Word Styles
Styles are one of the most essential parts of Word documents: in fact, the best way to create a consistent, well-formatted, professional-looking document is by using Word styles.
Styles define a set of formatting options that you can apply to any number of elements within a document. By accessing and modifying an existing style, all elements that reference that style will automatically change.
With the GemBox.Document API you can create and apply Word styles in your documents programmatically using C# and VB.NET.
The following example shows how to create built-in and custom Word styles and assign them to Run
and Paragraph
elements.
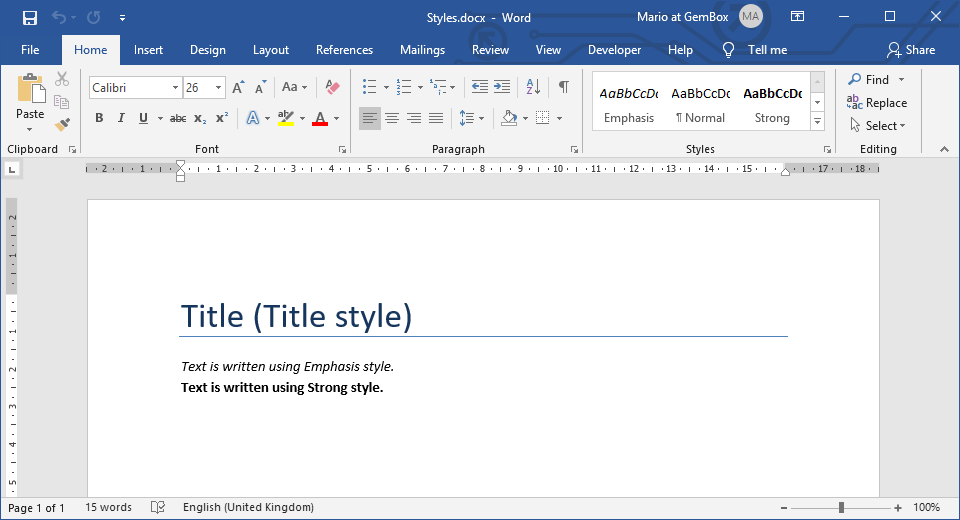
using GemBox.Document;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
DocumentModel document = new DocumentModel();
// Built-in styles can be created using Style.CreateStyle method.
var titleStyle = (ParagraphStyle)Style.CreateStyle(StyleTemplateType.Title, document);
// We can also create our own custom styles.
var emphasisStyle = new CharacterStyle("Emphasis");
emphasisStyle.CharacterFormat.Italic = true;
// To use styles, we first must add them to the document.
document.Styles.Add(titleStyle);
document.Styles.Add(emphasisStyle);
// Or we can use a utility method to get a built-in style or create and add a new one in a single statement.
var strongStyle = (CharacterStyle)document.Styles.GetOrAdd(StyleTemplateType.Strong);
document.Sections.Add(
new Section(document,
new Paragraph(document, "Title (Title style)") { ParagraphFormat = { Style = titleStyle } },
new Paragraph(document,
new Run(document, "Text is written using Emphasis style.") { CharacterFormat = { Style = emphasisStyle } },
new SpecialCharacter(document, SpecialCharacterType.LineBreak),
new Run(document, "Text is written using Strong style.") { CharacterFormat = { Style = strongStyle } })));
document.Save("Styles.%OutputFileType%");
}
}
Imports GemBox.Document
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document As New DocumentModel()
' Built-in styles can be created using Style.CreateStyle method.
Dim titleStyle = DirectCast(Style.CreateStyle(StyleTemplateType.Title, document), ParagraphStyle)
' We can also create our own custom styles.
Dim emphasisStyle As New CharacterStyle("Emphasis")
emphasisStyle.CharacterFormat.Italic = True
' To use styles, we first must add them to the document.
document.Styles.Add(titleStyle)
document.Styles.Add(emphasisStyle)
' Or we can use a utility method to get a built-in style or create and add a new one in a single statement.
Dim strongStyle = DirectCast(document.Styles.GetOrAdd(StyleTemplateType.Strong), CharacterStyle)
document.Sections.Add(
New Section(document,
New Paragraph(document, "Title (Title style)") With {.ParagraphFormat = New ParagraphFormat() With {.Style = titleStyle}},
New Paragraph(document,
New Run(document, "Text is written using Emphasis style.") With {.CharacterFormat = New CharacterFormat() With {.Style = emphasisStyle}},
New SpecialCharacter(document, SpecialCharacterType.LineBreak),
New Run(document, "Text is written using Strong style.") With {.CharacterFormat = New CharacterFormat() With {.Style = strongStyle}})))
document.Save("Styles.%OutputFileType%")
End Sub
End Module
You can create a new style or modify any existing style to define your required formatting and apply that style repeatedly throughout the Word document. This way, you will get efficient document formatting and reduced file size compared to the same document with direct formatting.
If you plan to use a template document, you should consider customizing or creating new styles in it and reusing them with GemBox.Document so that fewer lines of code are needed to format your content.
There are 4 types of styles:
On our Formattings and Styles help page, you can learn more about formatting properties of each style type.
By using styles, you can easily identify different parts of a document and extract those parts for further processing. For instance, you could retrieve only the titles, references, or footnotes from your document by searching for elements that use some targeted style.