Get email messages in Xamarin
The following example shows how you can use GemBox.Email to get the first email message from the INBOX using the ImapClient
in Xamarin.Forms application, on Android and iOS mobile devices..
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
xmlns:d="http://xamarin.com/schemas/2014/forms/design"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
x:Class="MainPage">
<StackLayout Padding="50"
Spacing="20" >
<Label Text="GemBox.Email Example"
HorizontalOptions="Center"
FontSize="Large"
Margin="0,0,0,30"/>
<Entry Placeholder="Imap host"
Text="{Binding Host}" />
<Entry Placeholder="User name"
Text="{Binding Username}" />
<Entry Placeholder="Password"
IsPassword="True"
Text="{Binding Password}" />
<ActivityIndicator x:Name="activity" />
<Button x:Name="button"
VerticalOptions="End"
Text="Get message"
Clicked="Button_Clicked" HorizontalOptions="Fill" />
</StackLayout>
</ContentPage>
using GemBox.Email;
using GemBox.Email.Imap;
using System;
using System.ComponentModel;
using System.Threading.Tasks;
using Xamarin.Forms;
public partial class MainPage : ContentPage
{
private readonly DataModel model;
public MainPage()
{
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
InitializeComponent();
model = new DataModel();
BindingContext = model;
}
private MailMessage GetMessage()
{
using (var imap = new ImapClient(model.Host))
{
imap.Connect();
imap.Authenticate(model.Username, model.Password);
imap.SelectInbox();
return imap.GetMessage(1);
}
}
private async void Button_Clicked(object sender, EventArgs e)
{
button.IsEnabled = false;
activity.IsRunning = true;
try
{
var message = await Task.Run(() => GetMessage());
await DisplayAlert("Message",
"\nSUBJECT: " + message.Subject +
"\nFROM: " + message.From.ToString() +
"\nDATE: " + message.Date.ToString(),
"Close");
}
catch (Exception ex)
{
await DisplayAlert("Error", ex.Message, "Close");
}
activity.IsRunning = false;
button.IsEnabled = true;
}
}
public class DataModel
{
public string Host { get; set; }
public string Username { get; set; }
public string Password { get; set; }
}
Imports GemBox.Email
Imports GemBox.Email.Imap
Imports System
Imports System.ComponentModel
Imports System.Threading.Tasks
Imports Xamarin.Forms
Partial Public Class MainPage
Inherits ContentPage
Private ReadOnly model As DataModel
Public Sub New()
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
InitializeComponent()
model = New DataModel()
BindingContext = model
End Sub
Private Function GetMessage() As MailMessage
Using imap As New ImapClient(model.Host)
imap.Connect()
imap.Authenticate(model.Username, model.Password)
imap.SelectInbox()
Return imap.GetMessage(1)
End Using
End Function
Private Async Sub Button_Clicked(sender As Object, e As EventArgs)
button.IsEnabled = False
activity.IsRunning = True
Try
Dim message = Await Task.Run(Function() GetMessage())
Await DisplayAlert("Message",
"\nSUBJECT: " + message.Subject &
"\nFROM: " + message.From.ToString() &
"\nDATE: " + message.Date.ToString(),
"Close")
Catch ex As Exception
Await DisplayAlert("Error", ex.Message, "Close")
End Try
activity.IsRunning = False
button.IsEnabled = True
End Sub
End Class
Public Class DataModel
Public Property Host As String
Public Property Username As String
Public Property Password As String
End Class
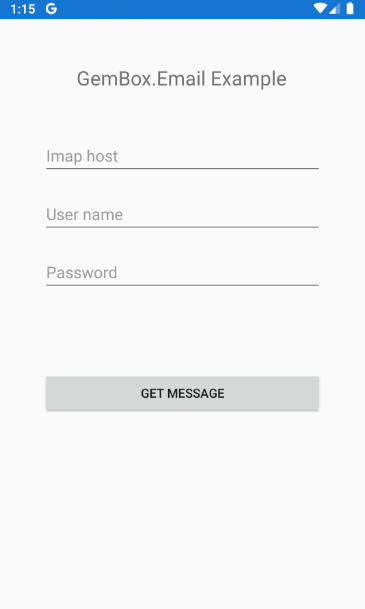