Create Emails
The following example shows how to create a simple message, with a subject and body, in C# or VB.NET using the GemBox.Email library.
using GemBox.Email;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Create new message.
MailMessage message = new MailMessage(
new MailAddress("sender@example.com", "Sender"),
new MailAddress("first.receiver@example.com", "First receiver"));
// Set subject and text body.
message.Subject = "Test email message with text body";
message.BodyText = "This is a test message with text body.";
message.Save("Create Message.%OutputFileType%");
}
}
Imports GemBox.Email
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Create new message.
Dim message As New MailMessage(
New MailAddress("sender@example.com", "Sender"),
New MailAddress("first.receiver@example.com", "First receiver"))
' Set subject And text body.
message.Subject = "Test email message with text body"
message.BodyText = "This is a test message with text body."
message.Save("Create Message.%OutputFileType%")
End Sub
End Module
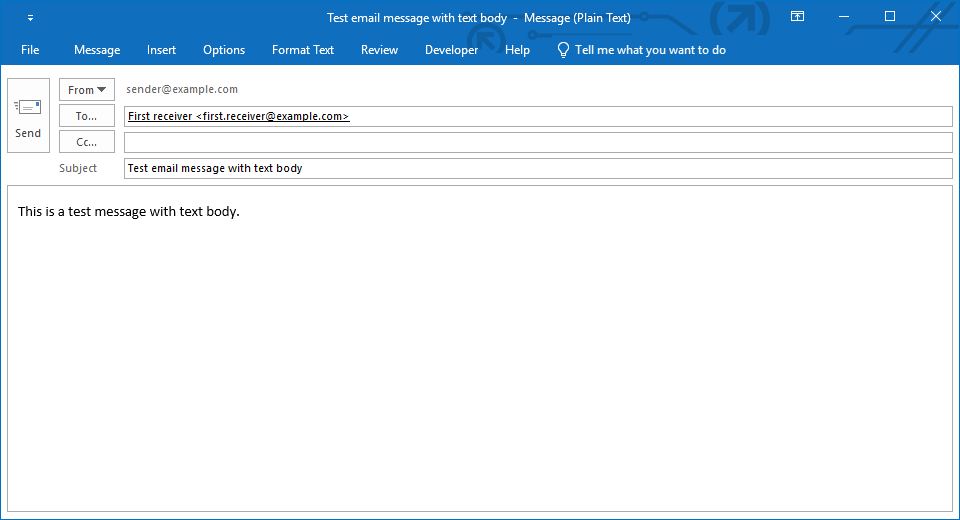
GemBox.Email represents an email with a MailMessage
object. This class provides properties and methods for specifying or accessing the email's sender, recipient, subject, body, and more. You can use it to generate new or inspect existing email messages.
With GemBox.Email, you can easily create more advanced email messages as well. For instance, you can check out our:
- Html With Attachment example, for creating emails with HTML content, CSS styling, embedded images and attachments.
- Header example, for creating emails with built-in or custom mail message headers.
- Calendar example, for creating emails with calendars.