List and modify folders on Exchange Server
The example below shows how you can list all folders on an Exchange server, create a new one, and then remove it using the GemBox.Email library in C# and VB.NET.
using GemBox.Email;
using GemBox.Email.Exchange;
using System;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Create a new Exchange client.
var exchangeClient = new ExchangeClient("<HOST> (e.g. https://outlook.office365.com/EWS/Exchange.asmx)");
exchangeClient.Authenticate("<USERNAME>", "<PASSWORD>");
// Create a new folder.
exchangeClient.CreateFolder("GemBox Folder");
// List folders on the server.
var folders = exchangeClient.ListFolders();
// Print folder info.
Console.WriteLine("Folder name".PadRight(28, ' ') + " | Items | Unread items | Children folders");
foreach (ExchangeFolderInfo folder in folders)
Console.WriteLine(
$"{folder.Name,-28} | " +
$"{folder.TotalCount,-5} | " +
$"{folder.UnreadCount,-12} | " +
$"{folder.ChildFolderCount,-16}");
// Delete a folder.
exchangeClient.DeleteFolder("GemBox Folder", false);
}
}
Imports GemBox.Email
Imports GemBox.Email.Exchange
Imports System
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Create a new Exchange client.
Dim exchangeClient = New ExchangeClient("<HOST> (e.g. https://outlook.office365.com/EWS/Exchange.asmx)")
exchangeClient.Authenticate("<USERNAME>", "<PASSWORD>")
' Create a new folder.
exchangeClient.CreateFolder("GemBox Folder")
' List folders on the server.
Dim folders = exchangeClient.ListFolders()
' Print folder info.
Console.WriteLine("Folder name".PadRight(28, " "c) + " | Items | Unread items | Children folders")
For Each folder As ExchangeFolderInfo In folders
Console.WriteLine(
$"{folder.Name,-28} | " +
$"{folder.TotalCount,-5} | " +
$"{folder.UnreadCount,-12} | " +
$"{folder.ChildFolderCount,-16}")
Next
' Delete a folder.
exchangeClient.DeleteFolder("GemBox Folder", False)
End Sub
End Module
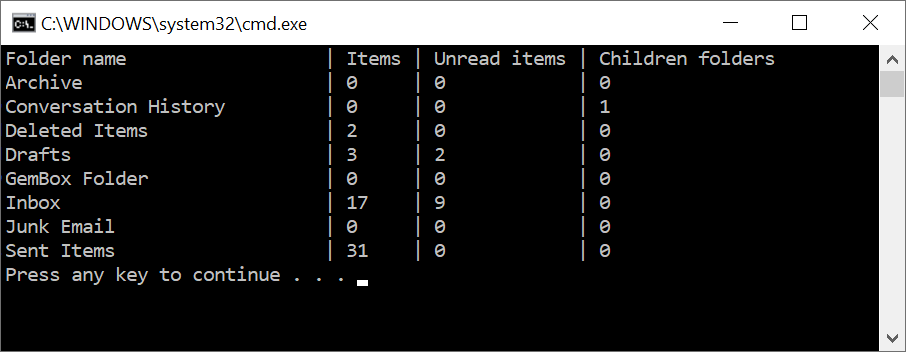
To modify folders on the Exchange server, you can use the ExchangeClient.CreateFolder
, ExchangeClient.DeleteFolder
, and ExchangeClient.RenameFolder
methods. With the ExchangeClient.GetFolderInfo
method, you can obtain information about folders like name, total messages count and unread messages count.