IMAP Client connection
The following example shows how you can use the GemBox.Email library to connect, authenticate, and disconnect from an IMAP email server.
using GemBox.Email;
using GemBox.Email.Imap;
using System;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Create new IMAP client.
using (var imap = new ImapClient("<ADDRESS> (e.g. imap.gmail.com)"))
{
// By default the connect timeout is 5 sec.
imap.ConnectTimeout = TimeSpan.FromSeconds(4);
// Connect to IMAP server.
imap.Connect();
Console.WriteLine("Connected.");
// Authenticate using the credentials; username and password.
imap.Authenticate("<USERNAME>", "<PASSWORD>");
Console.WriteLine("Authenticated.");
}
Console.WriteLine("Disconnected.");
}
}
Imports GemBox.Email
Imports GemBox.Email.Imap
Imports System
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Create new IMAP client.
Using imap As New ImapClient("<ADDRESS> (e.g. imap.gmail.com)")
' By default the connect timeout is 5 sec.
imap.ConnectTimeout = TimeSpan.FromSeconds(4)
' Connect to IMAP server.
imap.Connect()
Console.WriteLine("Connected.")
' Authenticate using the credentials; username and password.
imap.Authenticate("<USERNAME>", "<PASSWORD>")
Console.WriteLine("Authenticated.")
End Using
Console.WriteLine("Disconnected.")
End Sub
End Module
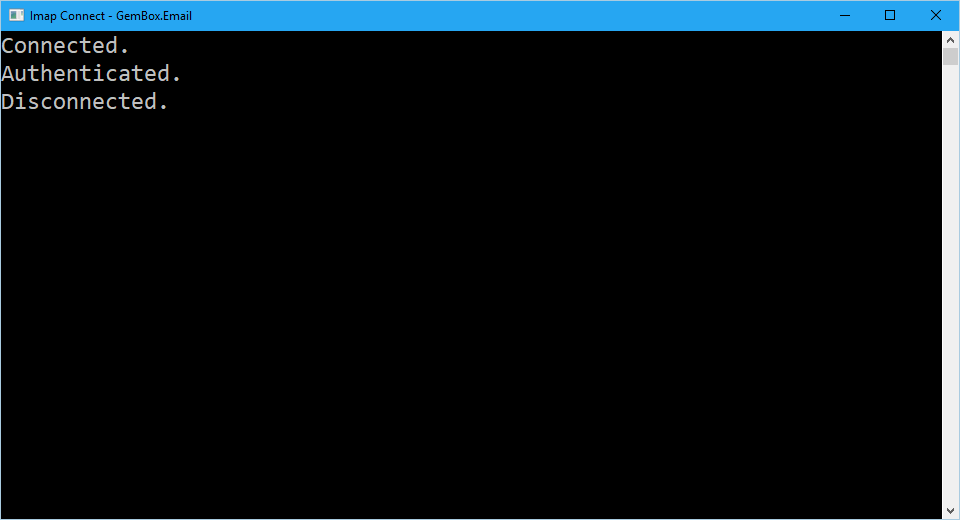
Internet Message Access Protocol (IMAP) is currently the most used protocol for managing an email box by multiple clients or devices. It has replaced the older POP protocol due to its advanced features, which include, but are not limited to, folders, flags and search support, and asynchronous updates by IDLE extension.
GemBox.Email enables you to work with the IMAP protocol in C# and VB.NET using an ImapClient
class. It supports the latest version (version 4; IMAP4) defined in RFC 3501. To learn about how to receive an email and connect with some common IMAP4 servers (like Gmail, Outlook, Yahoo, etc.), visit our Receiving example.
You can reuse the same connection established by the ImapClient
object and your email server multiple times.
When calling the ImapClient.Authenticate
method, the strongest possible password-based authentication mechanism will be used from the ImapClient.SupportedAuthentications
collection.
To disconnect from the email server, you can use either the ImapClient.Disconnect
or ImapClient.Dispose
method.
Last, ImapClient
utilizes the following protocol's extensions: