Email Address Validation
The following example shows how to use GemBox.Email to validate an email address, or a list of email addresses from your C# or VB.NET application.
using GemBox.Email;
using System;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Incorrectly formatted mail address.
string address = " <invalid.address@gemboxsoftware.com";
MailAddressValidationResult result = MailAddressValidator.Validate(address);
Console.WriteLine($"Address: {address,-40} | Result: {result.Status}");
// Non-existing mail address account.
address = "no-address@gemboxsoftware.com";
result = MailAddressValidator.Validate(address);
Console.WriteLine($"Address: {address,-40} | Result: {result.Status}");
// Non-existing mail address domain.
address = "no-domain@gemboxsoftware123.com";
result = MailAddressValidator.Validate(address);
Console.WriteLine($"Address: {address,-40} | Result: {result.Status}");
// Valid mail address.
address = "Info <info@gemboxsoftware.com>";
result = MailAddressValidator.Validate(address);
Console.WriteLine($"Address: {address,-40} | Result: {result.Status}");
}
}
Imports GemBox.Email
Imports System
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Incorrectly formatted mail address.
Dim address As String = " <invalid.address@gemboxsoftware.com"
Dim result As MailAddressValidationResult = MailAddressValidator.Validate(address)
Console.WriteLine($"Address: {address,-40} | Result: {result.Status}")
' Non-existing mail address account.
address = "no-address@gemboxsoftware.com"
result = MailAddressValidator.Validate(address)
Console.WriteLine($"Address: {address,-40} | Result: {result.Status}")
' Non-existing mail address domain.
address = "no-domain@gemboxsoftware123.com"
result = MailAddressValidator.Validate(address)
Console.WriteLine($"Address: {address,-40} | Result: {result.Status}")
' Valid mail address.
address = "Info <info@gemboxsoftware.com>"
result = MailAddressValidator.Validate(address)
Console.WriteLine($"Address: {address,-40} | Result: {result.Status}")
End Sub
End Module
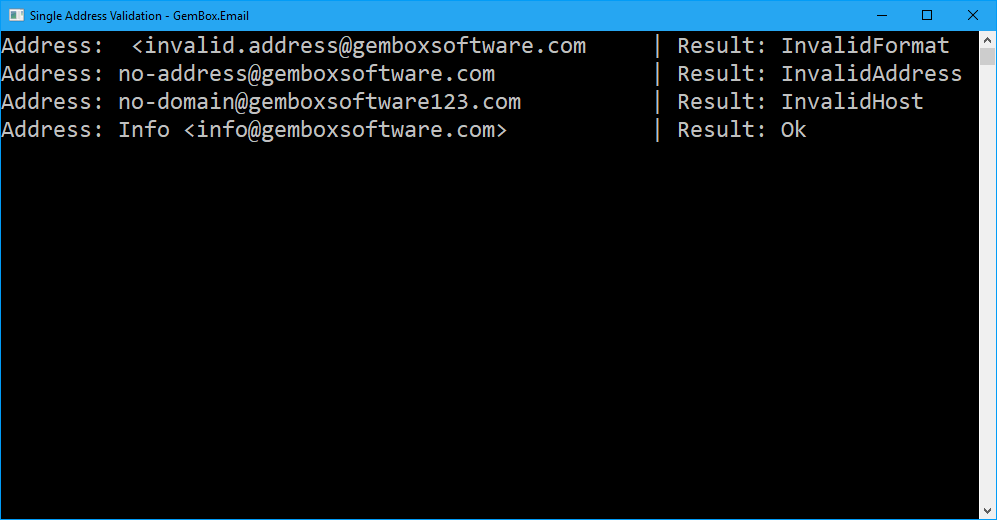
Email address validation has the following features:
- Email syntax verification – Checks the format of an email address to see if it conforms to IETF/RFC standards.
- Domain name validation – The DNS protocol checks the email's domain to see if it exists, and an MX record for the given domain is retrieved.
