Email Message Headers
The example below shows how to use the GemBox.Email library to modify header values manually or through provided properties in your C# and VB.NET applications. It also shows the link between headers and properties and how they are updated automatically.
using GemBox.Email;
using GemBox.Email.Mime;
using System;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Create new message.
MailMessage message = new MailMessage("sender@example.com", "receiver@example.com");
// Get 'From' property and 'From' header.
MailAddressCollection fromAddresses = message.From;
Header fromHeader = message.MimeEntity.Headers[HeaderId.From];
Console.WriteLine($"Original 'From' property value: {fromAddresses}");
// Change 'From' header value.
fromHeader.Body = "new.sender@example.com";
Console.WriteLine($"Modified 'From' property value: {fromAddresses}");
// Get 'To' property and 'To' header.
MailAddressCollection toAddresses = message.To;
Header toHeader = message.MimeEntity.Headers[HeaderId.To];
Console.WriteLine($"Original 'To' header value: {toHeader.Body}");
// Change 'To' property value.
toAddresses[0].Address = "new.receiver@example.com";
Console.WriteLine($"Modified 'To' header value: {toHeader.Body}");
}
}
Imports GemBox.Email
Imports GemBox.Email.Mime
Imports System
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Create new message.
Dim message As New MailMessage("sender@example.com", "receiver@example.com")
' Get 'From' property and 'From' header.
Dim fromAddresses As MailAddressCollection = message.From
Dim fromHeader As Header = message.MimeEntity.Headers(HeaderId.From)
Console.WriteLine($"Original 'From' property value: {fromAddresses}")
' Change 'From' header value.
fromHeader.Body = "new.sender@example.com"
Console.WriteLine($"Modified 'From' property value: {fromAddresses}")
' Get 'To' property and 'To' header.
Dim toAddresses As MailAddressCollection = message.To
Dim toHeader As Header = message.MimeEntity.Headers(HeaderId.To)
Console.WriteLine($"Original 'To' header value: {toHeader.Body}")
' Change 'To' property value.
toAddresses(0).Address = "new.receiver@example.com"
Console.WriteLine($"Modified 'To' header value: {toHeader.Body}")
End Sub
End Module
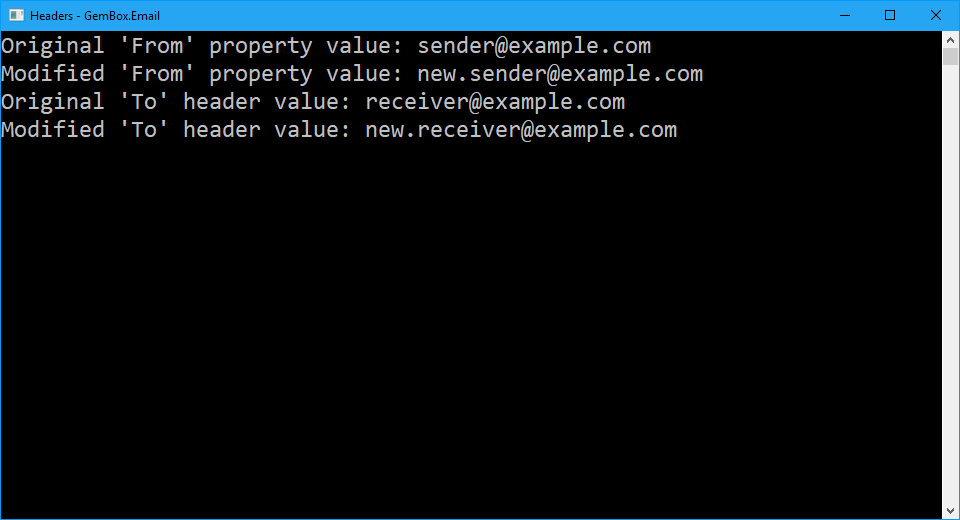
The MailMessage
object contains properties for required and commonly used message headers, such as MailMessage.Subject
, MailMessage.Sender
, etc.
It's also possible to add and remove headers from the HeaderCollection
collection.
The GemBox.Email Header class follows the RFC 5322 standard. A header consists of two parts: a name and a body. The first part (name) cannot be empty, while the body can be.
GemBox.Email supports adding both standard and custom headers. To add standard headers, use the The following example shows how you can add additional headers to an email message.HeaderId
identifiers.Add additional headers
using GemBox.Email;
using GemBox.Email.Mime;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Create new message.
MailMessage message = new MailMessage("sender@example.com", "receiver@example.com")
{
Subject = "Example Message",
BodyText = "High priority email with comments."
};
// Add message comments.
message.MimeEntity.Headers.Add(
new Header(HeaderId.Comments, "Example of mail message comment"));
// Add message priority.
message.MimeEntity.Headers.Add(
new Header("Importance", "high"));
message.MimeEntity.Headers.Add(
new Header("Priority", "urgent"));
message.MimeEntity.Headers.Add(
new Header("X-Priority", "1"));
message.Save("High Priority Message.%OutputFileType%");
}
}
Imports GemBox.Email
Imports GemBox.Email.Mime
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Create new message.
Dim message As New MailMessage("sender@example.com", "receiver@example.com") With
{
.Subject = "Example Message",
.BodyText = "High priority email with comments."
}
' Add message comments.
message.MimeEntity.Headers.Add(
New Header(HeaderId.Comments, "Example of mail message comment"))
' Add message priority.
message.MimeEntity.Headers.Add(
New Header("Importance", "high"))
message.MimeEntity.Headers.Add(
New Header("Priority", "urgent"))
message.MimeEntity.Headers.Add(
New Header("X-Priority", "1"))
message.Save("High Priority Message.%OutputFileType%")
End Sub
End Module