Create Text Boxes in Word Documents
The following example shows how you can create text boxes with different properties (such as Fill
, Outline
, etc.) using the GemBox.Document components in your C# and VB.NET applications.
using GemBox.Document;
using GemBox.Document.Drawing;
using System.Linq;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = DocumentModel.Load("%InputFileName%");
var paragraph = document.GetChildElements(true).OfType<Paragraph>().First();
// Create and add an inline textbox.
TextBox textbox1 = new TextBox(document,
Layout.Inline(new Size(10, 5, LengthUnit.Centimeter)),
ShapeType.Diamond);
textbox1.Blocks.Add(new Paragraph(document, "An inline TextBox created with GemBox.Document."));
textbox1.Blocks.Add(new Paragraph(document, "It has blue fill and outline."));
textbox1.Fill.SetSolid(new Color(222, 235, 247));
textbox1.Outline.Fill.SetSolid(Color.Blue);
paragraph.Inlines.Insert(0, textbox1);
// Create and add a floating textbox.
TextBox textBox2 = new TextBox(document,
Layout.Floating(
new HorizontalPosition(HorizontalPositionType.Right, HorizontalPositionAnchor.Margin),
new VerticalPosition(VerticalPositionType.Bottom, VerticalPositionAnchor.Margin),
new Size(6, 3, LengthUnit.Centimeter)),
new Paragraph(document, "A floating TextBox created with GemBox.Document."),
new Paragraph(document, "It's rotated and has a default fill and outline."));
textBox2.Layout.Transform.Rotation = 30;
paragraph.Inlines.Add(textBox2);
document.Save("Text Boxes.%OutputFileType%");
}
}
Imports GemBox.Document
Imports GemBox.Document.Drawing
Imports System.Linq
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document As DocumentModel = DocumentModel.Load("%InputFileName%")
Dim paragraph = document.GetChildElements(True).OfType(Of Paragraph)().First()
' Create and add an inline textbox.
Dim textbox1 As New TextBox(document,
Layout.Inline(New Size(10, 5, LengthUnit.Centimeter)),
ShapeType.Diamond)
textbox1.Blocks.Add(New Paragraph(document, "An inline TextBox created with GemBox.Document."))
textbox1.Blocks.Add(New Paragraph(document, "It has blue fill and outline."))
textbox1.Fill.SetSolid(New Color(222, 235, 247))
textbox1.Outline.Fill.SetSolid(Color.Blue)
paragraph.Inlines.Insert(0, textbox1)
' Create and add a floating textbox.
Dim textBox2 As New TextBox(document,
Layout.Floating(
New HorizontalPosition(HorizontalPositionType.Right, HorizontalPositionAnchor.Margin),
New VerticalPosition(VerticalPositionType.Bottom, VerticalPositionAnchor.Margin),
New Size(6, 3, LengthUnit.Centimeter)),
New Paragraph(document, "A floating TextBox created with GemBox.Document."),
New Paragraph(document, "It's rotated and has a default fill and outline."))
textBox2.Layout.Transform.Rotation = 30
paragraph.Inlines.Add(textBox2)
document.Save("Text Boxes.%OutputFileType%")
End Sub
End Module
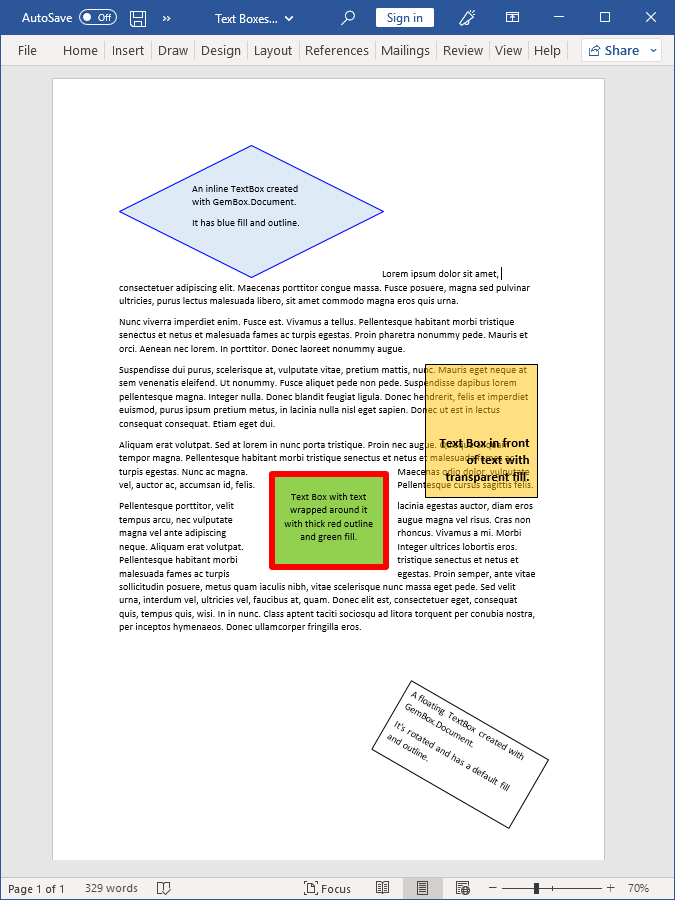
Textboxes can be inline (of InlineLayout
type), in which case the position of the textbox is constrained to the lines of text on the page. Alternatively, they can be floating (of FloatingLayout
type), in which case they can be positioned anywhere on the page.
You can read more about shapes and positioning on the Shapes and Layout help page.