SMTP Client in C# and VB.NET
The following example shows how to use the GemBox.Email component to connect to, authenticate, and disconnect from a remote SMTP server in your C# and VB.NET application.
using GemBox.Email;
using GemBox.Email.Smtp;
using System;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Create new SMTP client.
using (var smtp = new SmtpClient("<ADDRESS> (e.g. smtp.gmail.com)"))
{
// By default the connect timeout is 5 sec.
smtp.ConnectTimeout = TimeSpan.FromSeconds(4);
// Connect to SMTP server.
smtp.Connect();
Console.WriteLine("Connected.");
// Authenticate using the credentials; username and password.
smtp.Authenticate("<USERNAME>", "<PASSWORD>");
Console.WriteLine("Authenticated.");
}
Console.WriteLine("Disconnected.");
}
}
Imports GemBox.Email
Imports GemBox.Email.Smtp
Imports System
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Create new SMTP client.
Using smtp As New SmtpClient("<ADDRESS> (e.g. smtp.gmail.com)")
' By default the connect timeout is 5 sec.
smtp.ConnectTimeout = TimeSpan.FromSeconds(4)
' Connect to SMTP server.
smtp.Connect()
Console.WriteLine("Connected.")
' Authenticate using the credentials; username and password.
smtp.Authenticate("<USERNAME>", "<PASSWORD>")
Console.WriteLine("Authenticated.")
End Using
Console.WriteLine("Disconnected.")
End Sub
End Module
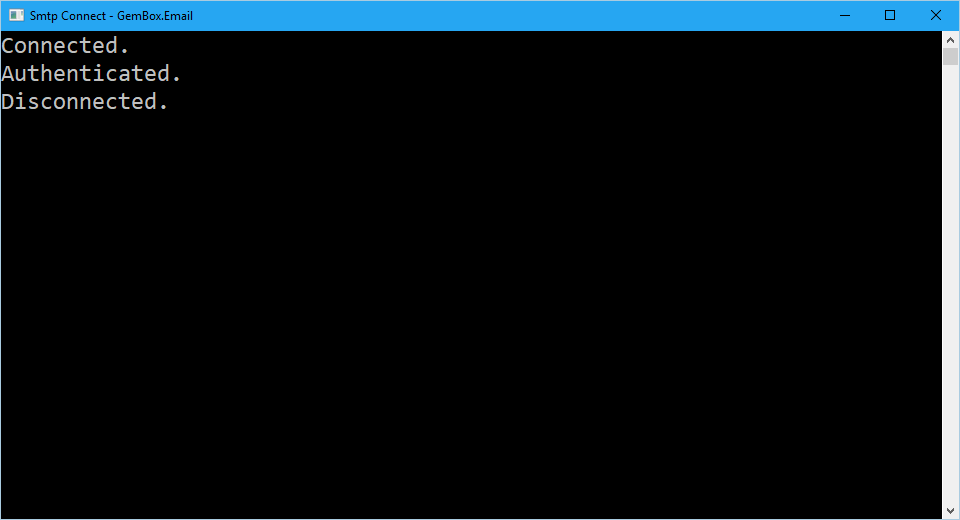
The Simple Mail Transfer Protocol (SMTP) is the only standard protocol for sending mail messages over the Internet. Even though it was defined in RFC 5321 more than 30 years ago, it is still used today, mainly due to its simplicity.
You can reuse the same connection that's established by the SmtpClient
object and your email server multiple times.
When calling the SmtpClient.Authenticate
method, the strongest possible password-based authentication mechanism will be used from the SmtpClient.SupportedAuthentications
collection.
To disconnect from the email server, you can use either the SmtpClient.Disconnect
or SmtpClient.Dispose
method.
Last, SmtpClient
utilizes the following protocol's extensions:
Visit our Send example to learn how to send an email and how to connect with some common SMTP servers (like Gmail, Outlook, Yahoo, etc.).