Add Pictures to Word Documents
The example below shows how you can use GemBox.Document to create pictures of different formats, sizes, and positions in C# and VB.NET.
using GemBox.Document;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = new DocumentModel();
var section = new Section(document);
document.Sections.Add(section);
var paragraph = new Paragraph(document);
section.Blocks.Add(paragraph);
// Create and add an inline picture with GIF image.
Picture picture1 = new Picture(document, "%#Zahnrad.gif%", 61, 53, LengthUnit.Pixel);
paragraph.Inlines.Add(picture1);
// Create and add a floating picture with PNG image.
Picture picture2 = new Picture(document, "%#Dices.png%");
FloatingLayout layout2 = new FloatingLayout(
new HorizontalPosition(HorizontalPositionType.Left, HorizontalPositionAnchor.Page),
new VerticalPosition(2, LengthUnit.Inch, VerticalPositionAnchor.Page),
picture2.Layout.Size);
layout2.WrappingStyle = TextWrappingStyle.InFrontOfText;
picture2.Layout = layout2;
paragraph.Inlines.Add(picture2);
// Create and add a floating picture with SVG image.
Picture picture3 = new Picture(document, "%#Graphics1.svg%", 400, 200, LengthUnit.Pixel);
FloatingLayout layout3 = new FloatingLayout(
new HorizontalPosition(3.5, LengthUnit.Inch, HorizontalPositionAnchor.Page),
new VerticalPosition(2, LengthUnit.Inch, VerticalPositionAnchor.Page),
picture3.Layout.Size);
layout3.WrappingStyle = TextWrappingStyle.BehindText;
picture3.Layout = layout3;
paragraph.Inlines.Add(picture3);
document.Save("Pictures.%OutputFileType%");
}
}
Imports GemBox.Document
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document As New DocumentModel()
Dim section As New Section(document)
document.Sections.Add(section)
Dim paragraph As New Paragraph(document)
section.Blocks.Add(paragraph)
' Create and add an inline picture with GIF image.
Dim picture1 As New Picture(document, "%#Zahnrad.gif%", 61, 53, LengthUnit.Pixel)
paragraph.Inlines.Add(picture1)
' Create and add a floating picture with PNG image.
Dim picture2 As New Picture(document, "%#Dices.png%")
Dim layout2 As New FloatingLayout(
New HorizontalPosition(HorizontalPositionType.Left, HorizontalPositionAnchor.Page),
New VerticalPosition(2, LengthUnit.Inch, VerticalPositionAnchor.Page),
picture2.Layout.Size)
layout2.WrappingStyle = TextWrappingStyle.InFrontOfText
picture2.Layout = layout2
paragraph.Inlines.Add(picture2)
' Create and add a floating picture with SVG image.
Dim picture3 As New Picture(document, "%#Graphics1.svg%", 400, 200, LengthUnit.Pixel)
Dim layout3 As New FloatingLayout(
New HorizontalPosition(3.5, LengthUnit.Inch, HorizontalPositionAnchor.Page),
New VerticalPosition(2, LengthUnit.Inch, VerticalPositionAnchor.Page),
picture3.Layout.Size)
layout3.WrappingStyle = TextWrappingStyle.BehindText
picture3.Layout = layout3
paragraph.Inlines.Add(picture3)
document.Save("Pictures.%OutputFileType%")
End Sub
End Module
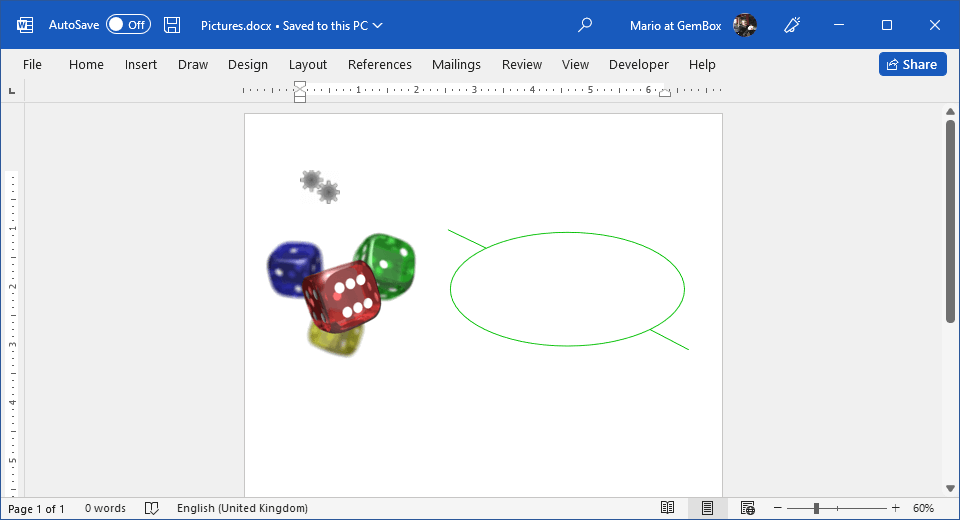
Pictures can be inline (of InlineLayout
type), in which case the position of an image is constrained to the lines of text on the page. Alternatively, they can be floating (of FloatingLayout
type), in which case they can be positioned anywhere on the page.
You can read more about this on the Shapes and Layout help page.
You can replace an image data using the The following example shows how you can add large images and resize them to fit the document's page.Picture.PictureStream
property. Images in SVG format are rendered in PDF files as vector graphics, resulting in smaller file sizes and better quality than bitmap images.Resize images in Word documents
using GemBox.Document;
using System;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = new DocumentModel();
var section = new Section(document);
document.Sections.Add(section);
var picture = new Picture(document, "%#Jellyfish.jpg%");
section.Blocks.Add(new Paragraph(document, picture));
var pictureLayout = picture.Layout;
var pictureSize = pictureLayout.Size;
var pageSetup = section.PageSetup;
var pageSize = new Size(
pageSetup.PageWidth - pageSetup.PageMargins.Left - pageSetup.PageMargins.Right,
pageSetup.PageHeight - pageSetup.PageMargins.Top - pageSetup.PageMargins.Bottom);
double ratioX = pageSize.Width / pictureSize.Width;
double ratioY = pageSize.Height / pictureSize.Height;
double ratio = Math.Min(ratioX, ratioY);
// Resize picture element's size.
if (ratio < 1)
pictureLayout.Size = new Size(pictureSize.Width * ratio, pictureSize.Height * ratio);
document.Save("LargePicture.%OutputFileType%");
}
}
Imports GemBox.Document
Imports System
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document As New DocumentModel()
Dim section As New Section(document)
document.Sections.Add(section)
Dim picture As New Picture(document, "%#Jellyfish.jpg%")
section.Blocks.Add(New Paragraph(document, picture))
Dim pictureLayout = picture.Layout
Dim pictureSize = pictureLayout.Size
Dim pageSetup = section.PageSetup
Dim pageSize = New Size(
pageSetup.PageWidth - pageSetup.PageMargins.Left - pageSetup.PageMargins.Right,
pageSetup.PageHeight - pageSetup.PageMargins.Top - pageSetup.PageMargins.Bottom)
Dim ratioX As Double = pageSize.Width / pictureSize.Width
Dim ratioY As Double = pageSize.Height / pictureSize.Height
Dim ratio As Double = Math.Min(ratioX, ratioY)
' Resize picture element's size.
If ratio < 1 Then
pictureLayout.Size = New Size(pictureSize.Width * ratio, pictureSize.Height * ratio)
End If
document.Save("LargePicture.%OutputFileType%")
End Sub
End Module