Receive and Read Email in C# and VB.NET
GemBox.Email can receive and read emails using either POP3 or IMAP4 protocols, from your C# and VB.NET applications.
Which protocol you'll use depends on your requirement. In essence, their main difference is that POP is used for downloading and reading emails locally and then deleting them on the server (client storage), while IMAP is used for fetching copies of emails and reading them locally, but leaving the original emails on the server (server storage).
The following example shows how you can receive email messages using PopClient
.
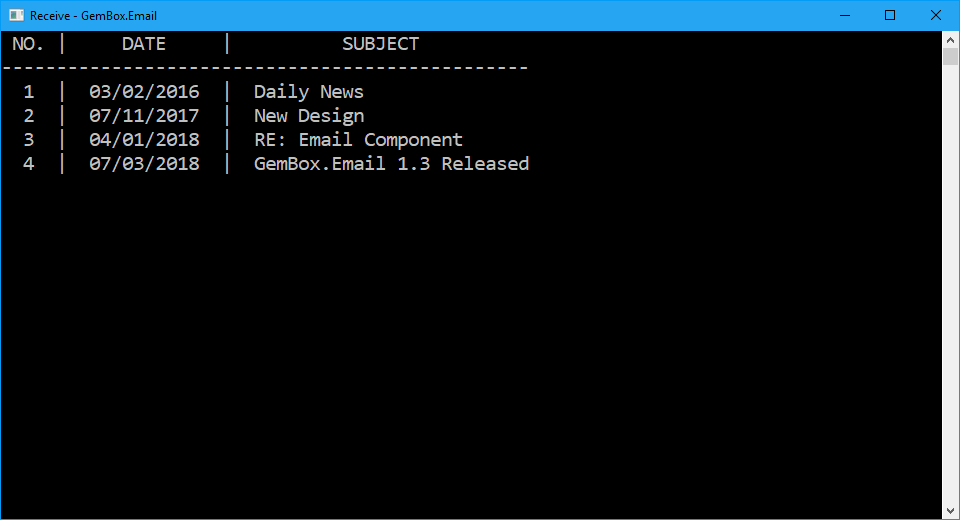
using System;
using GemBox.Email;
using GemBox.Email.Pop;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Create POP client.
using (var pop = new PopClient("<ADDRESS> (e.g. pop.gmail.com)"))
{
// Connect and sign to POP server.
pop.Connect();
pop.Authenticate("<USERNAME>", "<PASSWORD>");
// Read the number of currently available emails on the server.
int count = pop.GetCount();
Console.WriteLine(" NO. | DATE | SUBJECT ");
Console.WriteLine("------------------------------------------------");
// Download and receive all email messages.
for (int number = 1; number <= count; number++)
{
MailMessage message = pop.GetMessage(number);
// Read and display email's date and subject.
Console.WriteLine($" {number} | {message.Date.ToShortDateString()} | {message.Subject}");
}
}
}
}
Imports System
Imports GemBox.Email
Imports GemBox.Email.Pop
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Create POP client.
Using pop As New PopClient("<ADDRESS> (e.g. pop.gmail.com)")
' Connect and sign to POP server.
pop.Connect()
pop.Authenticate("<USERNAME>", "<PASSWORD>")
' Read the number of currently available emails on the server.
Dim count As Integer = pop.GetCount()
Console.WriteLine(" NO. | DATE | SUBJECT ")
Console.WriteLine("------------------------------------------------")
' Download and receive all email messages.
For number As Integer = 1 To count
Dim message As MailMessage = pop.GetMessage(number)
' Read and display email's date and subject.
Console.WriteLine($" {number} | {message.Date.ToShortDateString()} | {message.Subject}")
Next
End Using
End Sub
End Module
This next example does the same, but instead with ImapClient
.
using System;
using GemBox.Email;
using GemBox.Email.Imap;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Create IMAP client.
using (var imap = new ImapClient("<ADDRESS> (e.g. imap.gmail.com)"))
{
// Connect and sign to IMAP server.
imap.Connect();
imap.Authenticate("<USERNAME>", "<PASSWORD>");
// Select INBOX folder.
imap.SelectInbox();
// Read the number of currently available emails in selected mailbox folder.
int count = imap.SelectedFolder.Count;
Console.WriteLine(" NO. | DATE | SUBJECT ");
Console.WriteLine("------------------------------------------------");
// Download and receive all email messages from selected mailbox folder.
for (int number = 1; number <= count; number++)
{
MailMessage message = imap.GetMessage(number);
// Read and display email's date and subject.
Console.WriteLine($" {number} | {message.Date.ToShortDateString()} | {message.Subject}");
}
}
}
}
Imports System
Imports GemBox.Email
Imports GemBox.Email.Imap
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Create IMAP client.
Using imap As New ImapClient("<ADDRESS> (e.g. imap.gmail.com)")
' Connect and sign to IMAP server.
imap.Connect()
imap.Authenticate("<USERNAME>", "<PASSWORD>")
' Select INBOX folder.
imap.SelectInbox()
' Read the number of currently available emails in selected mailbox folder.
Dim count As Integer = imap.SelectedFolder.Count
Console.WriteLine(" NO. | DATE | SUBJECT ")
Console.WriteLine("------------------------------------------------")
' Download and receive all email messages from selected mailbox folder.
For number As Integer = 1 To count
Dim message As MailMessage = imap.GetMessage(number)
' Read and display email's date and subject.
Console.WriteLine($" {number} | {message.Date.ToShortDateString()} | {message.Subject}")
Next
End Using
End Sub
End Module
Both PopClient
and ImapClient
provide two ways for receiving emails. One way is the PopClient.GetMessage
or ImapClient.GetMessage
methods that are used in the above examples, which parse the downloaded emails from the server and return a MailMessage
object that can be further read with C# and VB.NET code.
The other way is the PopClient.SaveMessage
or ImapClient.SaveMessage
methods that can be used to save the downloaded email to a file or a stream without parsing it. To parse an email from a file or a stream, you can use MailMessage.Load
methods, as shown in Load example.
For more information about our email clients, you can refer to our Pop Client or Imap Client examples. To use POP or IMAP protocols with most major email providers, you'll first need to enable this connection by modifying your email account's security settings. The following links describe how to enable these settings: After setting the security settings, you can connect to your POP or IMAP server and read your mailbox by using the following server settings:Using POP or IMAP with Gmail, Outlook and Yahoo
Provider POP3 Host Port ConnectionSecurity Gmail pop.gmail.com 995 SSL Outlook pop-mail.outlook.com 995 SSL Office 365 outlook.office365.com 995 SSL Yahoo pop.mail.yahoo.com 995 SSL AOL pop.aol.com 995 SSL Provider IMAP4 Host Port ConnectionSecurity Gmail imap.gmail.com 993 SSL Outlook imap-mail.outlook.com 993 SSL Office 365 outlook.office365.com 993 SSL Yahoo imap.mail.yahoo.com 993 SSL AOL imap.aol.com 993 SSL