List and manipulate messages on Exchange Server
The following example shows how you can use GemBox.Email to list the first 10 messages from an Exchange server, print the body of unread messages, and mark them as read.
using GemBox.Email;
using GemBox.Email.Exchange;
using System;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Create a new Exchange client.
var exchangeClient = new ExchangeClient("<HOST> (e.g. https://outlook.office365.com/EWS/Exchange.asmx)");
exchangeClient.Authenticate("<USERNAME>", "<PASSWORD>");
// List the first ten messages in the Inbox folder.
var messageInfos = exchangeClient.ListMessages("Inbox", 0, 10);
foreach (ExchangeMessageInfo messageInfo in messageInfos)
{
if (!messageInfo.IsRead)
{
// Get the full mail message.
var mailMessage = exchangeClient.GetMessage(messageInfo.ExchangeMessageId);
Console.WriteLine("From: " + string.Join(", ", mailMessage.From));
Console.WriteLine(mailMessage.BodyHtml);
Console.WriteLine();
// Mark message as read.
exchangeClient.MarkMessageAsRead(messageInfo.ExchangeMessageId);
}
}
}
}
Imports GemBox.Email
Imports GemBox.Email.Exchange
Imports System
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Create a new Exchange client.
Dim exchangeClient = New ExchangeClient("<HOST> (e.g. https://outlook.office365.com/EWS/Exchange.asmx)")
exchangeClient.Authenticate("<USERNAME>", "<PASSWORD>")
' List the first ten messages in the Inbox folder.
Dim messageInfos = exchangeClient.ListMessages("Inbox", 0, 10)
For Each messageInfo As ExchangeMessageInfo In messageInfos
If Not messageInfo.IsRead Then
' Get the full mail message.
Dim mailMessage = exchangeClient.GetMessage(messageInfo.ExchangeMessageId)
Console.WriteLine("From: " + String.Join(", ", mailMessage.From))
Console.WriteLine(mailMessage.BodyHtml)
Console.WriteLine()
' Mark message as read.
exchangeClient.MarkMessageAsRead(messageInfo.ExchangeMessageId)
End If
Next
End Sub
End Module
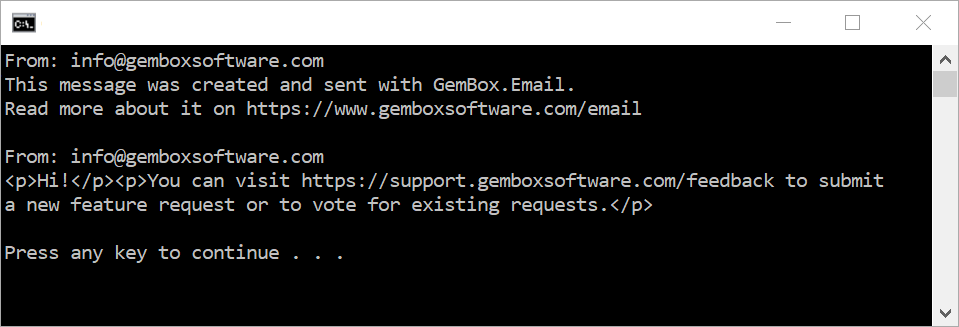
You can use the ExchangeClient.ListMessages
method to list messages in a folder and the ExchangeClient.GetMessage
method to obtain more information about a given message.
You can manipulate existing messages by using one of the following methods:
ExchangeClient.DeleteMessage
- deletes a messageExchangeClient.MoveMessage
- moves a message from one folder to anotherExchangeClient.CopyMessage
- copies a message to another folderExchangeClient.MarkMessageAsRead
- marks a message as readExchangeClient.MarkMessageAsUnread
- marks a message as unread