Load and Save a Mbox Message Collection
GemBox.Email provides an advanced API for working with the Mbox (Mboxrd) file format, allowing you to load, modify and save email in C# and VB.NET, as shown in the example below.
using GemBox.Email;
using System;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Load message collection.
MailMessageCollection messages = MailMessageCollection.Load("%InputFileName%");
// Display message information.
foreach (MailMessage message in messages)
{
Console.WriteLine($"From: {message.From}");
Console.WriteLine($"Subject: {message.Subject}");
Console.WriteLine($"Body: {message.BodyText}");
Console.WriteLine();
}
// Create new message.
MailMessage newMessage = new MailMessage("sender@example.com", "receiver@example.com")
{
Subject = "Test email message with a text body",
BodyText = "This is a test message with a text body."
};
// Add message to collection.
messages.Add(newMessage);
// Save message collection.
messages.Save("Modified Collection.mbox");
}
}
Imports GemBox.Email
Imports System
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Load message collection.
Dim messages As MailMessageCollection = MailMessageCollection.Load("%InputFileName%")
' Display message information.
For Each message As MailMessage In messages
Console.WriteLine($"From: {message.From}")
Console.WriteLine($"Subject: {message.Subject}")
Console.WriteLine($"Body: {message.BodyText}")
Console.WriteLine()
Next
' Create new message.
Dim newMessage As New MailMessage("sender@example.com", "receiver@example.com") With
{
.Subject = "Test email message with a text body",
.BodyText = "This is a test message with a text body."
}
' Add message to the collection
messages.Add(newMessage)
' Save message collection
messages.Save("Modified Collection.mbox")
End Sub
End Module
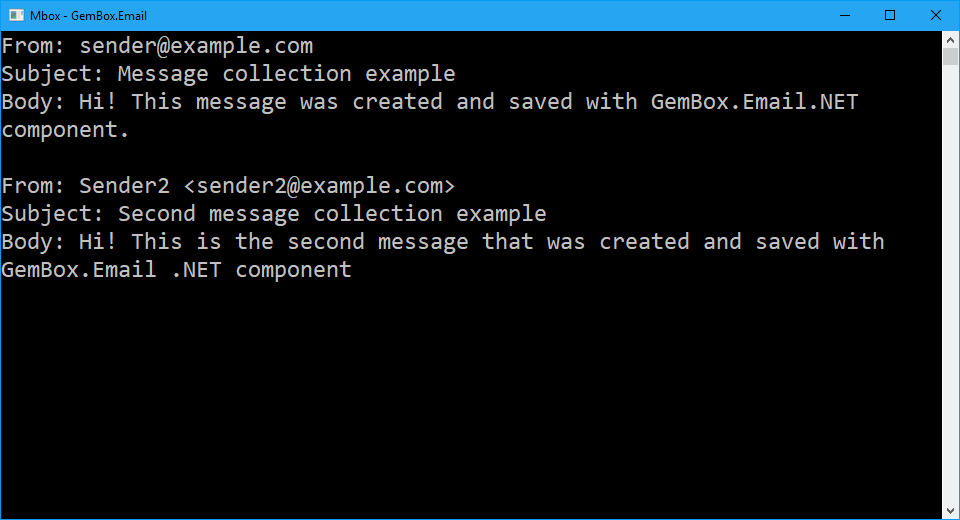
Besides basic features like loading (via MailMessageCollection.Load
methods), modifying and saving (via MailMessageCollection.Save
methods), you can use different loading methods, which speeds up the process.
For example, you can use MailMessageCollection.LazyLoad
for lazy message iteration or MailMessageCollection.LoadHeaders
for reading just message headers.