Folder Flags
This example shows how to use the GemBox.Email library to list IMAP folders and their flags.
using GemBox.Email;
using GemBox.Email.Imap;
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
using (ImapClient imap = new ImapClient("<ADDRESS> (e.g. imap.gmail.com)"))
{
imap.Connect();
imap.Authenticate("<USERNAME>", "<PASSWORD>");
// Select INBOX folder.
imap.SelectInbox();
// List INBOX folder flags.
IList<ImapFolderInfo> folders = imap.ListFolders();
foreach (string flag in imap.SelectedFolder.Flags)
Console.WriteLine(flag);
}
}
}
Imports GemBox.Email
Imports GemBox.Email.Imap
Imports System
Imports System.Collections.Generic
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Using imap As New ImapClient("<ADDRESS> (e.g. imap.gmail.com)")
imap.Connect()
imap.Authenticate("<USERNAME>", "<PASSWORD>")
' Select INBOX folder.
imap.SelectInbox()
' List INBOX folder flags.
Dim folders As IList(Of ImapFolderInfo) = imap.ListFolders()
For Each flag As String In imap.SelectedFolder.Flags
Console.WriteLine(flag)
Next
End Using
End Sub
End Module
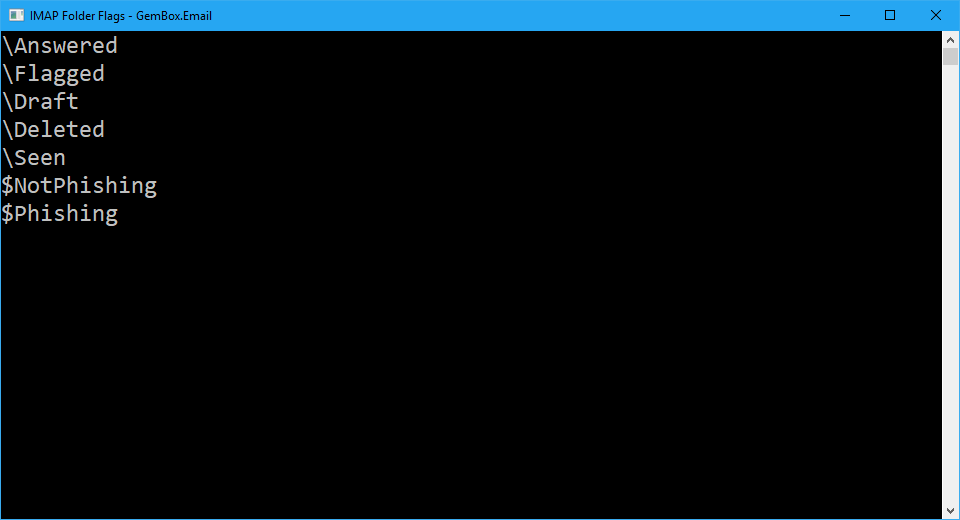
IMAP folder flags provide additional information about a folder, such as if a folder is selected, if it has any subfolders, etc.
GemBox.Email represents each folder's flags with a read-only ImapFolderInfo.Flags
collection. The possible flags that can occur are listed as an ImapFolderFlag
enumeration.
For more information about ImapClient
, check out our IMAP Client Connection example.