Load Emails
The following example shows how to load an email message file and read its properties like date, subject, recipients, and body using GemBox.Email in C# or VB.NET
using GemBox.Email;
using System;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Load message from email file.
MailMessage message = MailMessage.Load("%InputFileName%");
// Read message information.
Console.WriteLine($"Date: {message.Date}");
Console.WriteLine($"Subject: {message.Subject}");
Console.WriteLine($"From: {message.From}");
Console.WriteLine($"To: {message.To}");
if (message.Cc.Count > 0)
Console.WriteLine($"Cc: {message.Cc}");
if (message.Bcc.Count > 0)
Console.WriteLine($"Bcc: {message.Bcc}");
if (message.Attachments.Count > 0)
Console.WriteLine($"Attachments: {message.Attachments.Count}");
Console.WriteLine();
if (string.IsNullOrEmpty(message.BodyHtml))
Console.WriteLine(message.BodyText);
else
Console.WriteLine(message.BodyHtml);
}
}
Imports GemBox.Email
Imports System
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Load message from email file.
Dim message As MailMessage = MailMessage.Load("%InputFileName%")
' Read message information.
Console.WriteLine($"Date: {message.Date}")
Console.WriteLine($"Subject: {message.Subject}")
Console.WriteLine($"From: {message.From}")
Console.WriteLine($"To: {message.To}")
If (message.Cc.Count > 0) Then
Console.WriteLine($"Cc: {message.Cc}")
End If
If (message.Bcc.Count > 0) Then
Console.WriteLine($"Bcc: {message.Bcc}")
End If
If (message.Attachments.Count > 0) Then
Console.WriteLine($"Attachments: {message.Attachments.Count}")
End If
Console.WriteLine()
If (String.IsNullOrEmpty(message.BodyHtml)) Then
Console.WriteLine(message.BodyText)
Else
Console.WriteLine(message.BodyHtml)
End If
End Sub
End Module
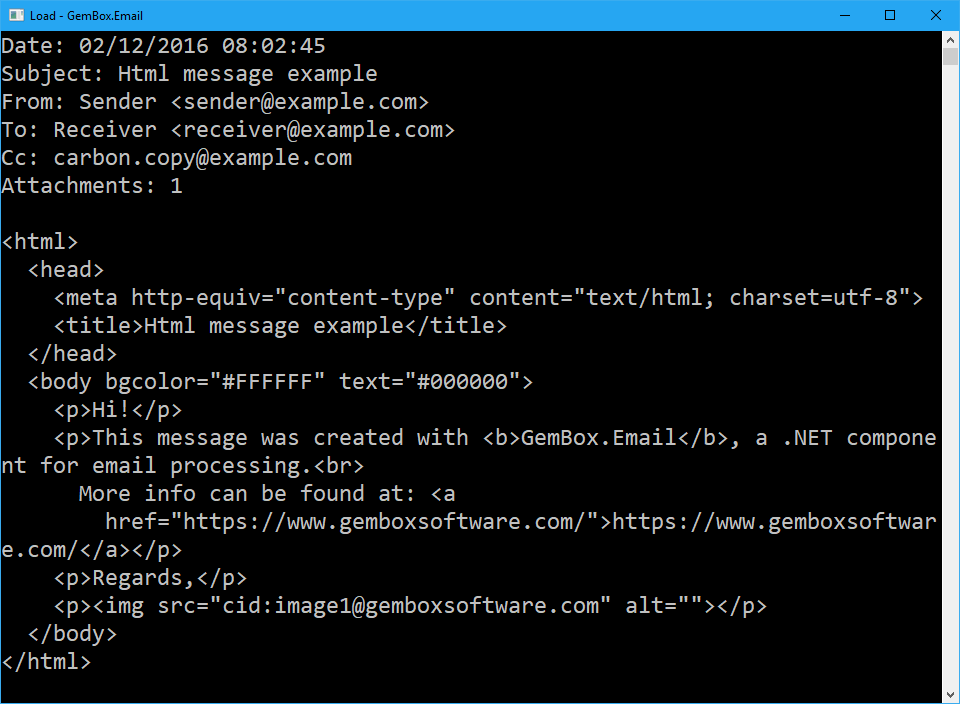
When using the MailMessage.Load(String)
method, GemBox.Email will select the required MailMessageFormat
based on the file's extension. So, for a file named Example.msg, it will use MailMessageFormat.Msg
and for a file named Example.eml, it will use MailMessageFormat.Eml
.