Word File Properties
Document properties contain some additional information and details about a file. It's also known as a Word file's metadata and can represent values like author, company, title, and subject. There are two types of document properties, BuiltIn
and Custom
.
If you need to read and write document properties in Word documents programmatically, you can use the GemBox.Document API in your C# and VB.NET applications.
The following example shows how you can read and write built-in and custom document properties.
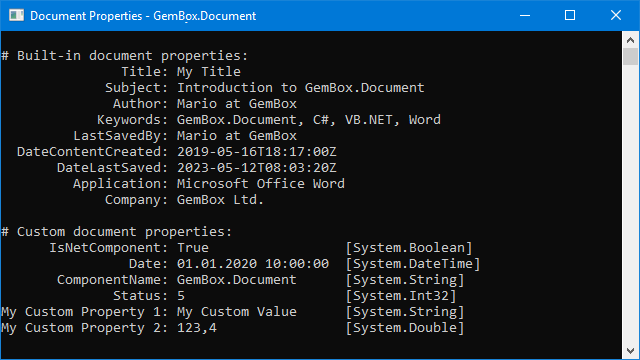
using System;
using GemBox.Document;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = DocumentModel.Load("%InputFileName%");
var documentProperties = document.DocumentProperties;
Console.WriteLine("# Built-in document properties:");
// Write built-in document properties.
documentProperties.BuiltIn[BuiltInDocumentProperty.Title] = "My Title";
documentProperties.BuiltIn[BuiltInDocumentProperty.DateLastSaved] = DateTime.Now.ToString("yyyy-MM-ddTHH:mm:ssZ");
// Read built-in document properties.
foreach (var builtinProperty in documentProperties.BuiltIn)
Console.WriteLine($"{builtinProperty.Key,20}: {builtinProperty.Value}");
Console.WriteLine();
Console.WriteLine("# Custom document properties:");
// Write custom document properties.
documentProperties.Custom["My Custom Property 1"] = "My Custom Value";
documentProperties.Custom["My Custom Property 2"] = 123.4;
// Read custom document properties.
foreach (var customProperty in documentProperties.Custom)
Console.WriteLine($"{customProperty.Key,20}: {customProperty.Value,-20} [{customProperty.Value.GetType()}]");
}
}
Imports System
Imports GemBox.Document
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document As DocumentModel = DocumentModel.Load("%InputFileName%")
Dim documentProperties = document.DocumentProperties
Console.WriteLine("# Built-in document properties:")
' Write built-in document properties.
documentProperties.BuiltIn(BuiltInDocumentProperty.Title) = "My Title"
documentProperties.BuiltIn(BuiltInDocumentProperty.DateLastSaved) = DateTime.Now.ToString("yyyy-MM-ddTHH:mm:ssZ")
' Read built-in document properties.
For Each builtinProperty In documentProperties.BuiltIn
Console.WriteLine($"{builtinProperty.Key,20}: {builtinProperty.Value}")
Next
Console.WriteLine()
Console.WriteLine("# Custom document properties:")
' Write custom document properties.
documentProperties.Custom("My Custom Property 1") = "My Custom Value"
documentProperties.Custom("My Custom Property 2") = 123.4
' Read custom document properties.
For Each customProperty In documentProperties.Custom
Console.WriteLine($"{customProperty.Key,20}: {customProperty.Value,-20} [{customProperty.Value.GetType()}]")
Next
End Sub
End Module
Update Properties and Variables
Using the fields of FieldType.DocProperty
, you can insert the values of the document's properties into the document's content.
Similarly, using the fields of FieldType.DocVariable
, you can insert the values of the document's variables into the document's content. The variables are stored in a DocumentModel.Variables
collection.
The following example shows how you can update the document's properties and variables, and display their updated values with Field
elements.
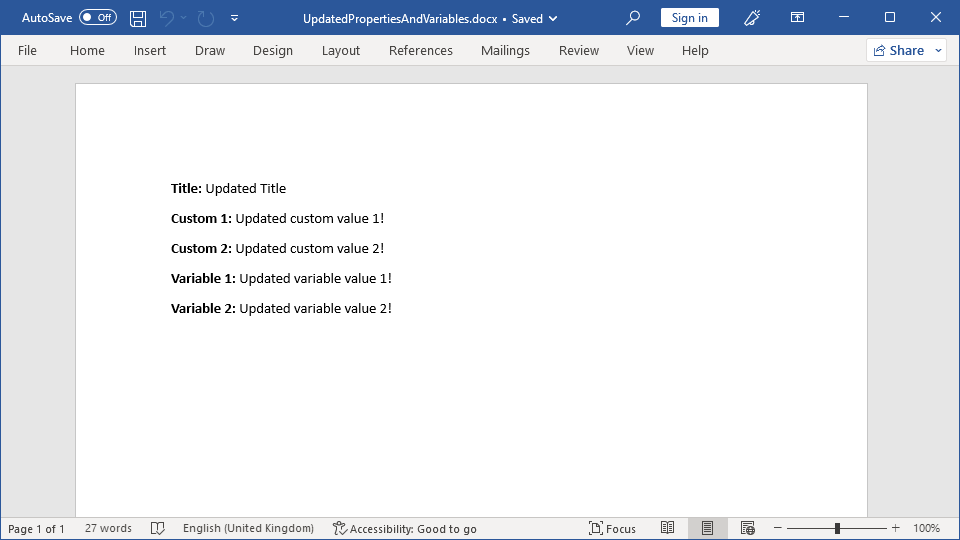
using System.Linq;
using GemBox.Document;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = DocumentModel.Load("%#PropertiesAndVariables.docx%");
// Update built-in and custom document properties.
document.DocumentProperties.BuiltIn[BuiltInDocumentProperty.Title] = "Updated Title";
document.DocumentProperties.Custom["Custom1"] = "Updated custom value 1!";
document.DocumentProperties.Custom["Custom2"] = "Updated custom value 2!";
// Update document variables.
document.Variables["Variable1"] = "Updated variable value 1!";
document.Variables["Variable2"] = "Updated variable value 2!";
var fields = document.GetChildElements(true, ElementType.Field)
.OfType<Field>()
.Where(f => f.FieldType == FieldType.DocProperty || f.FieldType == FieldType.DocVariable);
// Update DOCPROPERTY and DOCVARIABLE fields.
foreach (var field in fields)
field.Update();
document.Save("UpdatedPropertiesAndVariables.%OutputFileType%");
}
}
Imports System.Linq
Imports GemBox.Document
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document = DocumentModel.Load("%#PropertiesAndVariables.docx%")
' Update built-in and custom document properties.
document.DocumentProperties.BuiltIn(BuiltInDocumentProperty.Title) = "Updated Title"
document.DocumentProperties.Custom("Custom1") = "Updated custom value 1!"
document.DocumentProperties.Custom("Custom2") = "Updated custom value 2!"
' Update document variables.
document.Variables("Variable1") = "Updated variable value 1!"
document.Variables("Variable2") = "Updated variable value 2!"
Dim fields = document.GetChildElements(True, ElementType.Field) _
.OfType(Of Field)() _
.Where(Function(f) f.FieldType = FieldType.DocProperty OrElse f.FieldType = FieldType.DocVariable)
' Update DOCPROPERTY and DOCVARIABLE fields.
For Each field In fields
field.Update()
Next
document.Save("UpdatedPropertiesAndVariables.%OutputFileType%")
End Sub
End Module