- Email server validation – Uses the SMTP protocol to check the email server's (MX host) connection and availability.
- Mailbox verification – Checks the existence of a specified email address with the email server's report.
Note, the email address validation is not 100% accurate and may result in occasional false-positive or false-negative results. The reason is that the validation depends on multiple factors, some of which are not under GemBox.Email's control, such as firewalls, ISP settings, DNS settings, email server settings, etc. The example below shows how you can verify a collection of email addresses. To validate a collection of email addresses, you can use Upon validation, the method returns a read-only collection of The example below shows how you can customize email validation by specifying which aspects of an email address to validate. You can use Please note that the validation options always include the preceding ones. For example, Validate multiple email addresses
using GemBox.Email;
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Create a list of mail addresses.
List<MailAddress> addresses = new List<MailAddress>()
{
new MailAddress("no-address@gemboxsoftware.com"),
new MailAddress("no-domain@gemboxsoftware123.com"),
new MailAddress("info@gemboxsoftware.com")
};
// Validate address list and display results.
IList<MailAddressValidationResult> results = MailAddressValidator.Validate(addresses);
Console.WriteLine($"| {"MAIL ADDRESS",-35} | {"RESULT",15} |");
for (int i = 0; i < results.Count; i++)
Console.WriteLine($"| {addresses[i],-35} | {results[i].Status,15} |");
}
}
Imports GemBox.Email
Imports System
Imports System.Collections.Generic
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Create a list of mail addresses.
Dim addresses As New List(Of MailAddress)() From
{
New MailAddress("no-address@gemboxsoftware.com"),
New MailAddress("no-domain@gemboxsoftware123.com"),
New MailAddress("info@gemboxsoftware.com")
}
' Validate address list and display results.
Dim results As IList(Of MailAddressValidationResult) = MailAddressValidator.Validate(addresses)
Console.WriteLine($"| {"MAIL ADDRESS",-35} | {"RESULT",15} |")
For i = 0 To results.Count - 1
Console.WriteLine($"| {addresses(i),-35} | {results(i).Status,15} |")
Next
End Sub
End Module
MailAddressValidator.Validate
overload methods, which accept a collection parameter. These validation methods offer increased efficiency compared to individually validating multiple addresses in a loop. This efficiency is primarily achieved by grouping addresses with the same domain and validating them in a single pass. As a result, the process is accelerated, resource usage is minimized, and the risk of IP blacklisting is reduced.MailAddressValidationResult
values, representing the validation outcomes. Note these results maintain the same order as the provided collection of email addresses.Customize email address validation
using GemBox.Email;
using System;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
Console.WriteLine($"| {"OPTION",-10} | {"MAIL ADDRESS",-35} | {"RESULT",15} |");
// Creating email variables for validation
string invalidSyntaxMail = "invalid.address_gemboxsoftware.com";
string invalidDomainMail = "no-domain@gemboxsoftware123.com";
string invalidAddressMail = "no-address@gemboxsoftware.com";
string validMail = "info@gemboxsoftware.com";
// Incorrectly formatted mail address will fail syntax only validation.
var option = MailAddressValidationOptions.Syntax;
var result = MailAddressValidator.Validate(invalidSyntaxMail, option);
Console.WriteLine($"| {option,-10} | {invalidSyntaxMail,-35} | {result.Status,15} |");
// Non-existing mail address domain will succeed syntax only validation.
option = MailAddressValidationOptions.Syntax;
result = MailAddressValidator.Validate(invalidDomainMail, option);
Console.WriteLine($"| {option,-10} | {invalidDomainMail,-35} | {result.Status,15} |");
// Non-existing mail address domain will fail domain validation.
option = MailAddressValidationOptions.Domain;
result = MailAddressValidator.Validate(invalidDomainMail, option);
Console.WriteLine($"| {option,-10} | {invalidDomainMail,-35} | {result.Status,15} |");
// Non-existing mail address account in a valid domain will succeed domain validation.
