClient("<ADDRESS> (e.g. smtp.gmail.com)")
smtp.Connect()
smtp.Authenticate("<USERNAME>", "<PASSWORD>")
smtp.SendMessage(forwardMessage)
End Using
End Sub
End Module