Getting started with GemBox.Email
GemBox.Email is a .NET component that enables you to read, write, and convert email files (MSG, EML, and MHTML), or send and receive email messages (POP, IMAP, SMTP, and EWS) from .NET applications. GemBox.Email requires only .NET. It supports a wide range of .NET versions, as listed below: Before you can use GemBox.Email, you need to install it in your project. The best way is by adding a GemBox.Email NuGet package via NuGet Package Manager in Visual Studio. To install the package, you can follow the steps listed below. However, please note that this guide assumes you have already set up a Visual Studio project. If you haven't done that yet, you can refer to the official tutorial for guidance. As an alternative, you can open the NuGet Package Manager Console (Tools -> NuGet Package Manager -> Package Manager Console) and run the following command: We also provide DLL and setup files on GemBox.Email's Downloads page. A link for the latest setup file is located at the bottom of the page, under the 'Global Assembly' section. Once you have installed GemBox.Email, all you have to do is add the using directive and make sure you call the The ComponentInfo.SetLicense requires a license key as a parameter. If you are using the component in a Free mode, use "FREE-LIMITED-KEY" as a license key, and if you have purchased a Professional license, use the key you got in your email after the purchase. You can read more about GemBox.Email's working modes on the Evaluation and Licensing page. After that, you can write your application code for working with emails, like the code below. It shows how to create a Requirements
Installation
Install-Package GemBox.Email
Usage
ComponentInfo.SetLicense
method before using any member of the component. We suggest putting the call at the beginning of the Main()
method.MailMessage
, set BodyText
and BodyHtml
, add some Attachments
, and send it using SmtpClient
.
using GemBox.Email;
using GemBox.Email.Smtp;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Create new email message.
MailMessage message = new MailMessage("sender@example.com", "receiver@example.com");
// Add subject.
message.Subject = "GemBox.Email getting started example";
// Add HTML body
message.BodyHtml =
"<html><body>" +
"<p>Hi 👋,</p>" +
"<p>This message was created and sent with <a href='https://www.gemboxsoftware.com/email'>GemBox.Email</a></p>" +
"</body></html>";
// Add text body
message.BodyText =
"Hi 👋,\n" +
"This message was created and sent with GemBox.Email (https://www.gemboxsoftware.com/email)";
// Add file as attachment.
message.Attachments.Add(new Attachment("%#GemBoxSampleFile.pdf%"));
// Create new SMTP client and send an email message.
using (SmtpClient smtp = new SmtpClient("<ADDRESS> (e.g. smtp.gmail.com)"))
{
smtp.Connect();
smtp.Authenticate("<USERNAME>", "<PASSWORD>");
smtp.SendMessage(message);
}
}
}
Imports GemBox.Email
Imports GemBox.Email.Smtp
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Create New email message.
Dim message = New MailMessage("sender@example.com", "receiver@example.com")
' Add subject.
message.Subject = "GemBox.Email getting started example"
' Add HTML body.
message.BodyHtml =
"<html><body>" &
"<p>Hi 👋,</p>" &
"<p>This message was created and sent with <a href='https://www.gemboxsoftware.com/email'>GemBox.Email</a></p>" &
"</body></html>"
' Add text body.
message.BodyText =
"Hi 👋,\n" &
"This message was created and sent with GemBox.Email (https://www.gemboxsoftware.com/email)"
' Add file as attachment.
message.Attachments.Add(New Attachment("%#GemBoxSampleFile.pdf%"))
' Create New SMTP client And send an email message.
Using smtp As New SmtpClient("<ADDRESS> (e.g. smtp.gmail.com)")
smtp.Connect()
smtp.Authenticate("<USERNAME>", "<PASSWORD>")
smtp.SendMessage(message)
End Using
End Sub
End Module
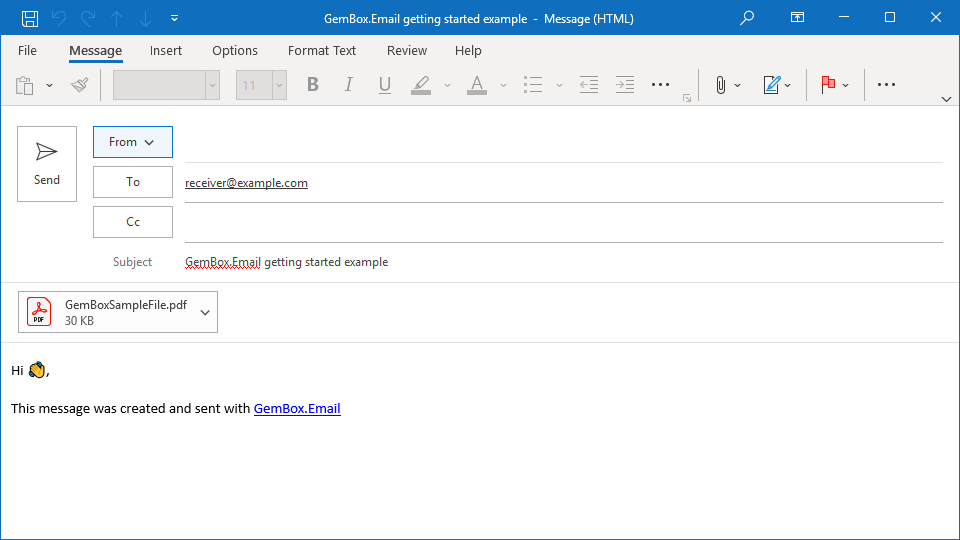
To learn more about GemBox.Email, check out our collection of examples listed on the menu. These examples will show you how to use the component in various email-processing tasks and platforms. Additionally, for detailed information on the API, head to our documentation pages. This resource offers a comprehensive API reference, explaining each method and property available within the component.