option = MailAddressValidationOptions.Domain;
result = MailAddressValidator.Validate(invalidAddressMail, option);
Console.WriteLine($"| {option,-10} | {invalidAddressMail,-35} | {result.Status,15} |");
// Non-existing mail address account in a valid domain will also succeed server validation, because the mail server is reachable
option = MailAddressValidationOptions.Server;
result = MailAddressValidator.Validate(invalidAddressMail, option);
Console.WriteLine($"| {option,-10} | {invalidAddressMail,-35} | {result.Status,15} |");
// Non-existing mail address account in a valid domain will fail mailbox validation
option = MailAddressValidationOptions.Mailbox;
result = MailAddressValidator.Validate(invalidAddressMail, option);
Console.WriteLine($"| {option,-10} | {invalidAddressMail,-35} | {result.Status,15} |");
// Valid mail address will succeed all validation steps.
option = MailAddressValidationOptions.Mailbox;
result = MailAddressValidator.Validate(validMail, option);
Console.WriteLine($"| {option,-10} | {validMail,-35} | {result.Status,15} |");
}
}
Imports GemBox.Email
Imports System
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Console.WriteLine($"| {"OPTION",-10} | {"MAIL ADDRESS",-35} | {"RESULT",15} |")
' Creating email variables for validation
Dim invalidSyntaxMail = "invalid.address_gemboxsoftware.com"
Dim invalidDomainMail = "no-domain@gemboxsoftware123.com"
Dim invalidAddressMail = "no-address@gemboxsoftware.com"
Dim validMail = "info@gemboxsoftware.com"
' Incorrectly formatted mail address will fail syntax only validation.
Dim [option] = MailAddressValidationOptions.Syntax
Dim result = MailAddressValidator.Validate(invalidSyntaxMail, [option])
Console.WriteLine($"| {[option],-10} | {invalidSyntaxMail,-35} | {result.Status,15} |")
' Non-existing mail address domain will succeed syntax only validation.
[option] = MailAddressValidationOptions.Syntax
result = MailAddressValidator.Validate(invalidDomainMail, [option])
Console.WriteLine($"| {[option],-10} | {invalidDomainMail,-35} | {result.Status,15} |")
' Non-existing mail address domain will fail domain validation.
[option] = MailAddressValidationOptions.Domain
result = MailAddressValidator.Validate(invalidDomainMail, [option])
Console.WriteLine($"| {[option],-10} | {invalidDomainMail,-35} | {result.Status,15} |")
' Non-existing mail address account in a valid domain will succeed domain validation.
[option] = MailAddressValidationOptions.Domain
result = MailAddressValidator.Validate(invalidAddressMail, [option])
Console.WriteLine($"| {[option],-10} | {invalidAddressMail,-35} | {result.Status,15} |")
' Non-existing mail address account in a valid domain will also succeed server validation, because the mail server is reachable
[option] = MailAddressValidationOptions.Server
result = MailAddressValidator.Validate(invalidAddressMail, [option])
Console.WriteLine($"| {[option],-10} | {invalidAddressMail,-35} | {result.Status,15} |")
' Non-existing mail address account in a valid domain will fail mailbox validation
[option] = MailAddressValidationOptions.Mailbox
result = MailAddressValidator.Validate(invalidAddressMail, [option])
Console.WriteLine($"| {[option],-10} | {invalidAddressMail,-35} | {result.Status,15} |")
' Valid mail address will succeed all validation steps.
[option] = MailAddressValidationOptions.Mailbox
result = MailAddressValidator.Validate(validMail, [option])
Console.WriteLine($"| {[option],-10} | {validMail,-35} | {result.Status,15} |")
End Sub
End Module
MailAddressValidationOptions
to specify which validation checks should be executed. For example, you can use the Domain
option to check only syntax and if the DNS host is being resolved. Another use case is to check only Syntax
when running on an environment without an internet connection.Domain
option includes Syntax
validation, Server
includes Domain
and Mailbox
includes all validation options.