Get email messages in MAUI
GemBox.Email is a standalone .NET component with cross-platform support for processing emails. With the help of MAUI, you can use it on platforms like Android, iOS, Linux, and macOS for sending, receiving, loading, saving, editing, and converting EML and MSG files.
The following example shows how to use GemBox.Email to get the first email message from INBOX using ImapClient
in the MAUI application on Android, iOS, Linux, and macOS.
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="EmailMaui.MainPage">
<ScrollView>
<VerticalStackLayout
Spacing="25"
Padding="30"
VerticalOptions="Center">
<Label Text="GemBox.Email Example"
HorizontalOptions="Center"
FontSize="Large"
Margin="0,0,0,30"/>
<Entry Placeholder="Imap host"
Text="{Binding Host}" />
<Entry Placeholder="User name"
Text="{Binding Username}" />
<Entry Placeholder="Password"
IsPassword="True"
Text="{Binding Password}" />
<ActivityIndicator x:Name="activity" />
<Button x:Name="button"
VerticalOptions="End"
Text="Get message"
Clicked="Button_Clicked" HorizontalOptions="Fill" />
</VerticalStackLayout>
</ScrollView>
</ContentPage>
using GemBox.Email;
using GemBox.Email.Imap;
namespace EmailMaui
{
public partial class MainPage : ContentPage
{
private readonly DataModel model;
static MainPage()
{
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
}
public MainPage()
{
InitializeComponent();
this.BindingContext = model = new DataModel();
}
private MailMessage GetMessage()
{
using (var imap = new ImapClient(model.Host))
{
imap.Connect();
imap.Authenticate(model.Username, model.Password);
imap.SelectInbox();
return imap.GetMessage(1);
}
}
private async void Button_Clicked(object sender, EventArgs e)
{
button.IsEnabled = false;
activity.IsRunning = true;
try
{
var message = await Task.Run(GetMessage);
await DisplayAlert("Message",
"\nSUBJECT: " + message.Subject +
"\nFROM: " + message.From.ToString() +
"\nDATE: " + message.Date.ToString(),
"Close");
}
catch (Exception ex)
{
await DisplayAlert("Error", ex.Message, "Close");
}
activity.IsRunning = false;
button.IsEnabled = true;
}
}
public class DataModel
{
public string Host { get; set; }
public string Username { get; set; }
public string Password { get; set; }
}
}
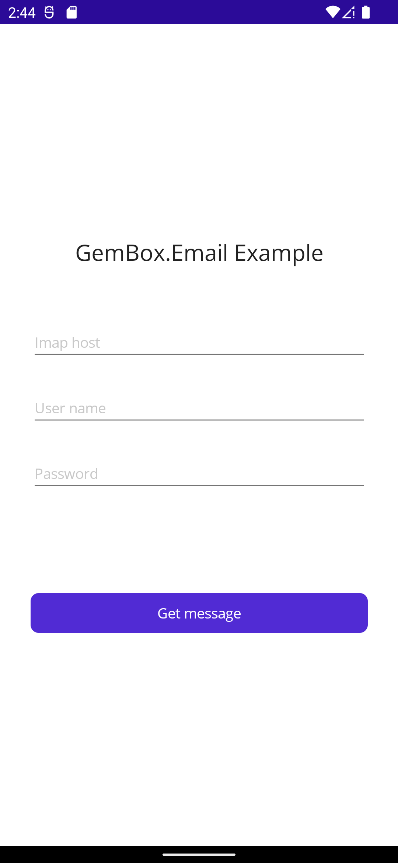