Set Paragraph Formatting in Word documents
The example below shows how you can use GemBox.Document for paragraph formatting in Word documents, in C# and VB.NET.
using GemBox.Document;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
DocumentModel document = new DocumentModel();
document.Sections.Add(
new Section(document,
new Paragraph(document, "This paragraph has centered text.")
{
ParagraphFormat =
{
Alignment = HorizontalAlignment.Center
}
},
new Paragraph(document, "This paragraph has the following properties:\nLeft indentation is 10 points.\nRight indentation is 20 points.\nHanging indentation is 30 points.")
{
ParagraphFormat =
{
LeftIndentation = 10,
RightIndentation = 20,
SpecialIndentation = 30
}
},
new Paragraph(document, "This paragraph has the following properties:\nFirst line indentation is 40 points.\nLine spacing is exactly 30 points.\nSpace after and before are 10 points.")
{
ParagraphFormat = new ParagraphFormat
{
SpecialIndentation = -40,
LineSpacing = 30,
LineSpacingRule = LineSpacingRule.Exactly,
SpaceBefore = 10,
SpaceAfter = 10
}
}));
Paragraph paragraph = new Paragraph(document, "This paragraph has borders and background color.");
paragraph.ParagraphFormat.Borders.SetBorders(MultipleBorderTypes.Outside, BorderStyle.Single, new Color(237, 125, 49), 2);
paragraph.ParagraphFormat.BackgroundColor = new Color(251, 228, 213);
document.Sections[0].Blocks.Add(paragraph);
document.Save("Paragraph Formatting.%OutputFileType%");
}
}
Imports GemBox.Document
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document As New DocumentModel()
document.Sections.Add(
New Section(document,
New Paragraph(document, "This paragraph has centered text.") With
{
.ParagraphFormat = New ParagraphFormat() With
{
.Alignment = HorizontalAlignment.Center
}
},
New Paragraph(document, "This paragraph has the following properties:" & vbLf & "Left indentation is 10 points." & vbLf & "Right indentation is 20 points." & vbLf & "Hanging indentation is 30 points.") With
{
.ParagraphFormat = New ParagraphFormat() With
{
.LeftIndentation = 10,
.RightIndentation = 20,
.SpecialIndentation = 30
}
},
New Paragraph(document, "This paragraph has the following properties:" & vbLf & "First line indentation is 40 points." & vbLf & "Line spacing is exactly 30 points." & vbLf & "Space after and before are 10 points.") With
{
.ParagraphFormat = New ParagraphFormat() With
{
.SpecialIndentation = -40,
.LineSpacing = 30,
.LineSpacingRule = LineSpacingRule.Exactly,
.SpaceAfter = 10,
.SpaceBefore = 10
}
}))
Dim paragraph As New Paragraph(document, "This paragraph has borders and background color.")
paragraph.ParagraphFormat.Borders.SetBorders(MultipleBorderTypes.Outside, BorderStyle.Single, New Color(237, 125, 49), 2)
paragraph.ParagraphFormat.BackgroundColor = New Color(251, 228, 213)
document.Sections(0).Blocks.Add(paragraph)
document.Save("Paragraph Formatting.%OutputFileType%")
End Sub
End Module
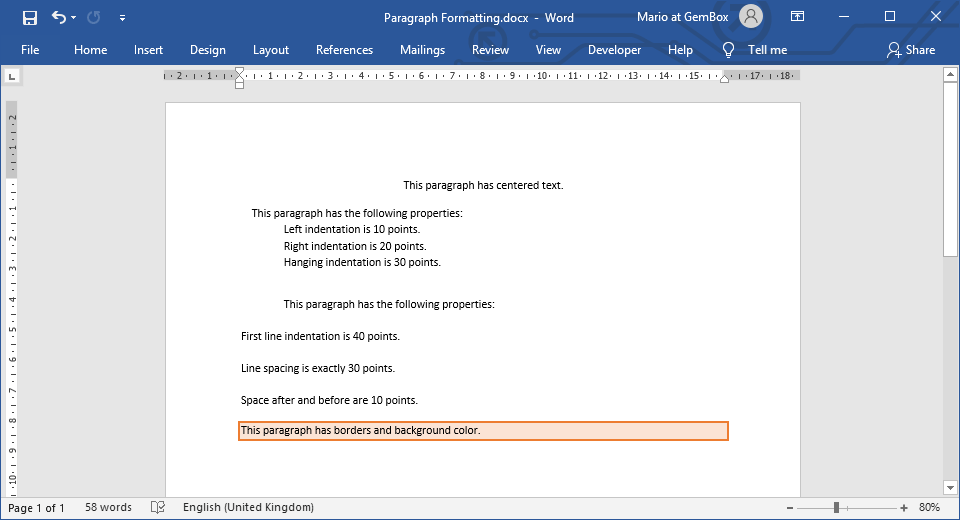
You have various formatting options at your disposal, like alignment, indentation, spacing, borders, tabs, and many more. The formatting can be set directly on the Paragraph
elements by using the Paragraph.ParagraphFormat
property.
Direct formatting has the highest priority in format resolution. If it's not present, then GemBox.Document can retrieve the formatting from a style applied to an element (ParagraphStyle.ParagraphFormat
).
If there is no style, it's possible to retrieve the formatting from the document's default formatting (DocumentModel.DefaultParagraphFormat
).
You can read more about this topic in the Styles example on the Formatting properties resolution help page.