Create and Save a Calendar
The following example shows how you can use GemBox.Email to create a new Calendar
with Event
, Task
, and Reminder
and then save it as a new iCalendar file.
using GemBox.Email;
using GemBox.Email.Calendar;
using System;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Create new calendar.
Calendar calendar = new Calendar();
// Create new event.
Event ev = new Event();
ev.Organizer = new MailAddress("info@gemboxsoftware.com", "GemBox Ltd.");
ev.Name = "GemBox Meeting Request";
ev.Location = "152 City Road, London";
ev.Start = new DateTime(2018, 1, 10, 9, 0, 0, DateTimeKind.Utc);
ev.End = new DateTime(2018, 1, 12, 18, 0, 0, DateTimeKind.Utc);
ev.Attendees.Add(new MailAddress("invited@example.com"));
// Create new reminder.
Reminder reminder = new Reminder();
reminder.ReminderAction = ReminderAction.Email;
reminder.ReminderAttendees.Add(new MailAddress("invited@example.com"));
reminder.TriggerBeforeStart = new TimeSpan(2, 0, 0, 0);
// Add reminder to the event.
ev.Reminders.Add(reminder);
// Add event to the calendar.
calendar.Events.Add(ev);
// Create new task.
Task task = new Task();
task.Organizer = new MailAddress("info@gemboxsoftware.com", "GemBox Ltd.");
task.Name = "Implement iCalendar Support";
task.Start = new DateTime(2018, 1, 1, 0, 0, 0, DateTimeKind.Utc);
task.Deadline = new DateTime(2018, 1, 15, 0, 0, 0, DateTimeKind.Utc);
task.Priority = 5;
task.Categories.Add("New '.ics' format support");
task.Attendees.Add(new MailAddress("programmer@example.com"));
// Add task to the calendar.
calendar.Tasks.Add(task);
calendar.Save("Create And Save.ics");
}
}
Imports GemBox.Email
Imports GemBox.Email.Calendar
Imports System
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Create new calendar.
Dim calendar As New Calendar()
' Create new event.
Dim ev As New [Event]()
ev.Organizer = New MailAddress("info@gemboxsoftware.com", "GemBox Ltd.")
ev.Name = "GemBox Meeting Request"
ev.Location = "152 City Road, London"
ev.Start = New DateTime(2018, 1, 10, 9, 0, 0, DateTimeKind.Utc)
ev.End = New DateTime(2018, 1, 12, 18, 0, 0, DateTimeKind.Utc)
ev.Attendees.Add(New MailAddress("invited@example.com"))
' Create new reminder.
Dim reminder As New Reminder()
reminder.ReminderAction = ReminderAction.Email
reminder.ReminderAttendees.Add(New MailAddress("invited@example.com"))
reminder.TriggerBeforeStart = New TimeSpan(2, 0, 0, 0)
' Add reminder to the event.
ev.Reminders.Add(reminder)
' Add event to the calendar.
calendar.Events.Add(ev)
' Create new task.
Dim task As New Task()
task.Organizer = New MailAddress("info@gemboxsoftware.com", "GemBox Ltd.")
task.Name = "Implement iCalendar Support"
task.Start = New DateTime(2018, 1, 1, 0, 0, 0, DateTimeKind.Utc)
task.Deadline = New DateTime(2018, 1, 15, 0, 0, 0, DateTimeKind.Utc)
task.Priority = 5
task.Categories.Add("New '.ics' format support")
task.Attendees.Add(New MailAddress("programmer@example.com"))
' Add task to the calendar.
calendar.Tasks.Add(task)
calendar.Save("Create And Save.ics")
End Sub
End Module
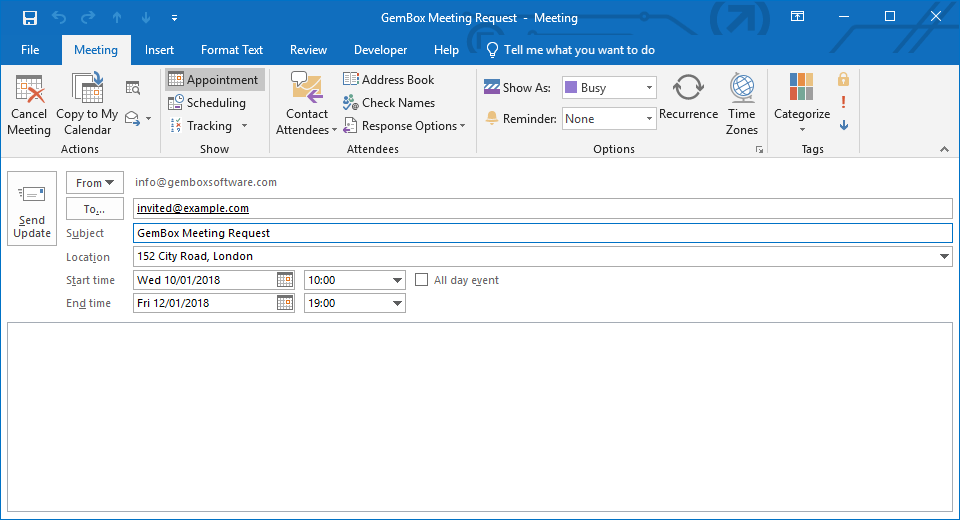
GemBox.Email provides a comprehensive but still very easy-to-use API for working with calendars, events, tasks and reminders in C# and VB.NET, as defined by RFC 5545 standard. That enables you to create reminders and alarms or to send invites, appointments, meeting requests, etc.
Apart from creating a new Calendar
object, you can load it from an iCalendar file (.ics), or retrieve it from an email with the MailMessage.Calendars
collection. You can also modify the Calendar
object, save it as a new or existing iCalendar file, or add it to the MailMessage.Calendars
collection.